Hi,
At the bottom of this post is part of the buy logic of an algo that I am working on. In short, when there is a buy signal, the order should place a stop limit order at 1 cent above the high. The order should be valid for the duration of the entire bar, which in this case is one minute, and if it has not been filled it should be canceled. For the most part, the algo is doing the right thing, but there are several instances I noticed where something is going wrong.
Instance 1:
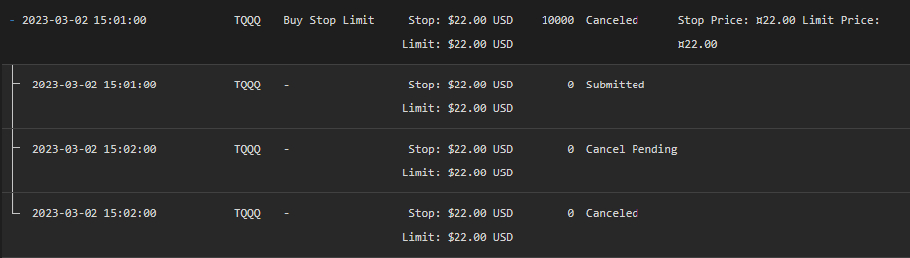
Instance 2:
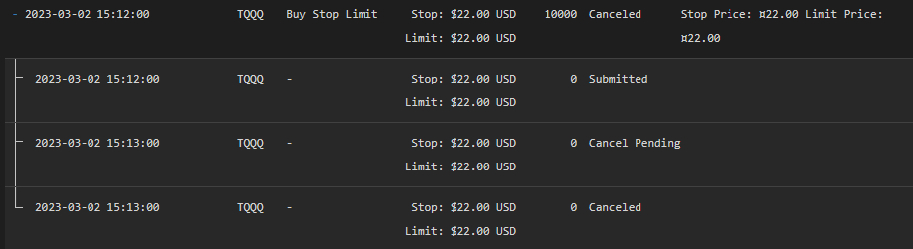
Instance 3:
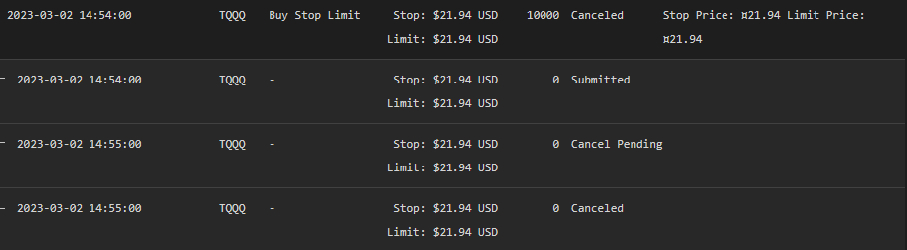
Could someone please help me understand what is going wrong in this program?
# region imports
from AlgorithmImports import *
# endregion
import pandas as pd
import numpy as np
from ta.trend import ema_indicator
from ta.volatility import bollinger_hband, bollinger_lband
import pandas_ta as ta
class LogicalBrownScorpion(QCAlgorithm):
def Initialize(self):
self.SetStartDate(2023, 3, 1)
self.SetEndDate(2023, 3, 24)
self.SetCash(1000000)
self.symbol = self.AddEquity("TQQQ", Resolution.Second, dataNormalizationMode=DataNormalizationMode.SplitAdjusted).Symbol
self.Consolidate(self.symbol, Resolution.Minute, self.CustomHandler)
self.barsSince = 0
self.ticket = None
self.OrderPlaced = False
self.entryPrice = None
self.SetWarmUp(300, Resolution.Minute)
def OnData(self, data: Slice):
try:
if self.IsWarmingUp: return
if self.Portfolio.Invested:
price = data.Bars[self.symbol].Close
if price >= self.entryPrice + .05:
self.Liquidate(self.symbol)
self.OrderPlaced = False
self.barsSince = 0
except:
pass
def CustomHandler(self, bar):
if self.IsWarmingUp: return
if self.OrderPlaced:
self.barsSince += 1
if self.barsSince == 1:
if self.ticket is not None:
self.ticket.Cancel()
self.OrderPlaced = False
self.barsSince = 0
if not self.Portfolio.Invested and self.OrderPlaced == False:
try:
history = self.History([self.symbol], 2, Resolution.Minute)
high_prices = history.high.unstack(level=0)[self.symbol]
current_high = round(float(high_prices[-1]), 2)
low_prices = history.low.unstack(level=0)[self.symbol]
current_low = round(float(low_prices[-1]), 2)
if buySignal1:
self.ticket = self.StopLimitOrder(self.symbol, 10000, current_high + .01, current_high + .01)
self.OrderPlaced = True
elif buySignal2:
self.ticket = self.StopLimitOrder(self.symbol, 10000, current_high + .01, current_high + .01)
self.OrderPlaced = True
except:
pass
Louis Szeto
Hi Rishab
You should place a LimitOrder, not a StopLimitOrder. StopLimitOrder is for a stop loss but not as a trigger.
Best
Louis
The material on this website is provided for informational purposes only and does not constitute an offer to sell, a solicitation to buy, or a recommendation or endorsement for any security or strategy, nor does it constitute an offer to provide investment advisory services by QuantConnect. In addition, the material offers no opinion with respect to the suitability of any security or specific investment. QuantConnect makes no guarantees as to the accuracy or completeness of the views expressed in the website. The views are subject to change, and may have become unreliable for various reasons, including changes in market conditions or economic circumstances. All investments involve risk, including loss of principal. You should consult with an investment professional before making any investment decisions.
Rishab Maheshwari
The material on this website is provided for informational purposes only and does not constitute an offer to sell, a solicitation to buy, or a recommendation or endorsement for any security or strategy, nor does it constitute an offer to provide investment advisory services by QuantConnect. In addition, the material offers no opinion with respect to the suitability of any security or specific investment. QuantConnect makes no guarantees as to the accuracy or completeness of the views expressed in the website. The views are subject to change, and may have become unreliable for various reasons, including changes in market conditions or economic circumstances. All investments involve risk, including loss of principal. You should consult with an investment professional before making any investment decisions.
To unlock posting to the community forums please complete at least 30% of Boot Camp.
You can continue your Boot Camp training progress from the terminal. We hope to see you in the community soon!