About Data Link
The Data Link dataset by Nasdaq, previously known as Quandl, is a collection of alternative data sets. It has indexed millions of time-series datasets from over 400 sources, which start in different years. This dataset is delivered on several frequencies, but the free data sets have often a daily frequency.
About Nasdaq
"Nasdaq" was initially an acronym for the National Association of Securities Dealers Automated Quotations. It was founded in 1971 by the National Association of Securities Dealers (NASD), now known as the Financial Industry Regulatory Authority (FINRA), with the goal to provide financial services and operate stock exchanges.
On Dec. 4th, 2018, Nasdaq announced it had acquired Quandl, Inc., a leading provider of alternative and core financial data. Quandl was founded by Tammer Kamel in 2012, with goal of making it easy for anyone to find and use high-quality data effectively in their professional decision-making. In 2021, Quandl was replaced by Nasdaq Data Link.
About QuantConnect
QuantConnect was founded in 2012 to serve quants everywhere with the best possible algorithmic trading technology. Seeking to disrupt a notoriously closed-source industry, QuantConnect takes a radically open-source approach to algorithmic trading. Through the QuantConnect web platform, more than 50,000 quants are served every month.
Algorithm Example
from AlgorithmImports import *
from QuantConnect.DataSource import *
class NasdaqDataLinkAlgorithm(QCAlgorithm):
def initialize(self) -> None:
self.set_start_date(1998, 7, 28)
self.set_end_date(1998, 9, 1)
self.set_cash(1000000)
### Requesting Data
# For premium datasets, provide your API Key
# NasdaqDataLink.set_auth_code(self.get_parameter("nasdaq-data-link-api-key"))
self.mrugesh_symbol = self.add_data(NasdaqDataLink, 'BSE/BOM512065', Resolution.DAILY).symbol
# Source: https://data.nasdaq.com/data/BSE/BOM512065-mrugesh-trading-ltd-eod-prices
self.london_gold_symbol = self.add_data(NasdaqCustomColumns, "LBMA/DAILY", Resolution.DAILY).symbol
# This dataset has multiple data columns, so create a custom class to set the value column name
# Source: https://data.nasdaq.com/data/LBMA/DAILY-london-gold-fixings-19911999
### Historical Data
history = self.history(self.mrugesh_symbol, 1000, Resolution.DAILY)
self.debug(f"We got {len(history)} items from our history request for {self.mrugesh_symbol} Nasdaq Data Link")
history = self.history(self.london_gold_symbol, 100, Resolution.DAILY)
self.debug(f"We got {len(history)} items from our history request for {self.london_gold_symbol} Nasdaq Data Link")
def on_data(self, slice: Slice) -> None:
### Accessing Data
if slice.contains_key(self.mrugesh_symbol):
self.set_holdings(self.mrugesh_symbol, -1)
if slice.contains_key(self.london_gold_symbol):
value = slice[self.london_gold_symbol].value
class NasdaqCustomColumns(NasdaqDataLink):
def __init__(self) -> None:
# Select the column "am usd".
self.value_column_name = "am usd"
Example Applications
The Nasdaq Data Link sources allow you to explore different kinds of data in their database to develop trading strategies. Examples include the following strategies:
- Using alternative data to regress market regime/asset price.
- Backtesting exotic derivatives or private Equity investments.
Explore Other Datasets
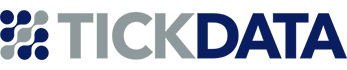
US Cash Indices
Dataset by TickData
US Future Options
Dataset by AlgoSeek
Binance Crypto Future Margin Rate Data
Dataset by QuantConnect