Supported Indicators
Correlation
Introduction
The Correlation Indicator is a valuable tool in technical analysis, designed to quantify the degree of relationship between the price movements of a target security (e.g., a stock or ETF) and a reference market index. It measures how closely the target’s price changes are aligned with the fluctuations of the index over a specific period of time, providing insights into the target’s susceptibility to market movements. A positive correlation indicates that the target tends to move in the same direction as the market index, while a negative correlation suggests an inverse relationship. A correlation close to 0 implies a weak or no linear relationship. Commonly, the SPX index is employed as the benchmark for the overall market when calculating correlation, ensuring a consistent and reliable reference point. This helps traders and investors make informed decisions regarding the risk and behavior of the target security in relation to market trends.
To view the implementation of this indicator, see the LEAN GitHub repository.
Using C Indicator
To create an automatic indicators for Correlation
, call the C
helper method from the QCAlgorithm
class. The C
method creates a Correlation
object, hooks it up for automatic updates, and returns it so you can used it in your algorithm. In most cases, you should call the helper method in the Initialize
initialize
method.
public class CorrelationAlgorithm : QCAlgorithm { private Symbol _symbol; private Symbol _reference; private Correlation _c; public override void Initialize() { _symbol = AddEquity("SPY", Resolution.Daily).Symbol; _reference = AddEquity("QQQ", Resolution.Daily).Symbol; _c = C(_symbol, reference, 20, correlationType=CorrelationType.Pearson); } public override void OnData(Slice data) { if (_c.IsReady) { // The current value of _c is represented by itself (_c) // or _c.Current.Value Plot("Correlation", "c", _c); } } }
class CorrelationAlgorithm(QCAlgorithm): def initialize(self) -> None: self._symbol = self.add_equity("SPY", Resolution.DAILY).symbol self.reference = self.add_equity("QQQ", Resolution.DAILY).symbol self._c = self.c(self._symbol, reference, 20, correlationType=CorrelationType.Pearson) def on_data(self, slice: Slice) -> None: if self._c.is_ready: # The current value of self._c is represented by self._c.current.value self.plot("Correlation", "c", self._c.current.value)
The following reference table describes the C
method:
c(target, reference, period, correlation_type=0, resolution=None, selector=None)
[source]Converts a composite FIGI identifier into a String)
- target (Symbol) — The target symbol of this indicator
- reference (Symbol) — The reference symbol of this indicator
- period (int) — The period of this indicator
- correlation_type (CorrelationType, optional) — Correlation type
- resolution (Resolution, optional) — The resolution
- selector (Callable[IBaseData, IBaseDataBar], optional) — Selects a value from the BaseData to send into the indicator, if null defaults to casting the input value to a TradeBar
The Correlation indicator for the given parameters
C(target, reference, period, correlationType=0, resolution=None, selector=None)
[source]Converts a composite FIGI identifier into a String)
- target (Symbol) — The target symbol of this indicator
- reference (Symbol) — The reference symbol of this indicator
- period (Int32) — The period of this indicator
- correlationType (CorrelationType, optional) — Correlation type
- resolution (Resolution, optional) — The resolution
- selector (Func<IBaseData, IBaseDataBar>, optional) — Selects a value from the BaseData to send into the indicator, if null defaults to casting the input value to a TradeBar
The Correlation indicator for the given parameters
If you don't provide a resolution, it defaults to the security resolution. If you provide a resolution, it must be greater than or equal to the resolution of the security. For instance, if you subscribe to hourly data for a security, you should update its indicator with data that spans 1 hour or longer.
For more information about the selector argument, see Alternative Price Fields.
For more information about plotting indicators, see Plotting Indicators.
You can manually create a Correlation
indicator, so it doesn't automatically update. Manual indicators let you update their values with any data you choose.
Updating your indicator manually enables you to control when the indicator is updated and what data you use to update it. To manually update the indicator, call the Update
update
method with a TradeBar
or QuoteBar
. The indicator will only be ready after you prime it with enough data.
public class CorrelationAlgorithm : QCAlgorithm { private Symbol _symbol; private Symbol _reference; private Correlation _c; public override void Initialize() { _symbol = AddEquity("SPY", Resolution.Daily).Symbol; _reference = AddEquity("QQQ", Resolution.Daily).Symbol; _c = new Correlation("", _symbol, reference, 20, correlationType=CorrelationType.Pearson); } public override void OnData(Slice data) { if (data.Bars.TryGetValue(_symbol, out var bar)) { _c.Update(bar); } if (data.Bars.TryGetValue(_reference, out bar)) { _c.Update(bar); } if (_c.IsReady) { // The current value of _c is represented by itself (_c) // or _c.Current.Value Plot("Correlation", "c", _c); } } }
class CorrelationAlgorithm(QCAlgorithm): def initialize(self) -> None: self._symbol = self.add_equity("SPY", Resolution.DAILY).symbol self.reference = self.add_equity("QQQ", Resolution.DAILY).symbol self._c = Correlation("", self._symbol, reference, 20, correlationType=CorrelationType.Pearson) def on_data(self, slice: Slice) -> None: bar = slice.bars.get(self._symbol) if bar: self._c.update(bar) bar = slice.bars.get(self.referece) if bar: self._c.update(bar) if self._c.is_ready: # The current value of self._c is represented by self._c.current.value self.plot("Correlation", "c", self._c.current.value)
To register a manual indicator for automatic updates with the security data, call the RegisterIndicator
register_indicator
method.
public class CorrelationAlgorithm : QCAlgorithm { private Symbol _symbol; private Symbol _reference; private Correlation _c; public override void Initialize() { _symbol = AddEquity("SPY", Resolution.Daily).Symbol; _reference = AddEquity("QQQ", Resolution.Daily).Symbol; _c = new Correlation("", _symbol, reference, 20, correlationType=CorrelationType.Pearson); RegisterIndicator(_symbol, _c, Resolution.Daily); RegisterIndicator(reference, _c, Resolution.Daily); } public override void OnData(Slice data) { if (_c.IsReady) { // The current value of _c is represented by itself (_c) // or _c.Current.Value Plot("Correlation", "c", _c); } } }
class CorrelationAlgorithm(QCAlgorithm): def initialize(self) -> None: self._symbol = self.add_equity("SPY", Resolution.DAILY).symbol self.reference = self.add_equity("QQQ", Resolution.DAILY).symbol self._c = Correlation("", self._symbol, reference, 20, correlationType=CorrelationType.Pearson) self.register_indicator(self._symbol, self._c, Resolution.DAILY) self.register_indicator(reference, self._c, Resolution.DAILY) def on_data(self, slice: Slice) -> None: if self._c.is_ready: # The current value of self._c is represented by self._c.current.value self.plot("Correlation", "c", self._c.current.value)
The following reference table describes the Correlation
constructor:
Correlation
The Correlation Indicator is a valuable tool in technical analysis, designed to quantify the degree of relationship between the price movements of a target security (e.g., a stock or ETF) and a reference market index. It measures how closely the target’s price changes are aligned with the fluctuations of the index over a specific period of time, providing insights into the target’s susceptibility to market movements. A positive correlation indicates that the target tends to move in the same direction as the market index, while a negative correlation suggests an inverse relationship. A correlation close to 0 implies a weak or no linear relationship. Commonly, the SPX index is employed as the benchmark for the overall market when calculating correlation, ensuring a consistent and reliable reference point. This helps traders and investors make informed decisions regarding the risk and behavior of the target security in relation to market trends.
get_enumerator()
Returns an enumerator that iterates through the history window.
IEnumerator[IndicatorDataPoint]
reset()
Resets this indicator to its initial state
to_detailed_string()
Provides a more detailed string of this indicator in the form of {Name} - {Value}
str
update(time, value)
Updates the state of this indicator with the given value and returns true if this indicator is ready, false otherwise
- time (datetime)
- value (float)
bool
update(input)
Updates the state of this indicator with the given value and returns true if this indicator is ready, false otherwise
- input (IBaseData)
bool
consolidators
The data consolidators associated with this indicator if any
The data consolidators associated with this indicator if any
ISet[IDataConsolidator]
current
Gets the current state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
Gets the current state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
IndicatorDataPoint
is_ready
Gets a flag indicating when the indicator is ready and fully initialized
Gets a flag indicating when the indicator is ready and fully initialized
bool
item
Indexes the history windows, where index 0 is the most recent indicator value. If index is greater or equal than the current count, it returns null. If the index is greater or equal than the window size, it returns null and resizes the windows to i + 1.
Indexes the history windows, where index 0 is the most recent indicator value. If index is greater or equal than the current count, it returns null. If the index is greater or equal than the window size, it returns null and resizes the windows to i + 1.
IndicatorDataPoint
name
Gets a name for this indicator
Gets a name for this indicator
str
previous
Gets the previous state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
Gets the previous state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
IndicatorDataPoint
samples
Gets the number of samples processed by this indicator
Gets the number of samples processed by this indicator
int
warm_up_period
Required period, in data points, for the indicator to be ready and fully initialized.
Required period, in data points, for the indicator to be ready and fully initialized.
int
window
A rolling window keeping a history of the indicator values of a given period
A rolling window keeping a history of the indicator values of a given period
RollingWindow[IndicatorDataPoint]
Correlation
The Correlation Indicator is a valuable tool in technical analysis, designed to quantify the degree of relationship between the price movements of a target security (e.g., a stock or ETF) and a reference market index. It measures how closely the target’s price changes are aligned with the fluctuations of the index over a specific period of time, providing insights into the target’s susceptibility to market movements. A positive correlation indicates that the target tends to move in the same direction as the market index, while a negative correlation suggests an inverse relationship. A correlation close to 0 implies a weak or no linear relationship. Commonly, the SPX index is employed as the benchmark for the overall market when calculating correlation, ensuring a consistent and reliable reference point. This helps traders and investors make informed decisions regarding the risk and behavior of the target security in relation to market trends.
GetEnumerator()
Returns an enumerator that iterates through the history window.
IEnumerator[IndicatorDataPoint]
Reset()
Resets this indicator to its initial state
ToDetailedString()
Provides a more detailed string of this indicator in the form of {Name} - {Value}
String
Update(time, value)
Updates the state of this indicator with the given value and returns true if this indicator is ready, false otherwise
- time (DateTime)
- value (decimal)
Boolean
Update(input)
Updates the state of this indicator with the given value and returns true if this indicator is ready, false otherwise
- input (IBaseData)
Boolean
Consolidators
The data consolidators associated with this indicator if any
The data consolidators associated with this indicator if any
ISet<IDataConsolidator>
Current
Gets the current state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
Gets the current state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
IndicatorDataPoint
IsReady
Gets a flag indicating when the indicator is ready and fully initialized
Gets a flag indicating when the indicator is ready and fully initialized
bool
Name
Gets a name for this indicator
Gets a name for this indicator
string
Previous
Gets the previous state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
Gets the previous state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
IndicatorDataPoint
Samples
Gets the number of samples processed by this indicator
Gets the number of samples processed by this indicator
int
WarmUpPeriod
Required period, in data points, for the indicator to be ready and fully initialized.
Required period, in data points, for the indicator to be ready and fully initialized.
Int32
Window
A rolling window keeping a history of the indicator values of a given period
A rolling window keeping a history of the indicator values of a given period
RollingWindow<IndicatorDataPoint>
[System.Int32]
Indexes the history windows, where index 0 is the most recent indicator value. If index is greater or equal than the current count, it returns null. If the index is greater or equal than the window size, it returns null and resizes the windows to i + 1.
Indexes the history windows, where index 0 is the most recent indicator value. If index is greater or equal than the current count, it returns null. If the index is greater or equal than the window size, it returns null and resizes the windows to i + 1.
IndicatorDataPoint
Visualization
The following image shows plot values of selected properties of Correlation
using the plotly library.
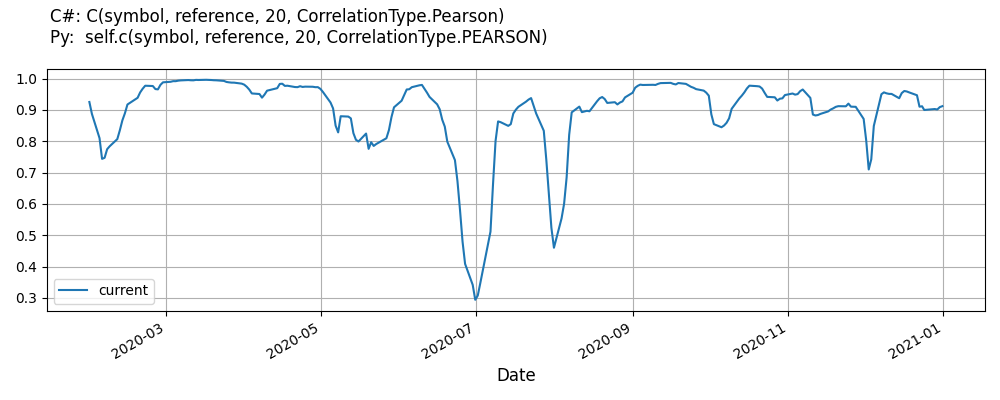
Indicator History
To get the historical data of the Correlation
indicator, call the IndicatorHistory
self.indicator_history
method.
This method resets your indicator, makes a history request, and updates the indicator with the historical data.
Just like with regular history requests, the IndicatorHistory
indicator_history
method supports time periods based on a trailing number of bars, a trailing period of time, or a defined period of time.
If you don't provide a resolution
argument, it defaults to match the resolution of the security subscription.
public class CorrelationAlgorithm : QCAlgorithm { private Symbol _symbol; private Symbol _reference; public override void Initialize() { _symbol = AddEquity("SPY", Resolution.Daily).Symbol; _reference = AddEquity("QQQ", Resolution.Daily).Symbol; var c = C(_symbol, reference, 20, correlationType=CorrelationType.Pearson); var countIndicatorHistory = IndicatorHistory(c, new[] { _symbol, _reference }, 100, Resolution.Minute); var timeSpanIndicatorHistory = IndicatorHistory(c, new[] { _symbol, _reference }, TimeSpan.FromDays(10), Resolution.Minute); var timePeriodIndicatorHistory = IndicatorHistory(c, new[] { _symbol, _reference }, new DateTime(2024, 7, 1), new DateTime(2024, 7, 5), Resolution.Minute); } }
class CorrelationAlgorithm(QCAlgorithm): def initialize(self) -> None: self._symbol = self.add_equity("SPY", Resolution.DAILY).symbol self._reference = self.add_equity("QQQ", Resolution.DAILY).symbol c = self.c(self._symbol, reference, 20, correlationType=CorrelationType.Pearson) count_indicator_history = self.indicator_history(c, [self._symbol, self._reference], 100, Resolution.MINUTE) timedelta_indicator_history = self.indicator_history(c, [self._symbol, self._reference], timedelta(days=10), Resolution.MINUTE) time_period_indicator_history = self.indicator_history(c, [self._symbol, self._reference], datetime(2024, 7, 1), datetime(2024, 7, 5), Resolution.MINUTE)
To make the IndicatorHistory
indicator_history
method update the indicator with an alternative price field instead of the close (or mid-price) of each bar, pass a selector
argument.
var indicatorHistory = IndicatorHistory(c, 100, Resolution.Minute, (bar) => ((TradeBar)bar).High);
indicator_history = self.indicator_history(c, 100, Resolution.MINUTE, lambda bar: bar.high) indicator_history_df = indicator_history.data_frame
If you already have a list of Slice objects, you can pass them to the IndicatorHistory
indicator_history
method to avoid the internal history request.
var history = History(new[] { _symbol, _reference }, 100, Resolution.Minute); var historyIndicatorHistory = IndicatorHistory(c, history);