Datasets
Crypto Futures
Create Subscriptions
Follow these steps to subscribe to a perpetual Crypto Futures contract:
- Load the assembly files and data types in their own cell.
- Import the data types.
- Create a
QuantBook
. - (Optional) Set the time zone to the data time zone.
- Call the
AddCryptoFuture
add_crypto_future
method with a ticker and then save a reference to the Crypto FutureSymbol
.
#load "../Initialize.csx"
#load "../QuantConnect.csx" #r "../Microsoft.Data.Analysis.dll" using QuantConnect; using QuantConnect.Data; using QuantConnect.Algorithm; using QuantConnect.Research; using QuantConnect.Indicators; using QuantConnect.Securities.CryptoFuture; using Microsoft.Data.Analysis;
var qb = new QuantBook();
qb = QuantBook()
qb.set_time_zone(TimeZones.UTC);
qb.set_time_zone(TimeZones.UTC)
var btcusd = qb.AddCryptoFuture("BTCUSD").Symbol; var ethusd = qb.AddCryptoFuture("ETHUSD").Symbol;
btcusd = qb.add_crypto_future("BTCUSD").symbol ethusd = qb.add_crypto_future("ETHUSD").symbol
To view the supported assets in the Crypto Futures datasets, see the Data Explorer.
Get Historical Data
You need a subscription before you can request historical data for a security. You can request an amount of historical data based on a trailing number of bars, a trailing period of time, or a defined period of time. You can also request historical data for a single contract, a subset of the contracts you created subscriptions for in your notebook, or all of the contracts in your notebook.
Trailing Number of Bars
To get historical data for a number of trailing bars, call the History
history
method with the Symbol
object(s) and an integer.
// Slice objects var singleHistorySlice = qb.History(btcusd, 10); var subsetHistorySlice = qb.History(new[] {btcusd, ethusd}, 10); var allHistorySlice = qb.History(10); // TradeBar objects var singleHistoryTradeBars = qb.History<TradeBar>(btcusd, 10); var subsetHistoryTradeBars = qb.History<TradeBar>(new[] {btcusd, ethusd}, 10); var allHistoryTradeBars = qb.History<TradeBar>(qb.Securities.Keys, 10); // QuoteBar objects var singleHistoryQuoteBars = qb.History<QuoteBar>(btcusd, 10); var subsetHistoryQuoteBars = qb.History<QuoteBar>(new[] {btcusd, ethusd}, 10); var allHistoryQuoteBars = qb.History<QuoteBar>(qb.Securities.Keys, 10);
# DataFrame of trade and quote data single_history_df = qb.history(btcusd, 10) subset_history_df = qb.history([btcusd, ethusd], 10) all_history_df = qb.history(qb.securities.keys(), 10) # DataFrame of trade data single_history_trade_bar_df = qb.history(TradeBar, btcusd, 10) subset_history_trade_bar_df = qb.history(TradeBar, [btcusd, ethusd], 10) all_history_trade_bar_df = qb.history(TradeBar, qb.securities.keys(), 10) # DataFrame of quote data single_history_quote_bar_df = qb.history(QuoteBar, btcusd, 10) subset_history_quote_bar_df = qb.history(QuoteBar, [btcusd, ethusd], 10) all_history_quote_bar_df = qb.history(QuoteBar, qb.securities.keys(), 10) # Slice objects all_history_slice = qb.history(10) # TradeBar objects single_history_trade_bars = qb.history[TradeBar](btcusd, 10) subset_history_trade_bars = qb.history[TradeBar]([btcusd, ethusd], 10) all_history_trade_bars = qb.history[TradeBar](qb.securities.keys(), 10) # QuoteBar objects single_history_quote_bars = qb.history[QuoteBar](btcusd, 10) subset_history_quote_bars = qb.history[QuoteBar]([btcusd, ethusd], 10) all_history_quote_bars = qb.history[QuoteBar](qb.securities.keys(), 10)
Trailing Period of Time
To get historical data for a trailing period of time, call the History
history
method with the Symbol
object(s) and a TimeSpan
timedelta
.
// Slice objects var singleHistorySlice = qb.History(btcusd, TimeSpan.FromDays(3)); var subsetHistorySlice = qb.History(new[] {btcusd, ethusd}, TimeSpan.FromDays(3)); var allHistorySlice = qb.History(10); // TradeBar objects var singleHistoryTradeBars = qb.History<TradeBar>(btcusd, TimeSpan.FromDays(3)); var subsetHistoryTradeBars = qb.History<TradeBar>(new[] {btcusd, ethusd}, TimeSpan.FromDays(3)); var allHistoryTradeBars = qb.History<TradeBar>(TimeSpan.FromDays(3)); // QuoteBar objects var singleHistoryQuoteBars = qb.History<QuoteBar>(btcusd, TimeSpan.FromDays(3), Resolution.Minute); var subsetHistoryQuoteBars = qb.History<QuoteBar>(new[] {btcusd, ethusd}, TimeSpan.FromDays(3), Resolution.Minute); var allHistoryQuoteBars = qb.History<QuoteBar>(qb.Securities.Keys, TimeSpan.FromDays(3), Resolution.Minute); // Tick objects var singleHistoryTicks = qb.History<Tick>(btcusd, TimeSpan.FromDays(3), Resolution.Tick); var subsetHistoryTicks = qb.History<Tick>(new[] {btcusd, ethusd}, TimeSpan.FromDays(3), Resolution.Tick); var allHistoryTicks = qb.History<Tick>(qb.Securities.Keys, TimeSpan.FromDays(3), Resolution.Tick);
# DataFrame of trade and quote data single_history_df = qb.history(btcusd, timedelta(days=3)) subset_history_df = qb.history([btcusd, ethusd], timedelta(days=3)) all_history_df = qb.history(qb.securities.keys(), timedelta(days=3)) # DataFrame of trade data single_history_trade_bar_df = qb.history(TradeBar, btcusd, timedelta(days=3)) subset_history_trade_bar_df = qb.history(TradeBar, [btcusd, ethusd], timedelta(days=3)) all_history_trade_bar_df = qb.history(TradeBar, qb.securities.keys(), timedelta(days=3)) # DataFrame of quote data single_history_quote_bar_df = qb.history(QuoteBar, btcusd, timedelta(days=3)) subset_history_quote_bar_df = qb.history(QuoteBar, [btcusd, ethusd], timedelta(days=3)) all_history_quote_bar_df = qb.history(QuoteBar, qb.securities.keys(), timedelta(days=3)) # DataFrame of tick data single_history_tick_df = qb.history(btcusd, timedelta(days=3), Resolution.TICK) subset_history_tick_df = qb.history([btcusd, ethusd], timedelta(days=3), Resolution.TICK) all_history_tick_df = qb.history(qb.securities.keys(), timedelta(days=3), Resolution.TICK) # Slice objects all_history_slice = qb.history(timedelta(days=3)) # TradeBar objects single_history_trade_bars = qb.history[TradeBar](btcusd, timedelta(days=3)) subset_history_trade_bars = qb.history[TradeBar]([btcusd, ethusd], timedelta(days=3)) all_history_trade_bars = qb.history[TradeBar](qb.securities.keys(), timedelta(days=3)) # QuoteBar objects single_history_quote_bars = qb.history[QuoteBar](btcusd, timedelta(days=3), Resolution.MINUTE) subset_history_quote_bars = qb.history[QuoteBar]([btcusd, ethusd], timedelta(days=3), Resolution.MINUTE) all_history_quote_bars = qb.history[QuoteBar](qb.securities.keys(), timedelta(days=3), Resolution.MINUTE) # Tick objects single_history_ticks = qb.history[Tick](btcusd, timedelta(days=3), Resolution.TICK) subset_history_ticks = qb.history[Tick]([btcusd, ethusd], timedelta(days=3), Resolution.TICK) all_history_ticks = qb.history[Tick](qb.securities.keys(), timedelta(days=3), Resolution.TICK)
Defined Period of Time
To get historical data for a specific period of time, call the History
method with the Symbol
object(s), a start DateTime
datetime
, and an end DateTime
datetime
. The start and end times you provide are based in the notebook time zone.
var startTime = new DateTime(2021, 1, 1); var endTime = new DateTime(2021, 2, 1); // Slice objects var singleHistorySlice = qb.History(btcusd, startTime, endTime); var subsetHistorySlice = qb.History(new[] {btcusd, ethusd}, startTime, endTime); var allHistorySlice = qb.History(qb.Securities.Keys, startTime, endTime); // TradeBar objects var singleHistoryTradeBars = qb.History<TradeBar>(btcusd, startTime, endTime); var subsetHistoryTradeBars = qb.History<TradeBar>(new[] {btcusd, ethusd}, startTime, endTime); var allHistoryTradeBars = qb.History<TradeBar>(qb.Securities.Keys, startTime, endTime); // QuoteBar objects var singleHistoryQuoteBars = qb.History<QuoteBar>(btcusd, startTime, endTime, Resolution.Minute); var subsetHistoryQuoteBars = qb.History<QuoteBar>(new[] {btcusd, ethusd}, startTime, endTime, Resolution.Minute); var allHistoryQuoteBars = qb.History<QuoteBar>(qb.Securities.Keys, startTime, endTime, Resolution.Minute); // Tick objects var singleHistoryTicks = qb.History<Tick>(btcusd, startTime, endTime, Resolution.Tick); var subsetHistoryTicks = qb.History<Tick>(new[] {btcusd, ethusd}, startTime, endTime, Resolution.Tick); var allHistoryTicks = qb.History<Tick>(qb.Securities.Keys, startTime, endTime, Resolution.Tick);
start_time = datetime(2021, 1, 1) end_time = datetime(2021, 2, 1) # DataFrame of trade and quote data single_history_df = qb.history(btcusd, start_time, end_time) subset_history_df = qb.history([btcusd, ethusd], start_time, end_time) all_history_df = qb.history(qb.securities.keys(), start_time, end_time) # DataFrame of trade data single_history_trade_bar_df = qb.history(TradeBar, btcusd, start_time, end_time) subset_history_trade_bar_df = qb.history(TradeBar, [btcusd, ethusd], start_time, end_time) all_history_trade_bar_df = qb.history(TradeBar, qb.securities.keys(), start_time, end_time) # DataFrame of quote data single_history_quote_bar_df = qb.history(QuoteBar, btcusd, start_time, end_time) subset_history_quote_bar_df = qb.history(QuoteBar, [btcusd, ethusd], start_time, end_time) all_history_quote_bar_df = qb.history(QuoteBar, qb.securities.keys(), start_time, end_time) # DataFrame of tick data single_history_tick_df = qb.history(btcusd, start_time, end_time, Resolution.TICK) subset_history_tick_df = qb.history([btcusd, ethusd], start_time, end_time, Resolution.TICK) all_history_tick_df = qb.history(qb.securities.keys(), start_time, end_time, Resolution.TICK) # TradeBar objects single_history_trade_bars = qb.history[TradeBar](btcusd, start_time, end_time) subset_history_trade_bars = qb.history[TradeBar]([btcusd, ethusd], start_time, end_time) all_history_trade_bars = qb.history[TradeBar](qb.securities.keys(), start_time, end_time) # QuoteBar objects single_history_quote_bars = qb.history[QuoteBar](btcusd, start_time, end_time, Resolution.MINUTE) subset_history_quote_bars = qb.history[QuoteBar]([btcusd, ethusd], start_time, end_time, Resolution.MINUTE) all_history_quote_bars = qb.history[QuoteBar](qb.securities.keys(), start_time, end_time, Resolution.MINUTE) # Tick objects single_history_ticks = qb.history[Tick](btcusd, start_time, end_time, Resolution.TICK) subset_history_ticks = qb.history[Tick]([btcusd, ethusd], start_time, end_time, Resolution.TICK) all_history_ticks = qb.history[Tick](qb.securities.keys(), start_time, end_time, Resolution.TICK)
Wrangle Data
You need some historical data to perform wrangling operations. The process to manipulate the historical data depends on its data type. To display pandas
objects, run a cell in a notebook with the pandas
object as the last line. To display other data formats, call the print
method.
You need some historical data to perform wrangling operations. Use LINQ to wrangle the data and then call the Console.WriteLine
method in a Jupyter Notebook to display the data. The process to manipulate the historical data depends on its data type.
DataFrame Objects
If the History
history
method returns a DataFrame
, the first level of the DataFrame
index is the encoded Crypto Future Symbol and the second level is the EndTime
end_time
of the data sample. The columns of the DataFrame
are the data properties.
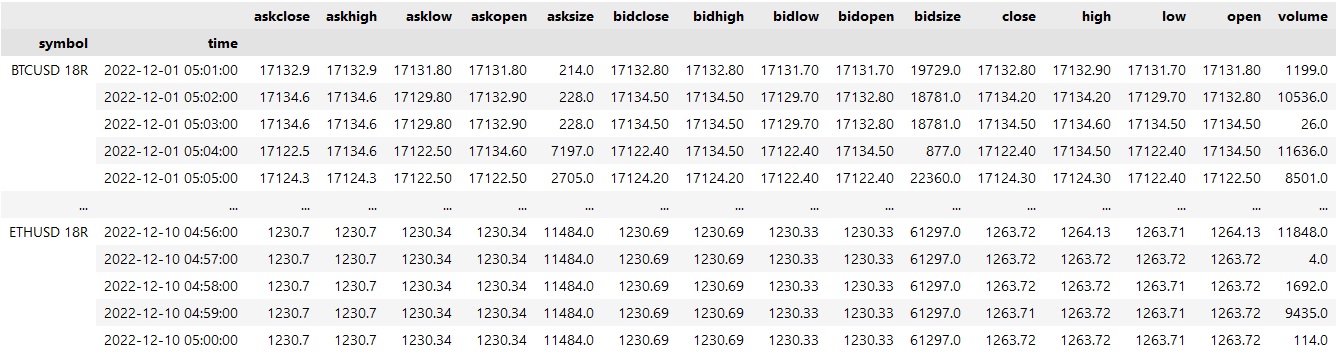
To select the historical data of a single Crypto Future, index the loc
property of the DataFrame
with the Crypto Future Symbol
.
all_history_df.loc[btcusd] # or all_history_df.loc['BTCUSD']
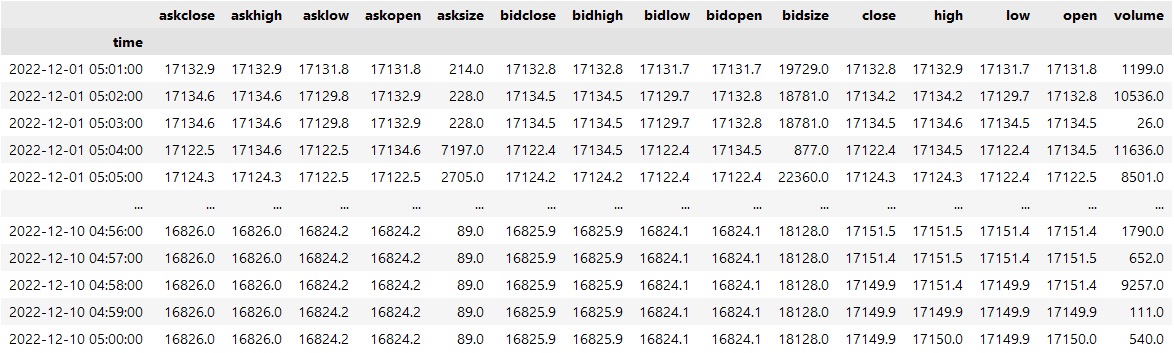
To select a column of the DataFrame
, index it with the column name.
all_history_df.loc[btcusd]['close']
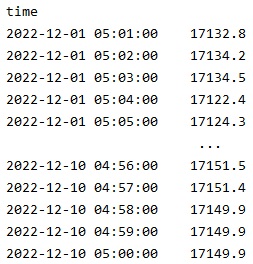
If you request historical data for multiple Crypto Futures contracts, you can transform the DataFrame
so that it's a time series of close values for all of the Crypto Futures contracts. To transform the DataFrame
, select the column you want to display for each Crypto Futures contract and then call the unstack method.
all_history_df['close'].unstack(level=0)
The DataFrame
is transformed so that the column indices are the Symbol
of each Crypto Futures contract and each row contains the close value.
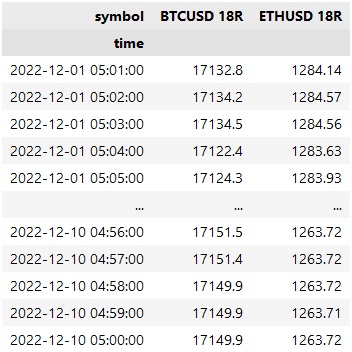
The historical data methods don't return DataFrame objects, but you can create one for efficient vectorized data wrangling.
using Microsoft.Data.Analysis; var columns = new DataFrameColumn[] { new PrimitiveDataFrameColumn("Time", history.Select(x => x[btcusd].EndTime)), new DecimalDataFrameColumn("BTCUSD Open", history.Select(x => x[btcusd].Open)), new DecimalDataFrameColumn("BTCUSD High", history.Select(x => x[btcusd].High)), new DecimalDataFrameColumn("BTCUSD Low", history.Select(x => x[btcusd].Low)), new DecimalDataFrameColumn("BTCUSD Close", history.Select(x => x[btcusd].Close)) }; var df = new DataFrame(columns); df
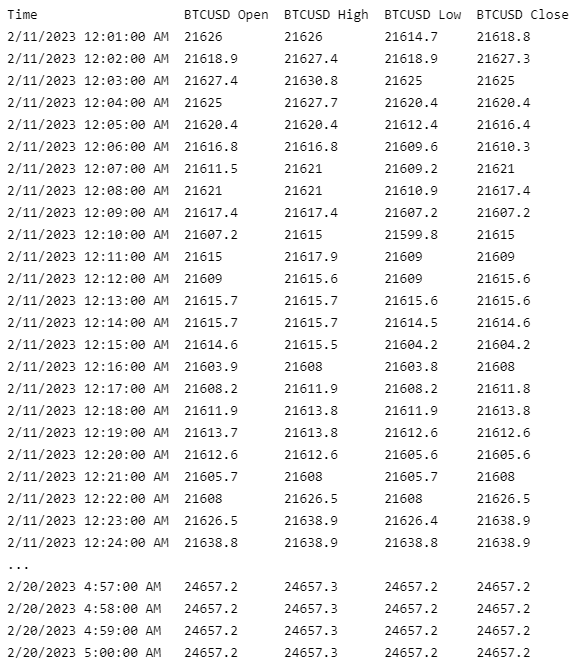
To select a particular column of the DataFrame, index it with the column name.
df["BTCUSD close"]
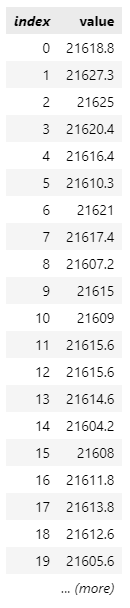
Slice Objects
If the History
history
method returns Slice
objects, iterate through the Slice
objects to get each one. The Slice
objects may not have data for all of your Crypto Future subscriptions. To avoid issues, check if the Slice
contains data for your Crypto Futures contract before you index it with the Crypto Future Symbol
.
foreach (var slice in allHistorySlice) { if (slice.Bars.ContainsKey(btcusd)) { var tradeBar = slice.Bars[btcusd]; } if (slice.QuoteBars.ContainsKey(btcusd)) { var quoteBar = slice.QuoteBars[btcusd]; } }
for slice in all_history_slice: if slice.bars.contains_key(btcusd): trade_bar = slice.bars[btcusd] if slice.quote_bars.contains_key(btcusd): quote_bar = slice.quote_bars[btcusd]
You can also iterate through each TradeBar
and QuoteBar
in the Slice
.
foreach (var slice in allHistorySlice) { foreach (var kvp in slice.Bars) { var symbol = kvp.Key; var tradeBar = kvp.Value; } foreach (var kvp in slice.QuoteBars) { var symbol = kvp.Key; var quoteBar = kvp.Value; } }
for slice in all_history_slice: for kvp in slice.bars: symbol = kvp.key trade_bar = kvp.value for kvp in slice.quote_bars: symbol = kvp.key quote_bar = kvp.value
You can also use LINQ to select each TradeBar
in the Slice
for a given Symbol
.
var tradeBars = allHistorySlice.Where(slice => slice.Bars.ContainsKey(btcusd)).Select(slice => slice.Bars[btcusd]);
TradeBar Objects
If the History
history
method returns TradeBar
objects, iterate through the TradeBar
objects to get each one.
foreach (var tradeBar in singleHistoryTradeBars) { Console.WriteLine(tradeBar); }
for trade_bar in single_history_trade_bars: print(trade_bar)
If the History
history
method returns TradeBars
, iterate through the TradeBars
to get the TradeBar
of each Crypto Futures contract. The TradeBars
may not have data for all of your Crypto Future subscriptions. To avoid issues, check if the TradeBars
object contains data for your security before you index it with the Crypto Future Symbol
.
foreach (var tradeBars in allHistoryTradeBars) { if (tradeBars.ContainsKey(btcusd)) { var tradeBar = tradeBars[btcusd]; } }
for trade_bars in all_history_trade_bars: if trade_bars.contains_key(btcusd): trade_bar = trade_bars[btcusd]
You can also iterate through each of the TradeBars
.
foreach (var tradeBars in allHistoryTradeBars) { foreach (var kvp in tradeBars) { var symbol = kvp.Key; var tradeBar = kvp.Value; } }
for trade_bars in all_history_trade_bars: for kvp in trade_bars: symbol = kvp.Key trade_bar = kvp.Value
QuoteBar Objects
If the History
history
method returns QuoteBar
objects, iterate through the QuoteBar
objects to get each one.
foreach (var quoteBar in singleHistoryQuoteBars) { Console.WriteLine(quoteBar); }
for quote_bar in single_history_quote_bars: print(quote_bar)
If the History
history
method returns QuoteBars
, iterate through the QuoteBars
to get the QuoteBar
of each Crypto Futures contract. The QuoteBars
may not have data for all of your Crypto Future subscriptions. To avoid issues, check if the QuoteBars
object contains data for your security before you index it with the Crypto Future Symbol
.
foreach (var quoteBars in allHistoryQuoteBars) { if (quoteBars.ContainsKey(btcusd)) { var quoteBar = quoteBars[btcusd]; } }
for quote_bars in all_history_quote_bars: if quote_bars.contains_key(btcusd): quote_bar = quote_bars[btcusd]
You can also iterate through each of the QuoteBars
.
foreach (var quoteBars in allHistoryQuoteBars) { foreach (var kvp in quoteBars) { var symbol = kvp.Key; var quoteBar = kvp.Value; } }
for quote_bars in all_history_quote_bars: for kvp in quote_bars: symbol = kvp.key quote_bar = kvp.value
Tick Objects
If the History
history
method returns Tick
TICK
objects, iterate through the Tick
TICK
objects to get each one.
foreach (var tick in singleHistoryTicks) { Console.WriteLine(tick); }
for tick in single_history_ticks: print(tick)
If the History
history
method returns Ticks
, iterate through the Ticks
to get the Tick
TICK
of each Crypto Futures contract. The Ticks
may not have data for all of your Crypto Future subscriptions. To avoid issues, check if the Ticks
object contains data for your security before you index it with the Crypto Future Symbol
.
foreach (var ticks in allHistoryTicks) { if (ticks.ContainsKey(btcusd)) { var tick = ticks[btcusd]; } }
for ticks in all_history_ticks: if ticks.contains_key(btcusd): ticks = ticks[btcusd]
You can also iterate through each of the Ticks
.
foreach (var ticks in allHistoryTicks) { foreach (var kvp in ticks) { var symbol = kvp.Key; var tick = kvp.Value; } }
for ticks in all_history_ticks: for kvp in ticks: symbol = kvp.key tick = kvp.value
The Ticks
objects only contain the last tick of each security for that particular timeslice
Plot Data
You need some historical Crypto Futures data to produce plots. You can use many of the supported plotting librariesPlot.NET package to visualize data in various formats. For example, you can plot candlestick and line charts.
Candlestick Chart
Follow these steps to plot candlestick charts:
- Get some historical data.
- Import the
plotly
Plot.NET
library. - Create a
Candlestick
. - Create a
Layout
. - Create the
Figure
. - Assign the
Layout
to the chart. - Show the
Figure
.
history = qb.history(btcusd, datetime(2021, 11, 23), datetime(2021, 12, 8), Resolution.DAILY).loc[btcusd]
var history = qb.History<TradeBar>(btcusd, new DateTime(2021, 11, 23), new DateTime(2021, 12, 8), Resolution.Daily);
import plotly.graph_objects as go
#r "../Plotly.NET.dll" using Plotly.NET; using Plotly.NET.LayoutObjects;
candlestick = go.Candlestick(x=history.index, open=history['open'], high=history['high'], low=history['low'], close=history['close'])
var chart = Chart2D.Chart.Candlestick<decimal, decimal, decimal, decimal, DateTime, string>( history.Select(x => x.Open), history.Select(x => x.High), history.Select(x => x.Low), history.Select(x => x.Close), history.Select(x => x.EndTime) );
layout = go.Layout(title=go.layout.Title(text='BTCUSD 18R OHLC'), xaxis_title='Date', yaxis_title='Price', xaxis_rangeslider_visible=False)
LinearAxis xAxis = new LinearAxis(); xAxis.SetValue("title", "Time"); LinearAxis yAxis = new LinearAxis(); yAxis.SetValue("title", "Price ($)"); Title title = Title.init("BTCUSD 18R OHLC"); Layout layout = new Layout(); layout.SetValue("xaxis", xAxis); layout.SetValue("yaxis", yAxis); layout.SetValue("title", title);
fig = go.Figure(data=[candlestick], layout=layout)
chart.WithLayout(layout);
fig.show()
HTML(GenericChart.toChartHTML(chart))
Candlestick charts display the open, high, low, and close prices of the security.
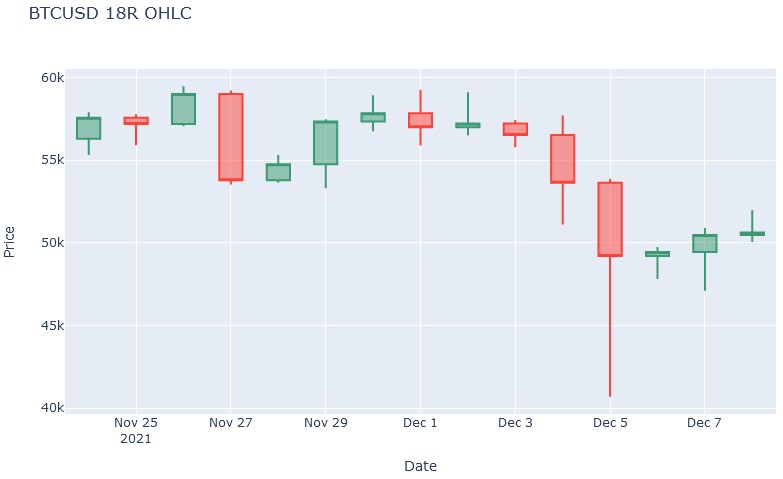
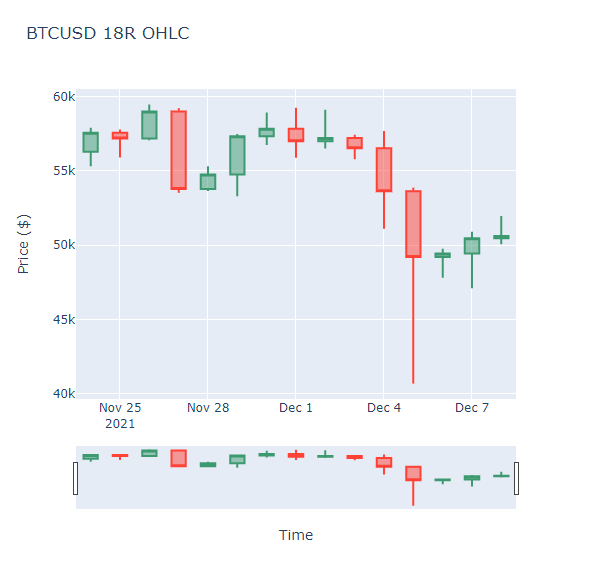
Line Chart
Follow these steps to plot line charts using built-in methodsPlotly.NET
package:
- Get some historical data.
- Select the data to plot.
- Call the
plot
method on thepandas
object. - Create
Line
charts. - Create a
Layout
. - Combine the charts and assign the
Layout
to the chart. - Show the plot.
history = qb.history([btcusd, ethusd], datetime(2021, 11, 23), datetime(2021, 12, 8), Resolution.DAILY)
var history = qb.history(new List<Symbol> { btcusd, ethusd }, new DateTime(2021, 11, 23), new DateTime(2021, 12, 8), Resolution.DAILY);
volume = history['volume'].unstack(level=0)
volume.plot(title="Volume", figsize=(15, 10))
var chart1 = Chart2D.Chart.Line<DateTime, decimal, string>( history.Select(x => x[btcusd].EndTime), history.Select(x => x[btcusd].Volume), Name: "BTCUSD 18R" ); var chart2 = Chart2D.Chart.Line<DateTime, decimal, string>( history.Select(x => x[ethusd].EndTime), history.Select(x => x[ethusd].Volume), Name: "ETHUSD 18R" );
LinearAxis xAxis = new LinearAxis(); xAxis.SetValue("title", "Time"); LinearAxis yAxis = new LinearAxis(); yAxis.SetValue("title", "Volume"); Title title = Title.init("BTCUSD 18R & ETHUSD 18R Volume"); Layout layout = new Layout(); layout.SetValue("xaxis", xAxis); layout.SetValue("yaxis", yAxis); layout.SetValue("title", title);
var chart = Plotly.NET.Chart.Combine(new []{chart1, chart2}); chart.WithLayout(layout);
plt.show()
HTML(GenericChart.toChartHTML(chart))
Line charts display the value of the property you selected in a time series.
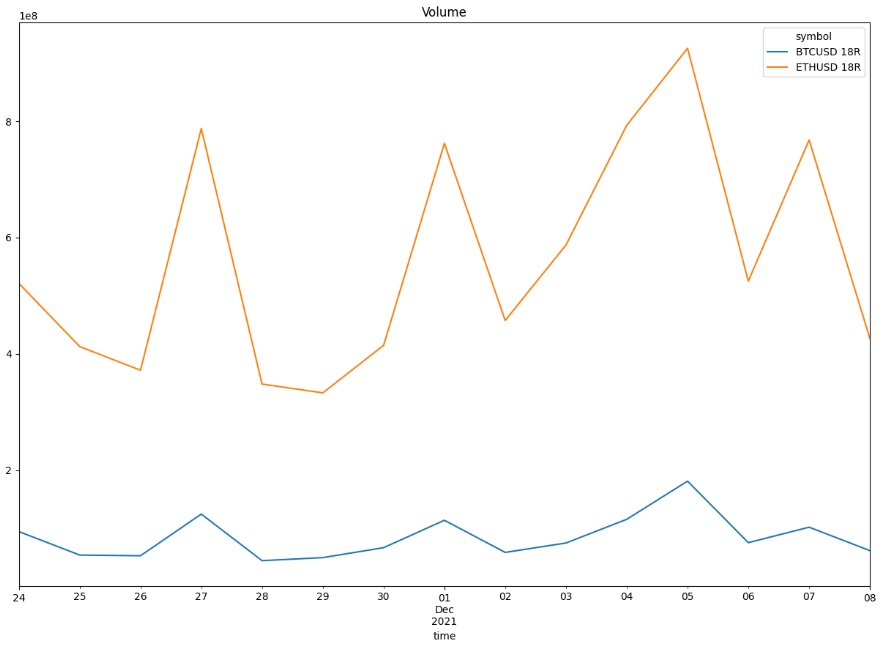
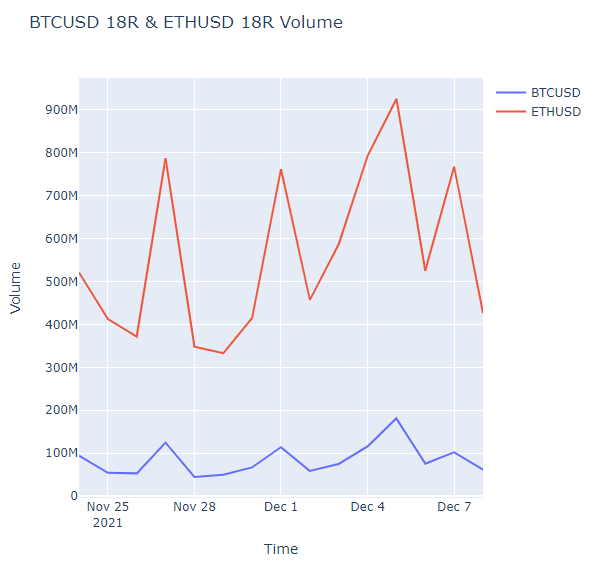