Charting
Matplotlib
Introduction
matplotlib
is the most popular 2d-charting library for python. It allows you to easily create histograms, scatter plots, and various other charts. In addition, pandas
is integrated with matplotlib
, so you can seamlessly move between data manipulation and data visualization. This makes matplotlib
great for quickly producing a chart to visualize your data.
Import Libraries
Follow these steps to import the libraries that you need:
- Import the
matplotlib
,mplfinance
, andnumpy
libraries. - Import, and then call, the
register_matplotlib_converters
method.
import matplotlib.pyplot as plt import mplfinance import numpy as np
from pandas.plotting import register_matplotlib_converters register_matplotlib_converters()
Get Historical Data
Get some historical market data to produce the plots. For example, to get data for a bank sector ETF and some banking companies over 2021, run:
qb = QuantBook() tickers = ["XLF", # Financial Select Sector SPDR Fund "COF", # Capital One Financial Corporation "GS", # Goldman Sachs Group, Inc. "JPM", # J P Morgan Chase & Co "WFC"] # Wells Fargo & Company symbols = [qb.add_equity(ticker, Resolution.DAILY).symbol for ticker in tickers] history = qb.history(symbols, datetime(2021, 1, 1), datetime(2022, 1, 1))
Create Candlestick Chart
You must import the plotting libraries and get some historical data to create candlestick charts.
In this example, we'll create a candlestick chart that shows the open, high, low, and close prices of one of the banking securities. Follow these steps to create the candlestick chart:
- Select a
Symbol
. - Slice the
history
DataFrame
with thesymbol
. - Rename the columns.
- Call the
plot
method with thedata
, chart type, style, title, y-axis label, and figure size.
symbol = symbols[0]
data = history.loc[symbol]
data.columns = ['Close', 'High', 'Low', 'Open', 'Volume']
mplfinance.plot(data, type='candle', style='charles', title=f'{symbol.value} OHLC', ylabel='Price ($)', figratio=(15, 10))
The Jupyter Notebook displays the candlestick chart.
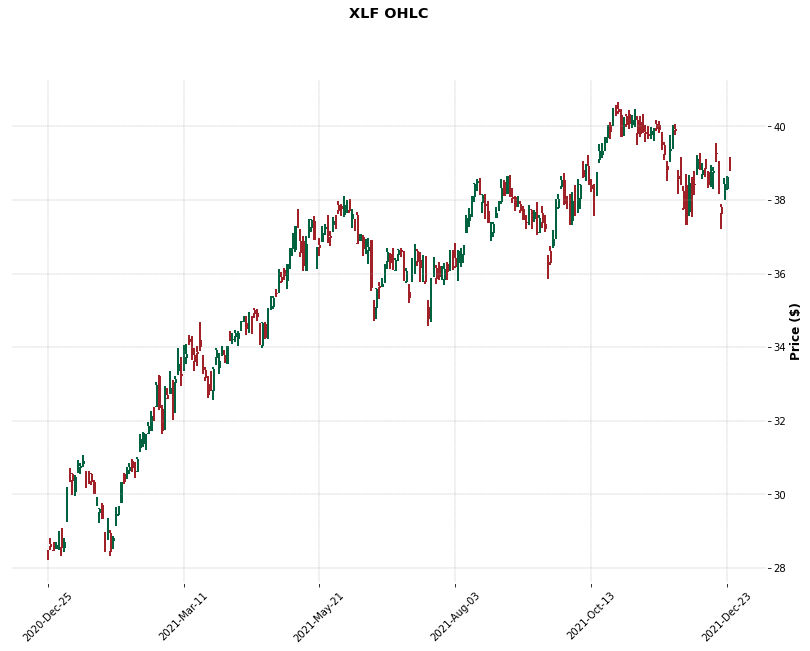
Create Line Plot
You must import the plotting libraries and get some historical data to create line charts.
In this example, you create a line chart that shows the closing price for one of the banking securities. Follow these steps to create the line chart:
- Select a
Symbol
. - Slice the
history
DataFrame withsymbol
and then select the close column. - Call the
plot
method with a title and figure size.
symbol = symbols[0]
data = history.loc[symbol]['close']
data.plot(title=f"{symbol} Close Price", figsize=(15, 10));
The Jupyter Notebook displays the line plot.
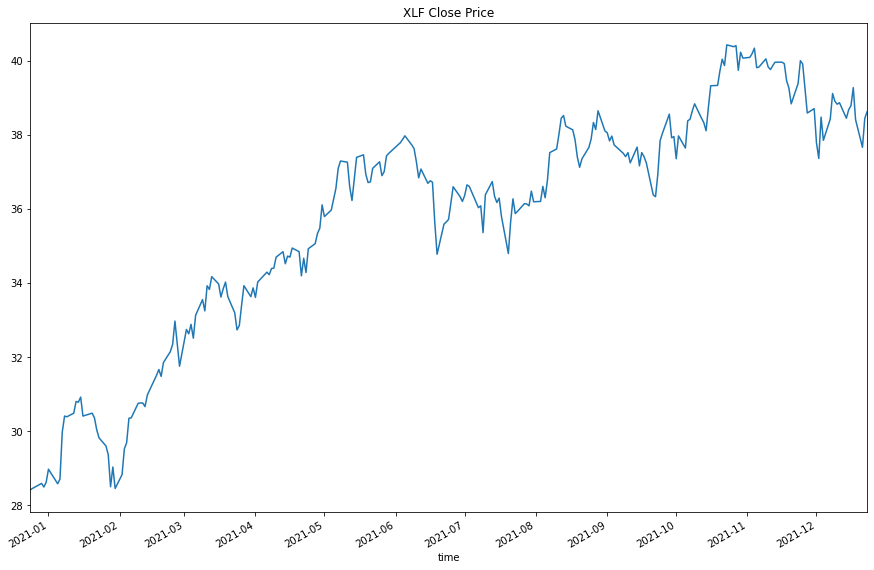
Create Scatter Plot
You must import the plotting libraries and get some historical data to create scatter plots.
In this example, you create a scatter plot that shows the relationship between the daily returns of two banking securities. Follow these steps to create the scatter plot:
- Select the 2
Symbol
s. - Slice the
history
DataFrame with eachSymbol
and then select the close column. - Call the
pct_change
anddropna
methods on eachSeries
. - Call the
polyfit
method with thedaily_returns1
,daily_returns2
, and a degree. - Call the
linspace
method with the minimum and maximum values on the x-axis. - Calculate the y-axis coordinates of the regression line.
- Call the
plot
method with the coordinates and color of the regression line. - In the same cell that you called the
plot
method, call thescatter
method with the 2 daily return series. - In the same cell that you called the
scatter
method, call thetitle
,xlabel
, andylabel
methods with a title and axis labels.
For example, to select the Symbol
s of the first 2 bank stocks, run:
symbol1 = symbols[1] symbol2 = symbols[2]
close_price1 = history.loc[symbol1]['close'] close_price2 = history.loc[symbol2]['close']
daily_returns1 = close_price1.pct_change().dropna() daily_returns2 = close_price2.pct_change().dropna()
m, b = np.polyfit(daily_returns1, daily_returns2, deg=1)
This method call returns the slope and intercept of the ordinary least squares regression line.
x = np.linspace(daily_returns1.min(), daily_returns1.max())
y = m*x + b
plt.plot(x, y, color='red')
plt.scatter(daily_returns1, daily_returns2)
plt.title(f'{symbol1} vs {symbol2} daily returns Scatter Plot') plt.xlabel(symbol1.value) plt.ylabel(symbol2.value);
The Jupyter Notebook displays the scatter plot.
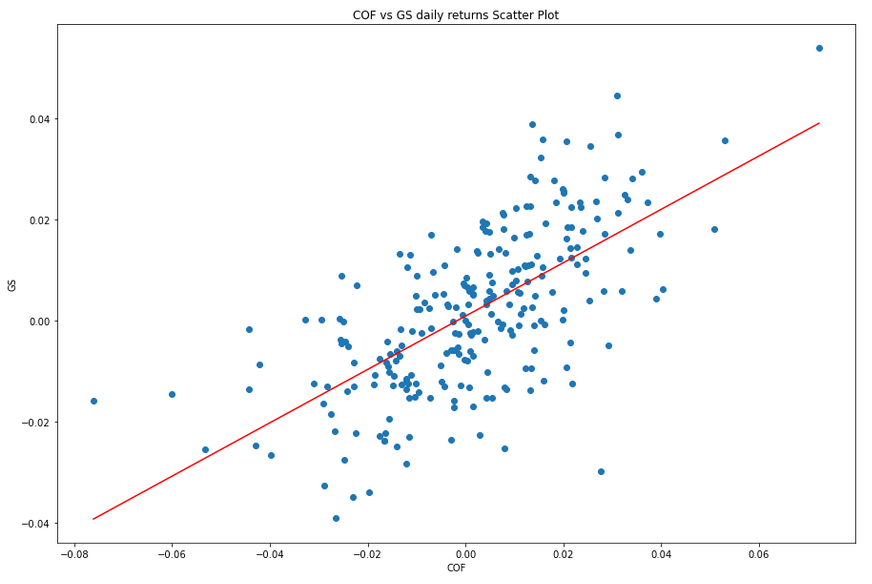
Create Histogram
You must import the plotting libraries and get some historical data to create histograms.
In this example, you create a histogram that shows the distribution of the daily percent returns of the bank sector ETF. In addition to the bins in the histogram, you overlay a normal distribution curve for comparison. Follow these steps to create the histogram:
- Select the
Symbol
. - Slice the
history
DataFrame with thesymbol
and then select the close column. - Call the
pct_change
method and then call thedropna
method. - Call the
mean
andstd
methods. - Call the
linspace
method with the lower limit, upper limit, and number data points for the x-axis of the normal distribution curve. - Calculate the y-axis values of the normal distribution curve.
- Call the
plot
method with the data for the normal distribution curve. - In the same cell that you called the
plot
method, call thehist
method with the daily return data and the number of bins. - In the same cell that you called the
hist
method, call thetitle
,xlabel
, andylabel
methods with a title and the axis labels.
symbol = symbols[0]
close_prices = history.loc[symbol]['close']
daily_returns = close_prices.pct_change().dropna()
mean = daily_returns.mean() std = daily_returns.std()
x = np.linspace(-3*std, 3*std, 1000)
pdf = 1/(std * np.sqrt(2*np.pi)) * np.exp(-(x-mean)**2 / (2*std**2))
plt.plot(x, pdf, label="Normal Distribution")
plt.hist(daily_returns, bins=20)
plt.title(f'{symbol} Return Distribution') plt.xlabel('Daily Return') plt.ylabel('Count');
The Jupyter Notebook displays the histogram.
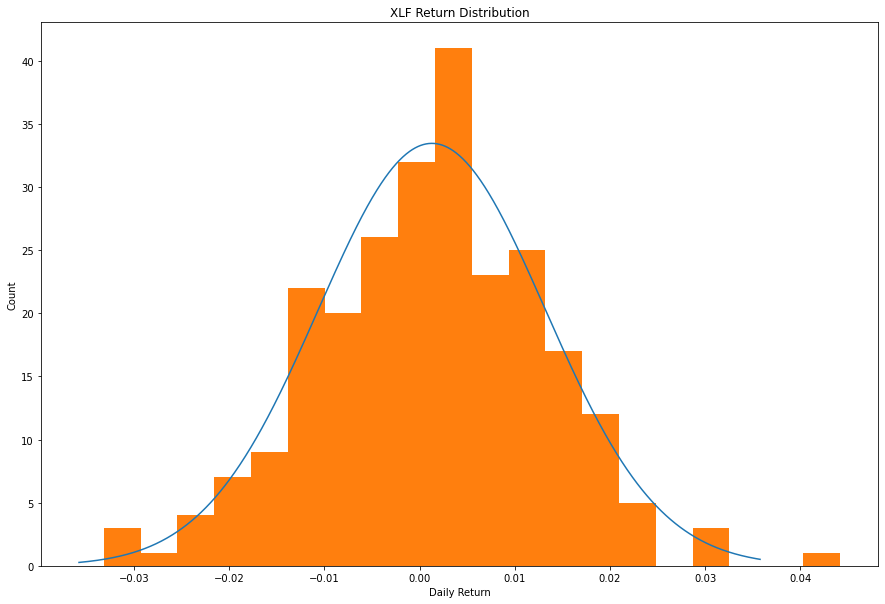
Create Bar Chart
You must import the plotting libraries and get some historical data to create bar charts.
In this example, you create a bar chart that shows the average daily percent return of the banking securities. Follow these steps to create the bar chart:
- Select the close column and then call the
unstack
method. - Call the
pct_change
method and then multiply by 100. - Call the
mean
method. - Call the
figure
method with a figure size. - Call the
bar
method with the x-axis and y-axis values. - In the same cell that you called the
bar
method, call thetitle
,xlabel
, andylabel
methods with a title and the axis labels.
close_prices = history['close'].unstack(level=0)
daily_returns = close_prices.pct_change() * 100
avg_daily_returns = daily_returns.mean()
plt.figure(figsize=(15, 10))
plt.bar(avg_daily_returns.index, avg_daily_returns)
plt.title('Banking Stocks Average Daily % Returns') plt.xlabel('Tickers') plt.ylabel('%');
The Jupyter Notebook displays the bar chart.
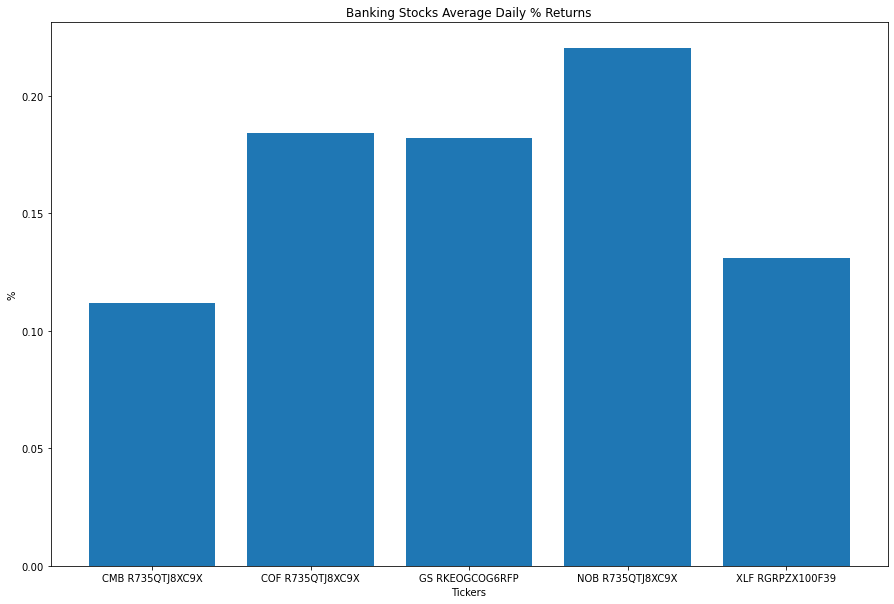
Create Heat Map
You must import the plotting libraries and get some historical data to create heat maps.
In this example, you create a heat map that shows the correlation between the daily returns of the banking securities. Follow these steps to create the heat map:
- Select the close column and then call the
unstack
method. - Call the
pct_change
method. - Call the
corr
method. - Call the
imshow
method with the correlation matrix, a color map, and an interpolation method. - In the same cell that you called the
imshow
method, call thetitle
,xticks
, andyticks
, methods with a title and the axis tick labels. - In the same cell that you called the
imshow
method, call thecolorbar
method.
close_prices = history['close'].unstack(level=0)
daily_returns = close_prices.pct_change()
corr_matrix = daily_returns.corr()
plt.imshow(corr_matrix, cmap='hot', interpolation='nearest')
plt.title('Banking Stocks and Bank Sector ETF Correlation Heat Map') plt.xticks(np.arange(len(tickers)), labels=tickers) plt.yticks(np.arange(len(tickers)), labels=tickers)
plt.colorbar();
The Jupyter Notebook displays the heat map.
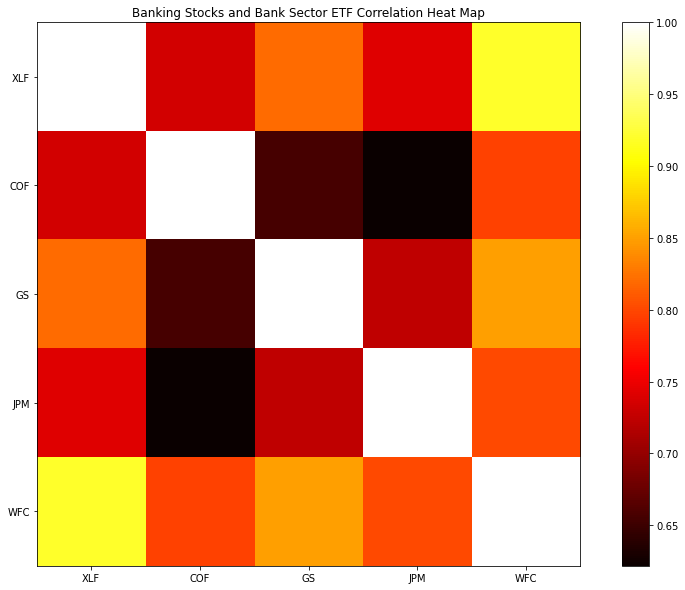
Create Pie Chart
You must import the plotting libraries and get some historical data to create pie charts.
In this example, you create a pie chart that shows the weights of the banking securities in a portfolio if you allocate to them based on their inverse volatility. Follow these steps to create the pie chart:
- Select the close column and then call the
unstack
method. - Call the
pct_change
method. - Call the
var
method and then take the inverse. - Call the
pie
method with theinverse_variance Series
, the plot labels, and a display format. - In the cell that you called the
pie
method, call thetitle
method with a title.
close_prices = history['close'].unstack(level=0)
daily_returns = close_prices.pct_change()
inverse_variance = 1 / daily_returns.var()
plt.pie(inverse_variance, labels=inverse_variance.index, autopct='%1.1f%%')
plt.title('Banking Stocks and Bank Sector ETF Allocation');
The Jupyter Notebook displays the pie chart.
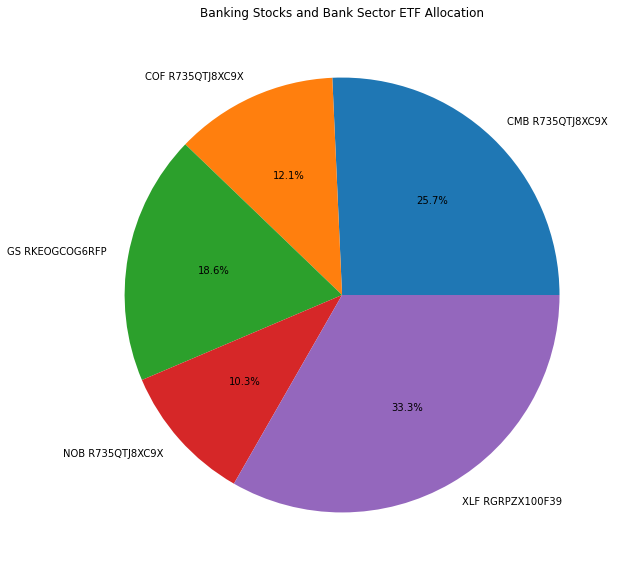