Hi,
I am trying to write a program that takes in one-minute resolution historical data from a CSV file that is stored in DropBox. Here is a link to the DropBox file: I notice that every time I try to run the code, I don't get an error, but I get the message waiting for chart in the backtest screen. I tried waiting for over an hour and still notice that the message is the same. Could someone please help me understand what I am doing wrong in my code?
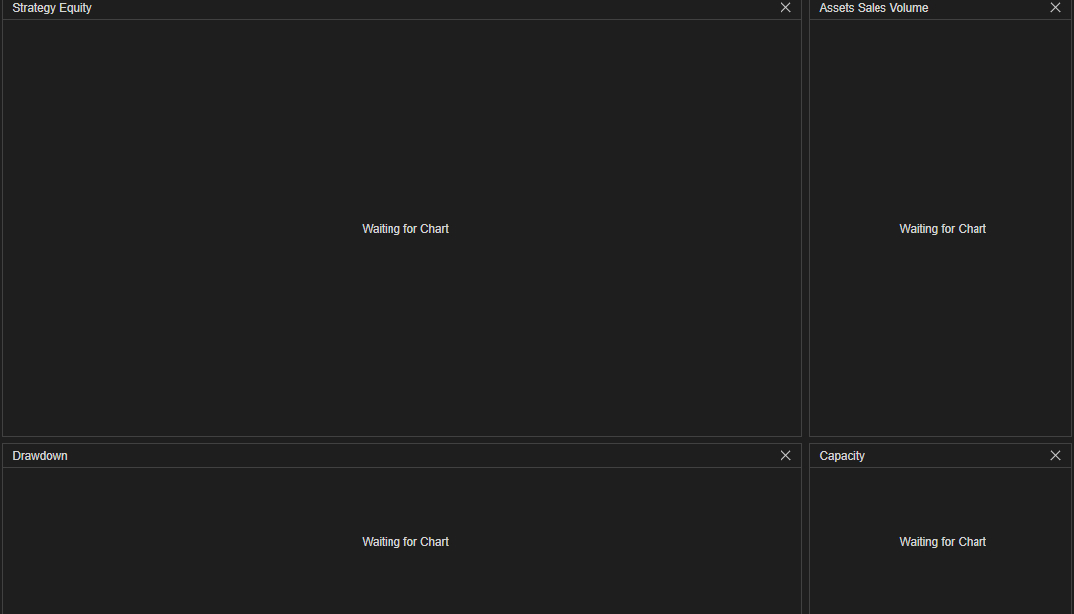
Below is the code that I am running.
# region imports
from AlgorithmImports import *
from datetime import datetime
from QuantConnect import *
from QuantConnect.Algorithm import *
from QuantConnect.Data import SubscriptionDataSource
from QuantConnect.Python import PythonData
import pandas as pd
# endregion
class PensiveMagentaAlligator(QCAlgorithm):
def Initialize(self):
self.SetStartDate(2023, 4, 1)
self.SetEndDate(2023, 5, 3)
self.SetCash(10000000)
self.symbol = self.AddData(MyCustomDataType, "IBM", Resolution.Minute).Symbol
self.SetWarmUp(300, Resolution.Minute)
def OnData(self, data):
if self.IsWarmingUp: return
hstv = self.History(self.symbol, 500, Resolution.Minute)
histClose = pd.DataFrame(hstv)["close"]
histHigh = pd.DataFrame(hstv)["high"]
histLow = pd.DataFrame(hstv)["low"]
self.Log(histClose[-1])
class MyCustomDataType(PythonData):
def GetSource(self, config: SubscriptionDataConfig, date: datetime, isLive: bool) -> SubscriptionDataSource:
return SubscriptionDataSource("https://www.dropbox.com/s/uiwrna3r9fqmxwa/IBM_Intraday.csv?dl=1", SubscriptionTransportMedium.RemoteFile)
def Reader(self, config: SubscriptionDataConfig, line: str, date: datetime, isLive: bool) -> BaseData:
if not (line.strip()):
return None
index = MyCustomDataType()
index.Symbol = "IBM"
try:
data = line.split(',')
index.Time = datetime.strptime(data[0], "%Y-%m-%d %H:%M:%S")
index.Value = float(data[4])
index["open"] = float(data[1])
index["high"] = float(data[2])
index["low"] = float(data[3])
index["close"] = float(data[4])
except ValueError:
return None
return index
Yuri Lopukhov
100Mb is a lot of data, and processing it takes quite a lot of time. I noticed that it processes all of the file each time you call History, so basically on every minute bar, so it takes quite a lot of time. Perhaps a better approach would be to download the file with self.Download() and process it manually. Or you could remove dates you are not interested in from the file and reduce it's size.
Rishab Maheshwari
The material on this website is provided for informational purposes only and does not constitute an offer to sell, a solicitation to buy, or a recommendation or endorsement for any security or strategy, nor does it constitute an offer to provide investment advisory services by QuantConnect. In addition, the material offers no opinion with respect to the suitability of any security or specific investment. QuantConnect makes no guarantees as to the accuracy or completeness of the views expressed in the website. The views are subject to change, and may have become unreliable for various reasons, including changes in market conditions or economic circumstances. All investments involve risk, including loss of principal. You should consult with an investment professional before making any investment decisions.
To unlock posting to the community forums please complete at least 30% of Boot Camp.
You can continue your Boot Camp training progress from the terminal. We hope to see you in the community soon!