Indicators
Combining Indicators
Create Subscriptions
You need to subscribe to some market data in order to calculate indicator values.
var qb = new QuantBook(); var symbol = qb.AddEquity("SPY").Symbol;
qb = QuantBook() symbol = qb.add_equity("SPY").symbol
Create Indicator Timeseries
You need to subscribe to some market data and create a composite indicator in order to calculate a timeseries of indicator values. In this example, use a 10-period SimpleMovingAverage
of a 10-period RelativeStrengthIndex
indicator.
var rsi = new RelativeStrengthIndex(10); var sma = new SimpleMovingAverage(10); var smaOfRsi = IndicatorExtensions.Of(sma, rsi);
rsi = RelativeStrengthIndex(10) sma = SimpleMovingAverage(10) sma_of_rsi = IndicatorExtensions.of(sma, rsi)
Follow these steps to create an indicator timeseries:
- Get some historical data.
- Create a
RollingWindow
for each attribute of the indicator to hold their values. - Attach a handler method to the indicator that updates the
RollingWindow
objects. - Iterate the historical market data to update the indicators and the
RollingWindow
s. - Display the data.
- Populate a
DataFrame
with the data in theRollingWindow
objects.
var history = qb.History(symbol, 70, Resolution.Daily);
history = qb.history[TradeBar](symbol, 70, Resolution.DAILY)
In this example, save 50 data points.
var time = new RollingWindow<DateTime>(50); var window = new Dictionary<string, RollingWindow<decimal>>(); window["SMA Of RSI"] = new RollingWindow<decimal>(50); window["rollingsum"] = new RollingWindow<decimal>(50);
window = {} window['time'] = RollingWindow[DateTime](50) window["SMA Of RSI"] = RollingWindow[float](50) window["rollingsum"] = RollingWindow[float](50)
smaOfRsi.Updated += (sender, updated) => { var indicator = (SimpleMovingAverage)sender; // Use terminal indicator class. time.Add(updated.EndTime); window["SMA Of RSI"].Add(updated); window["rollingsum"].Add(indicator.RollingSum); };
def update_sma_of_rsi_window(sender: object, updated: IndicatorDataPoint) -> None: indicator = sender window['time'].add(updated.end_time) window["SMA Of RSI"].add(updated.value) window["rollingsum"].add(indicator.rolling_sum.current.value) sma_of_rsi.updated += UpdateSmaOfRsiWindow
When the indicator receives new data, the preceding handler method adds the new IndicatorDataPoint
values into the respective RollingWindow
.
foreach(var bar in history){ // Update the base indicators, the composite indicator will update automatically when the base indicator is updated. rsi.Update(bar.EndTime, bar.Close); }
for bar in history: rsi.update(bar.end_time, bar.close)
Console.WriteLine($"time,{string.Join(',', window.Select(kvp => kvp.Key))}"); foreach (var i in Enumerable.Range(0, 5).Reverse()) { var data = string.Join(", ", window.Select(kvp => Math.Round(kvp.Value[i],6))); Console.WriteLine($"{time[i]:yyyyMMdd}, {data}"); }
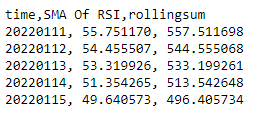
sma_of_rsi_dataframe = pd.DataFrame(window).set_index('time')
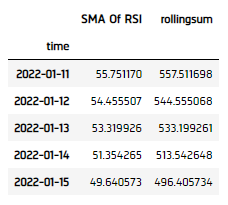
Plot Indicators
Jupyter Notebooks don't currently support libraries to plot historical data, but we are working on adding the functionality. Until the functionality is added, use Python to plot composite indicators.
Follow these steps to plot the indicator values:
- Select the columns/features to plot.
- Call the
plot
method. - Show the plots.
sma_of_rsi_plot = sma_of_rsi_dataframe[["SMA Of RSI"]]
sma_of_rsi_plot.plot(title="SPY SMA(10) of RSI(10)", figsize=(15, 10))
plt.show()
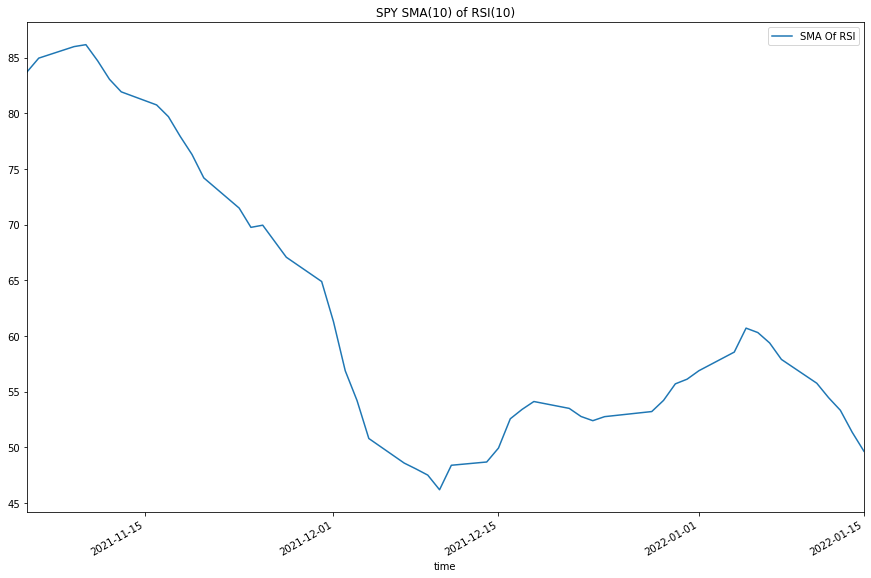