Option Strategies
Protective Put
Introduction
A Protective Put consists of buying a long position in a stock and a long position in put Options for the same amount of stock. Protective puts aim to hedge the long position of a stock with a long ATM or slightly OTM put Option. At any time for American Options or at expiration for European Options, if the stock moves above the strike price, the Option contract becomes worthless but the long stock position acquires an unrealized gain. If the underlying price moves below the strike, you can exercise the Options contract, which sells your underlying position at the put Option strike price.
Implementation
Follow these steps to implement the protective put strategy:
- In the
Initialize
initialize
method, set the start date, end date, starting cash, and Options universe. - In the
OnData
on_data
method, select the Option contract. - In the
OnData
on_data
method, place the orders.
private Symbol _symbol; public override void Initialize() { SetStartDate(2014, 1, 1); SetEndDate(2014, 3, 1); SetCash(100000); UniverseSettings.Asynchronous = true; var option = AddOption("IBM"); _symbol = option.Symbol; option.SetFilter(universe => universe.IncludeWeeklys().NakedPut(30, 0)); }
def initialize(self) -> None: self.set_start_date(2014, 1, 1) self.set_end_date(2014, 3, 1) self.set_cash(100000) self.universe_settings.asynchronous = True option = self.add_option("IBM") self._symbol = option.symbol option.set_filter(lambda universe: universe.include_weeklys().naked_put(30, 0))
The NakedPut
naked_put
filter narrows the universe down to just the one contract you need to form a protective put.
public override void OnData(Slice slice) { if (Portfolio.Invested || !slice.OptionChains.TryGetValue(_symbol, out var chain)) { return; } // Find ATM put with the farthest expiry var expiry = chain.Max(x => x.Expiry); var atmPut = chain .Where(x => x.Right == OptionRight.Put && x.Expiry == expiry) .OrderBy(x => Math.Abs(x.Strike - chain.Underlying.Price)) .FirstOrDefault();
def on_data(self, slice: Slice) -> None: if self.portfolio.invested: return chain = slice.option_chains.get(self._symbol) if not chain: return # Find ATM put with the farthest expiry expiry = max([x.expiry for x in chain]) put_contracts = sorted([x for x in chain if x.right == OptionRight.PUT and x.expiry == expiry], key=lambda x: abs(chain.underlying.price - x.strike)) if not put_contracts: return atm_put = put_contracts[0]
Approach A: Call the OptionStrategies.ProtectivePut
OptionStrategies.protective_put
method with the details of each leg and then pass the result to the Buy
buy
method.
var protectivePut = OptionStrategies.ProtectivePut(_symbol, atmPut.Strike, expiry); Buy(protectivePut, 1);
protective_put = OptionStrategies.protective_put(self._symbol, atm_put.strike, expiry) self.buy(protective_put, 1)
Approach B: Create a list of Leg
objects and then call the Combo Market Ordercombo_market_order, Combo Limit Ordercombo_limit_order, or Combo Leg Limit Ordercombo_leg_limit_order method.
var legs = new List<Leg>() { Leg.Create(atmPut.Symbol, 1), Leg.Create(chain.Underlying.Symbol, chain.Underlying.SymbolProperties.ContractMultiplier) }; ComboMarketOrder(legs, 1);
legs = [ Leg.create(atm_put.symbol, 1), Leg.create(chain.underlying.symbol, chain.underlying.symbol_properties.contract_multiplier) ] self.combo_market_order(legs, 1)
Strategy Payoff
The payoff of the strategy is
$$ \begin{array}{rcll} P^{K}_T & = & (K - S_T)^{+}\\ P_T & = & (S_T - S_0 + P^{K}_T - P^{K}_0)\times m - fee \end{array} $$ $$ \begin{array}{rcll} \textrm{where} & P^{K}_T & = & \textrm{Put value at time T}\\ & S_T & = & \textrm{Underlying asset price at time T}\\ & K & = & \textrm{Put strike price}\\ & P_T & = & \textrm{Payout total at time T}\\ & S_0 & = & \textrm{Underlying asset price when the trade opened}\\ & P^{K}_0 & = & \textrm{Put price when the trade opened (credit received)}\\ & m & = & \textrm{Contract multiplier}\\ & T & = & \textrm{Time of expiration} \end{array} $$The following chart shows the payoff at expiration:
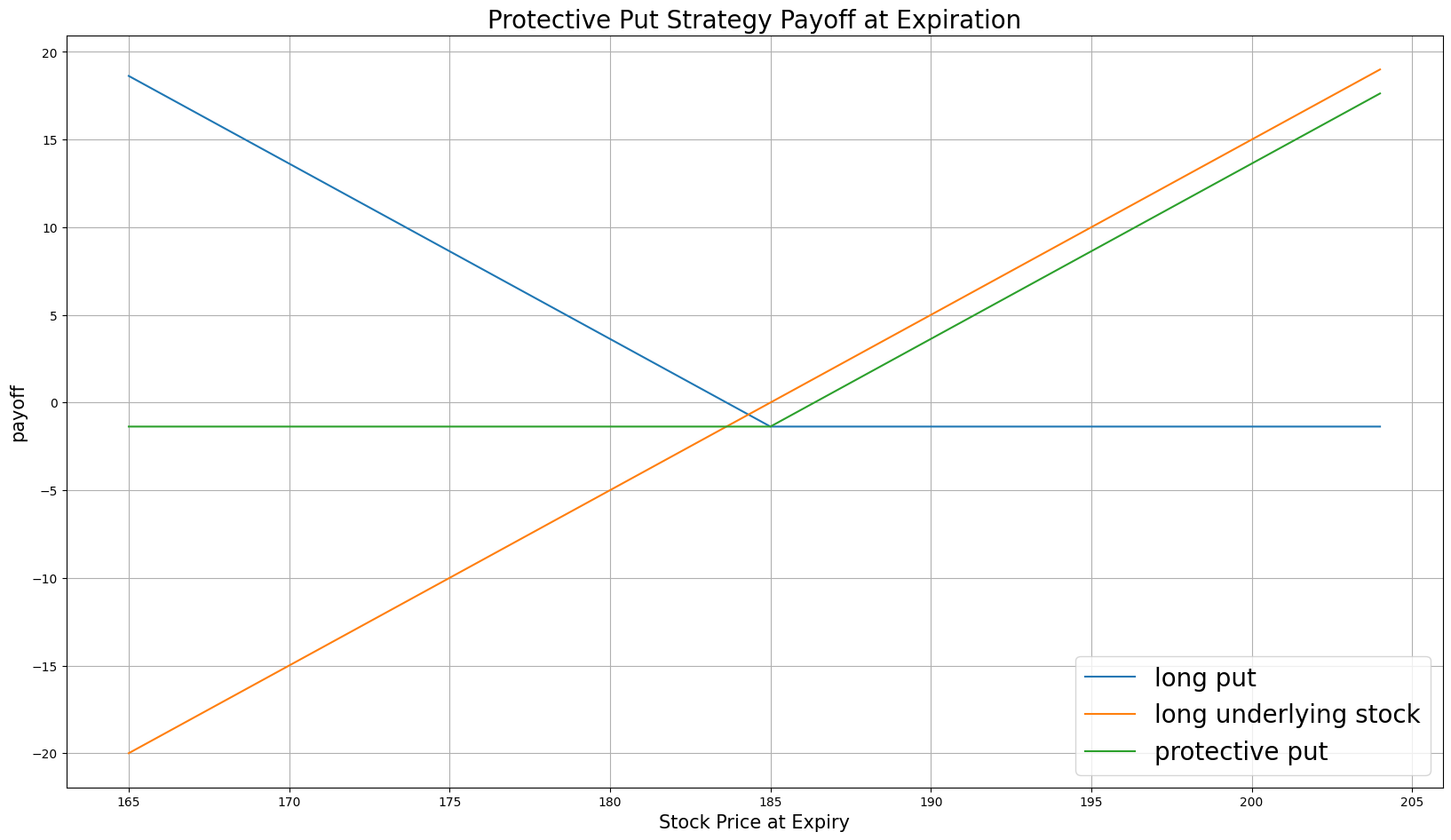
The maximum profit is $S_T - S_0 - P^{K}_0$, which occurs when the underlying price is above the $S_0 + P^{K}_0$.
The maximum loss is $P^{K}_0$, which occurs when the underlying price drops.
Example
The following table shows the price details of the assets in the algorithm:
Asset | Price ($) | Strike ($) |
---|---|---|
Put | 1.53 | 185.00 |
Underlying Equity at start of the trade | 187.07 | - |
Underlying Equity at expiration | 190.01 | - |
Therefore, the payoff is
$$ \begin{array}{rcll} P^{K}_T & = & (K - S_T)^{+}\\ & = & (185 - 190.1)^{+}\\ & = & 0\\ P_T & = & (S_T - S_0 + P^{K}_T - P^{K}_0)\times m - fee\\ & = & (190.01 - 187.07 + 0 - 1.53)\times m - fee\\ & = & 1.41 \times 100 - 2\\ & = & 139 \end{array} $$So, the strategy gains $139.
The following algorithm implements a protective put Option strategy: