About US SEC Filings
The US SEC Filings dataset provides the quarterly financial earning reports that the United States Securities and Exchange Commission (SEC) requires from publicly traded companies in the US. The data covers 15,000 US Equities, starts in January 1998, and is delivered on a daily frequency. The data is sourced from the SEC's Electronic Data Gathering, Analysis, and Retrieval (EDGAR) system. QuantConnect downloads and formats the Quarterly Financial Reports (10-Q) and Annual Financial Report (8-K) filings of companies into a format for easy consumption by LEAN.
About Securities and Exchange Commission
The mission of the U.S. Securities and Exchange Commission is to protect investors, maintain fair, orderly, and efficient markets, and facilitate capital formation. The SEC oversees the key participants in the securities world, including securities exchanges, securities brokers and dealers, investment advisors, and mutual funds. The SEC is concerned primarily with promoting the disclosure of important market-related information, maintaining fair dealing, and protecting against fraud.
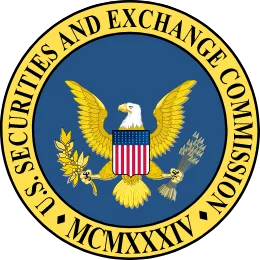
About QuantConnect
QuantConnect was founded in 2012 to serve quants everywhere with the best possible algorithmic trading technology. Seeking to disrupt a notoriously closed-source industry, QuantConnect takes a radically open-source approach to algorithmic trading. Through the QuantConnect web platform, more than 50,000 quants are served every month.
Algorithm Example
from AlgorithmImports import *
from QuantConnect.DataSource import *
class SECReport8KAlgorithm(QCAlgorithm):
def initialize(self) -> None:
self.set_start_date(2019, 1, 1)
self.set_end_date(2019, 12, 31)
self.set_cash(100000)
self.universe_settings.resolution = Resolution.DAILY
self.add_universe(self.coarse_selector)
self.dataset_symbol_by_symbol = {}
self.long_symbols = []
self.rebalance = False
# Request underlying equity data.
ibm = self.add_equity("IBM", Resolution.DAILY).symbol
# Add news data for the underlying IBM asset
earnings_filing = self.add_data(SECReport10Q, ibm, Resolution.DAILY).symbol
# Request 120 days of history with the SECReport10Q IBM custom data Symbol
history = self.history(SECReport10Q, earnings_filing, 120, Resolution.DAILY)
# Count the number of items we get from our history request
self.debug(f"We got {len(history)} items from our history request")
def coarse_selector(self, coarse: List[CoarseFundamental]) -> List[Symbol]:
coarse = sorted([cf for cf in coarse if cf.has_fundamental_data],
key=lambda cf: cf.dollar_volume, reverse=True)[:10]
return [cf.symbol for cf in coarse]
def on_data(self, slice: Slice) -> None:
# Get all SEC data and loop over it
for report in slice.Get(SECReport8K).Values:
underlying_symbol = report.symbol.underlying
# Skip the Symbol if it's no longer in the universe
if underlying_symbol not in self.dataset_symbol_by_symbol:
if underlying_symbol in self.long_symbols:
self.rebalance = True
self.long_symbols.remove(underlying_symbol)
continue
# Get the length of all contents contained within the report
report_text_length = sum([len(i.text) for i in report.report.documents])
if report_text_length > 20000:
if underlying_symbol not in self.long_symbols:
self.rebalance = True
self.long_symbols.append(underlying_symbol)
elif underlying_symbol in self.long_symbols:
self.rebalance = True
self.long_symbols.remove(underlying_symbol)
if not self.rebalance:
return
self.rebalance = False
portfolio_targets = []
equal_weighting = 1 / len(self.long_symbols) if len(self.long_symbols) > 0 else 0
for symbol, security_holding in self.portfolio.items():
weight = 0
if symbol in self.long_symbols:
weight = equal_weighting
elif not security_holding.invested:
continue
portfolio_targets.append(PortfolioTarget(symbol, weight))
self.set_holdings(portfolio_targets)
def on_securities_changed(self, changes: SecurityChanges) -> None:
for security in changes.added_securities:
self.dataset_symbol_by_symbol[security.symbol] = self.add_data(SECReport8K, security.symbol).symbol
for security in changes.removed_securities:
dataset_symbol = self.dataset_symbol_by_symbol.pop(security.symbol, None)
if dataset_symbol:
self.remove_security(dataset_symbol)
Example Applications
The US SEC Filings dataset enables you to create strategies using information from SEC reports. Examples include the following strategies:
- Extracting information about corporate earnings from the documents for further analysis
- Applying sentiment analysis to the text content of the documents (for example, keyword scoring and ranking)
Pricing
Cloud Access
SEC Filling data in the QuantConnect Cloud for your backtesting and live trading purposes.
Download On Premise
SEC Fillings data archived in LEAN format for on premise backtesting and research. One file per ticker.
Explore Other Datasets
US Equity Security Master
Dataset by QuantConnect
Binance Crypto Future Margin Rate Data
Dataset by QuantConnect
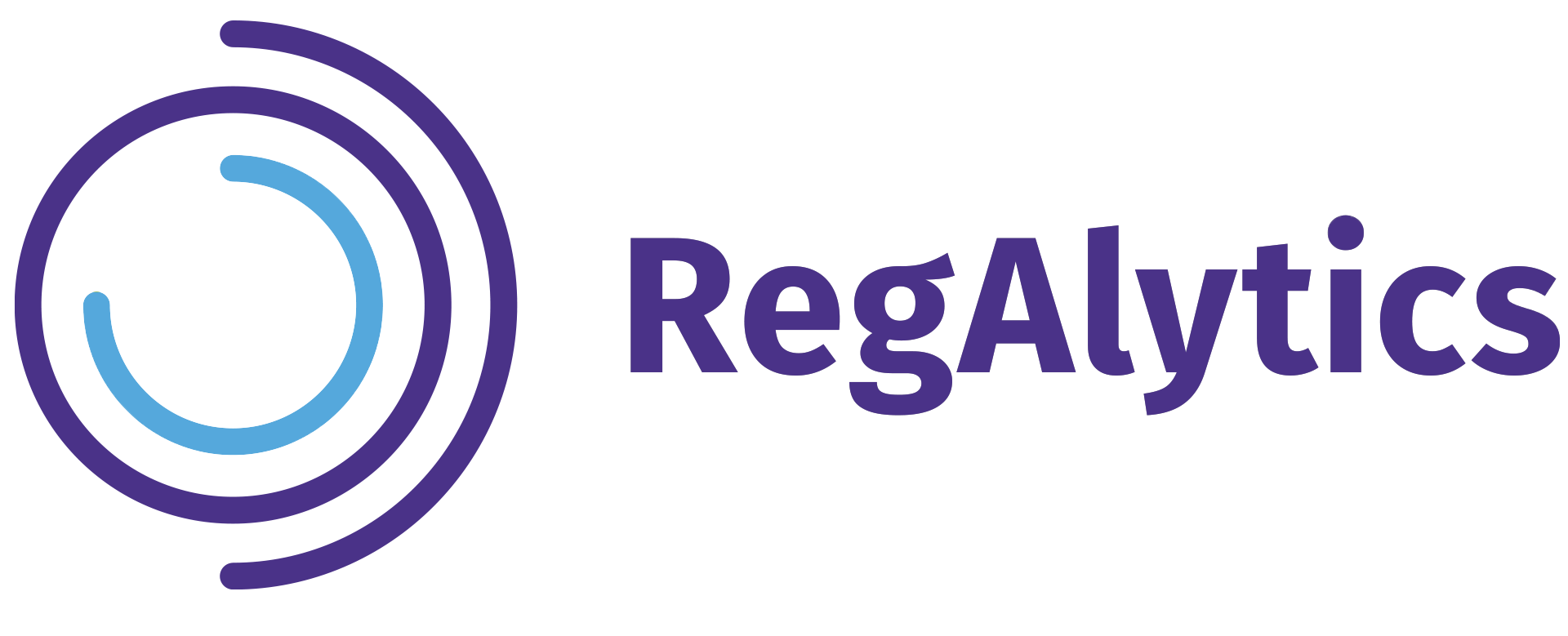
US Regulatory Alerts - Financial Sector
Dataset by RegAlytics