Indicators
Custom Resolutions
Create Subscriptions
You need to subscribe to some market data in order to calculate indicator values.
var qb = new QuantBook(); var symbol = qb.AddEquity("SPY").Symbol;
qb = QuantBook() symbol = qb.add_equity("SPY").symbol
Create Indicator Timeseries
You need to subscribe to some market data and create an indicator in order to calculate a timeseries of indicator values.
Follow these steps to create an indicator timeseries:
- Get some historical data.
- Create a data-point indicator.
- Create a
RollingWindow
for each attribute of the indicator to hold their values. - Attach a handler method to the indicator that updates the
RollingWindow
objects. - Create a
TradeBarConsolidator
to consolidate data into the custom resolution. - Attach a handler method to feed data into the consolidator and updates the indicator with the consolidated bars.
- Iterate through the historical market data and update the indicator.
- Display the data.
- Populate a
DataFrame
with the data in theRollingWindow
objects.
var history = qb.History(symbol, 70, Resolution.Daily);
history = qb.history[TradeBar](symbol, 70, Resolution.DAILY)
In this example, use a 20-period 2-standard-deviation BollingerBands
indicator.
var bb = new BollingerBands(20, 2);
bb = BollingerBands(20, 2)
var time = new RollingWindow<DateTime>(50); var window = new Dictionary<string, RollingWindow<decimal>>(); window["bollingerbands"] = new RollingWindow<decimal>(50); window["lowerband"] = new RollingWindow<decimal>(50); window["middleband"] = new RollingWindow<decimal>(50); window["upperband"] = new RollingWindow<decimal>(50); window["bandwidth"] = new RollingWindow<decimal>(50); window["percentb"] = new RollingWindow<decimal>(50); window["standarddeviation"] = new RollingWindow<decimal>(50); window["price"] = new RollingWindow<decimal>(50);
window = {} window['time'] = RollingWindow[DateTime](50) window["bollingerbands"] = RollingWindow[float](50) window["lowerband"] = RollingWindow[float](50) window["middleband"] = RollingWindow[float](50) window["upperband"] = RollingWindow[float](50) window["bandwidth"] = RollingWindow[float](50) window["percentb"] = RollingWindow[float](50) window["standarddeviation"] = RollingWindow[float](50) window["price"] = RollingWindow[float](50)
bb.Updated += (sender, updated) => { var indicator = (BollingerBands)sender; time.Add(updated.EndTime); window["bollingerbands"].Add(updated); window["lowerband"].Add(indicator.LowerBand); window["middleband"].Add(indicator.MiddleBand); window["upperband"].Add(indicator.UpperBand); window["bandwidth"].Add(indicator.BandWidth); window["percentb"].Add(indicator.PercentB); window["standarddeviation"].Add(indicator.StandardDeviation); window["price"].Add(indicator.Price); };
def update_bollinger_band_window(sender: object, updated: IndicatorDataPoint) -> None: indicator = sender window['time'].add(updated.end_time) window["bollingerbands"].add(updated.value) window["lowerband"].add(indicator.lower_band.current.value) window["middleband"].add(indicator.middle_band.current.value) window["upperband"].add(indicator.upper_band.current.value) window["bandwidth"].add(indicator.band_width.current.value) window["percentb"].add(indicator.percent_b.current.value) window["standarddeviation"].add(indicator.standard_deviation.current.value) window["price"].add(indicator.price.current.value) bb.updated += UpdateBollingerBandWindow
When the indicator receives new data, the preceding handler method adds the new IndicatorDataPoint
values into the respective RollingWindow
.
var consolidator = new TradeBarConsolidator(TimeSpan.FromDays(7));
consolidator = TradeBarConsolidator(timedelta(days=7))
consolidator.DataConsolidated += (sender, consolidated) => { bb.Update(consolidated.EndTime, consolidated.Close); };
consolidator.data_consolidated += lambda sender, consolidated: bb.update(consolidated.end_time, consolidated.close)
When the consolidator receives 7 days of data, the handler generates a 7-day TradeBar
and update the indicator.
foreach(var bar in history) { consolidator.Update(bar); }
for bar in history: consolidator.update(bar)
Console.WriteLine($"time,{string.Join(',', window.Select(kvp => kvp.Key))}"); foreach (var i in Enumerable.Range(0, 5).Reverse()) { var data = string.Join(", ", window.Select(kvp => Math.Round(kvp.Value[i],6))); Console.WriteLine($"{time[i]:yyyyMMdd}, {data}"); }

bb_dataframe = pd.DataFrame(window).set_index('time')
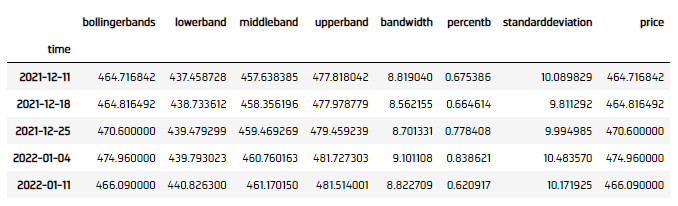
Plot Indicators
Jupyter Notebooks don't currently support libraries to plot historical data, but we are working on adding the functionality. Until the functionality is added, use Python to plot indicators.
Follow these steps to plot the indicator values:
- Select the columsn to plot.
- Call the
plot
method. - Show the plot.
df = bb_dataframe[['lowerband', 'middleband', 'upperband', 'price']]
df.plot()
plt.show()
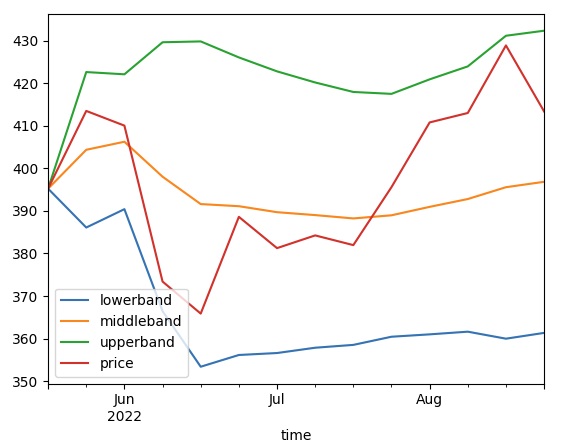