Key Concepts
Getting Started
Introduction
Quantitative trading is a method of trading where computer programs execute a set of defined trading rules in an automated fashion. Quants take a scientific approach to trading, applying concepts from mathematics, time series analysis, statistics, computer science, and machine learning. Compared to discretionary traders, quants can respond faster to new information and are at less risk to their emotions during trades. Since quants can concurrently trade many strategies while discretionary traders only have the mental bandwidth to trade a small number of concurrent strategies, quant traders can have more diversified portfolios.
Learn Programming
We aim to make it as easy as possible to use QuantConnect, but you still need to be able to program. The following table provides some resources to get you started:
Type | Name | Producer |
---|---|---|
Video | C# Fundamentals for Absolute Beginners | Microsoft |
Text | C# Jump Start - Advanced Concepts | Microsoft |
Video | Top 20 C# Questions | Microsoft |
Text | C# Tutorial | tutorialspoint |
Text | Introduction to Financial Python | QuantConnect |
Text/Video | Introduction to Python | |
Interactive | Code Academy - Python | Code Academy |
Text | Python Pandas Tutorial | tutorialspoint |
Enroll in Bootcamp
Bootcamp is an online coding experience where QuantConnect team and community members teach you to write your first algorithms. Bootcamp gives you step-by-step instructions on practical and beginner-friendly topics, so it's a great way to learn LEAN. The lessons cover many topics that you use as you write your own algorithms, including universe selection, indicators, and consolidators. To get started, check out the course library.
Example Algorithm
The following video demonstrates how to implement an algorithm that buys and holds an S&P 500 index ETF:
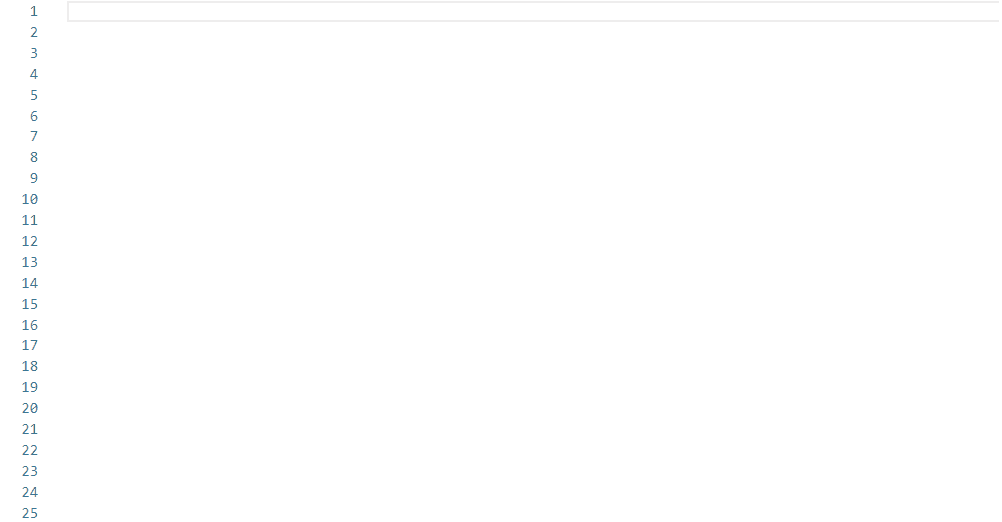
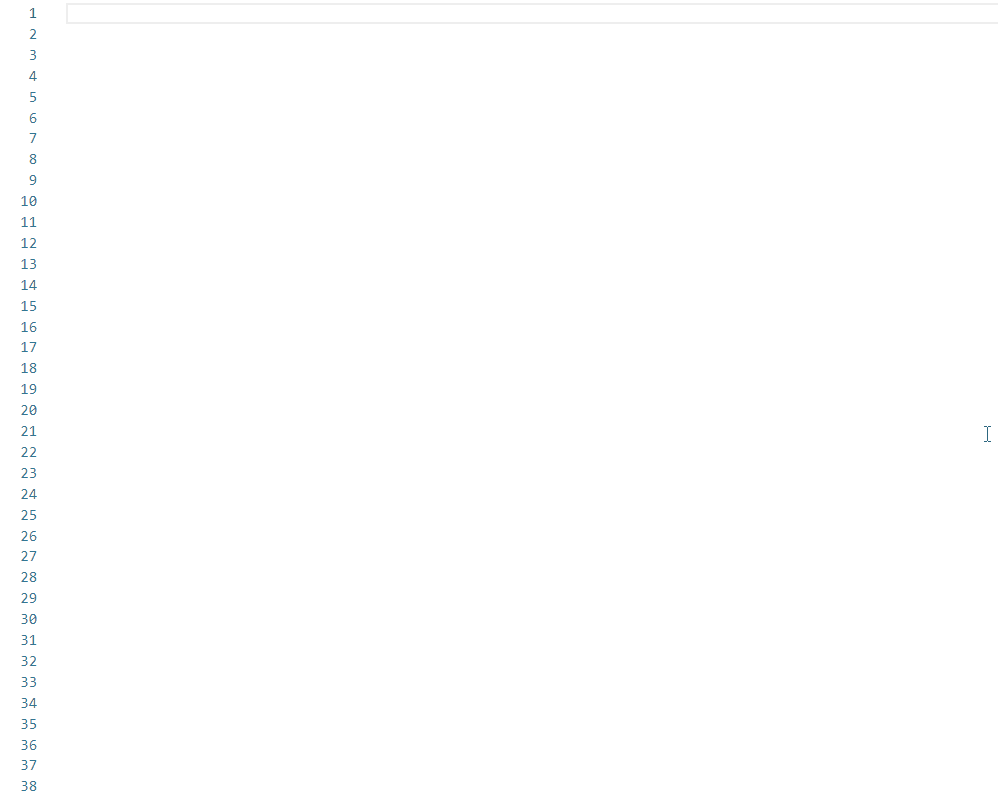
// Import all the functionality you need to run algorithms using QuantConnect.Data; // Define a trading algorithm that is a subclass of QCAlgorithm namespace QuantConnect.Algorithm.CSharp { public class MyAlgorithm : QCAlgorithm { // Define an Initialize method. // This method is the entry point of your algorithm where you define a series of settings. // LEAN only calls this method one time, at the start of your algorithm. public override void Initialize() { // Set the start and end dates SetStartDate(2018, 1, 1); SetEndDate(2022, 6, 1); // Set the starting cash balance to $100,000 USD SetCash(100000); // Add data for the S&P500 index ETF AddEquity("SPY"); } // Define an OnData method. // This method receives all the data you subscribe to in discrete time slices. // It's where you make trading decisions. public override void OnData(Slice slice) { // Allocate 100% of the portfolio to SPY if (!Portfolio.Invested) { SetHoldings("SPY", 1); } } } }
# Import all the functionality you need to run algorithms from AlgorithmImports import * # Define a trading algorithm that is a subclass of QCAlgorithm class MyAlgorithm(QCAlgorithm): # Define an Initialize method. # This method is the entry point of your algorithm where you define a series of settings. # LEAN only calls this method one time, at the start of your algorithm. def initialize(self) -> None: # Set start and end dates self.set_start_date(2018, 1, 1) self.set_end_date(2022, 6, 1) # Set the starting cash balance to $100,000 USD self.set_cash(100000) # Add data for the S&P500 index ETF self.add_equity("SPY") # Define an OnData method. # This method receives all the data you subscribe to in discrete time slices. # It's where you make trading decisions. def on_data(self, slice: Slice) -> None: # Allocate 100% of the portfolio to SPY if not self.portfolio.invested: self.set_holdings("SPY", 1)