Key Concepts
Getting Started
Introduction
The Research Environment is a Jupyter notebook-based, interactive commandline environment where you can access our data through the QuantBook
class. The environment supports both Python and C#. If you use Python, you can import code from the code files in your project into the Research Environment to aid development.
Before you run backtests, we recommend testing your hypothesis in the Research Environment. It's easier to perform data analysis and produce plots in the Research Environment than in a backtest.
Before backtesting or live trading with machine learning models, you may find it beneficial to train them in the Research Environment, save them in the Object Store, and then load them from the Object Store into the backtesting and live trading environment
In the Research Environment, you can also use the QuantConnect API to import your backtest results for further analysis.
Example
The following snippet demonstrates how to use the Research Environment to plot the price and Bollinger Bands of the S&P 500 index ETF, SPY:
The following snippet demonstrates how to use the Research Environment to print the price of the S&P 500 index ETF, SPY:
// Load the required assembly files and data types #load "../Initialize.csx" #load "../QuantConnect.csx" using QuantConnect; using QuantConnect.Data; using QuantConnect.Algorithm; using QuantConnect.Research; // Create a QuantBook var qb = new QuantBook(); // Create a security subscription var symbol = qb.AddEquity("SPY").Symbol; // Request some historical data var history = qb.History(symbol, 70, Resolution.Daily); foreach (var tradeBar in history) { Console.WriteLine($"{tradeBar.EndTime} :: {tradeBar.ToString()}"); }
# Create a QuantBook qb = QuantBook() # Create a security subscription spy = qb.add_equity("SPY") # Request some historical data history = qb.history(qb.securities.keys, 360, Resolution.DAILY) # Calculate the Bollinger Bands bbdf = qb.indicator(BollingerBands(30, 2), spy.symbol, 360, Resolution.DAILY) # Plot the data bbdf.drop('standarddeviation', 1).plot()
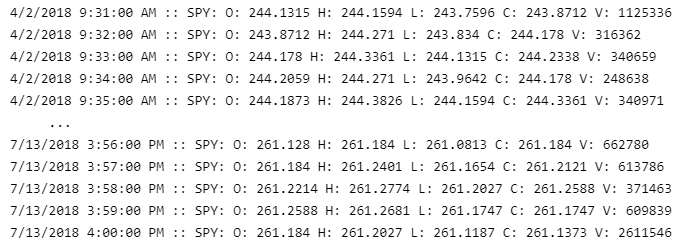
Open Notebooks
Each new project you create contains a notebook file by default. Follow these steps to open the notebook:
- Open the project.
- In the right navigation menu, click the
Explorer icon.
- In the Explorer panel, expand the section.
- Click the Research.ipynbresearch.ipynb file.
Run Notebook Cells
Notebooks are a collection of cells where you can write code snippets or MarkDown. To execute a cell, press Shift+Enter.
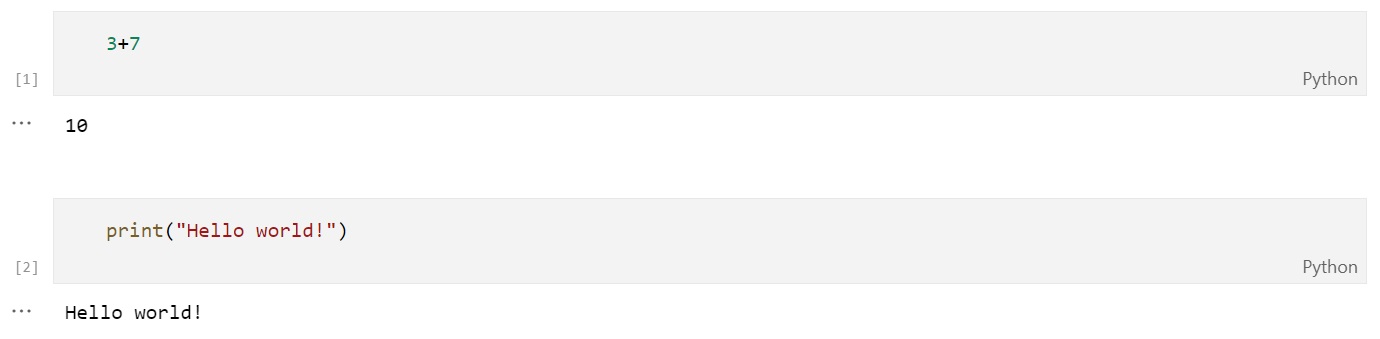
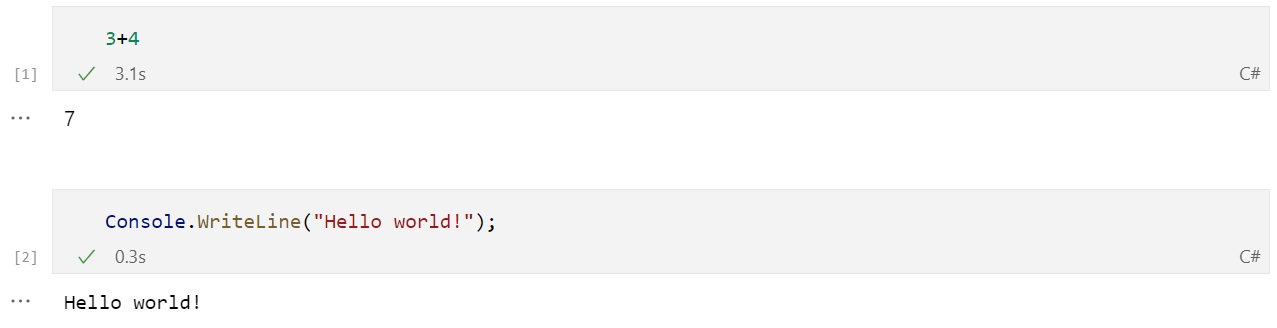
The following describes some helpful keyboard shortcuts to speed up your research:
Keyboard Shortcut | Description |
---|---|
Shift+Enter | Run the selected cell. |
a | Insert a cell above the selected cell. |
b | Insert a cell below the selected cell. |
x | Cut the selected cell. |
v | Paste the copied or cut cell. |
z | Undo cell actions. |
Stop Nodes
You need stop node permissions to stop research nodes in the cloud.
Follow these steps to stop a research node:
- Open the project.
- In the right navigation menu, click the
Resources icon.
- Click the button next to the research node you want to stop.
Add Notebooks
Follow these steps to add notebook files to a project:
- Open the project.
- In the right navigation menu, click the
Explorer icon.
- In the Explorer panel, expand the section.
- Click the
New File icon.
- Enter fileName.ipynb.
- Press Enter.
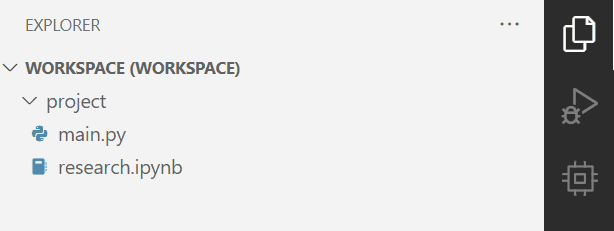
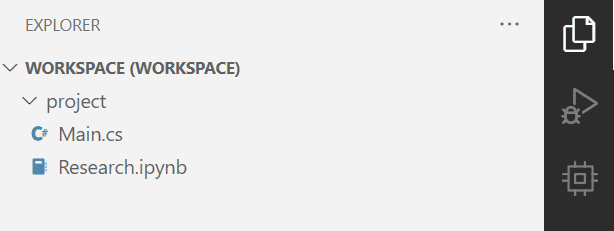
Rename Notebooks
Follow these steps to rename a notebook in a project:
- Open the project.
- In the right navigation menu, click the
Explorer icon.
- In the Explorer panel, right-click the notebook you want to rename and then click .
- Enter the new name and then press .
Delete Notebooks
Follow these steps to delete a notebook in a project:
- Open the project.
- In the right navigation menu, click the
Explorer icon.
- In the Explorer panel, right-click the notebook you want to delete and then click .
- Click .
Learn Jupyter
The following table lists some helpful resources to learn Jupyter:
Type | Name | Producer |
---|---|---|
Text | Jupyter Tutorial | tutorialspoint |
Text | Jupyter Notebook Tutorial: The Definitive Guide | DataCamp |
Text | An Introduction to DataFrame | Microsoft Developer Blogs |