Option Strategies
Covered Put
Introduction
A Covered Put consists of a short position in a stock and a short position in put Options for the same amount of stock. Covered puts aim to profit from the Option premium by selling puts written on the stock you already shorted. At any time for American Options or at expiration for European Options, if the stock moves below the strike price, you keep the premium and still maintain the underlying Equity position. If the underlying price moves above the strike, the Option buyer can exercise the Options contract, which mean you buy the stock at the strike price but you will still keep the premium. Another risk of a covered put comes from the short stock position, which can drop in value.
Implementation
Follow these steps to implement the covered put strategy:
- In the
Initialize
initialize
method, set the start date, end date, starting cash, and Options universe. - In the
OnData
on_data
method, select the Option contract. - In the
OnData
on_data
method, place the orders.
private Symbol _symbol; public override void Initialize() { SetStartDate(2014, 1, 1); SetEndDate(2014, 3, 1); SetCash(100000); UniverseSettings.Asynchronous = true; var option = AddOption("IBM"); _symbol = option.Symbol; option.SetFilter(universe => universe.IncludeWeeklys().NakedPut(30, 0)); }
def initialize(self) -> None: self.set_start_date(2014, 1, 1) self.set_end_date(2014, 3, 1) self.set_cash(100000) self.universe_settings.asynchronous = True option = self.add_option("IBM") self._symbol = option.symbol option.set_filter(lambda universe: universe.include_weeklys().naked_put(30, 0))
The NakedPut
naked_put
filter narrows the universe down to just the one contract you need to form a covered put.
public override void OnData(Slice slice) { if (Portfolio.Invested || !slice.OptionChains.TryGetValue(_symbol, out var chain)) { return; } // Find ATM put with the farthest expiry var expiry = chain.Max(x => x.Expiry); var atmput = chain .Where(x => x.Right == OptionRight.Put && x.Expiry == expiry) .OrderBy(x => Math.Abs(x.Strike - chain.Underlying.Price)) .FirstOrDefault();
def on_data(self, slice: Slice) -> None: if self.portfolio.invested: return chain = slice.option_chains.get(self._symbol) if not chain: return # Find ATM put with the farthest expiry expiry = max([x.expiry for x in chain]) put_contracts = sorted([x for x in chain if x.right == OptionRight.PUT and x.expiry == expiry], key=lambda x: abs(chain.underlying.price - x.strike)) if not put_contracts: return atm_put = put_contracts[0]
Approach A: Call the OptionStrategies.CoveredPut
OptionStrategies.covered_put
method with the details of each leg and then pass the result to the Buy
buy
method.
var coveredPut = OptionStrategies.CoveredPut(_symbol, atmput.Strike, expiry); Buy(coveredPut, 1);
covered_put = OptionStrategies.covered_put(self._symbol, atm_put.strike, expiry) self.buy(covered_put, 1)
Approach B: Create a list of Leg
objects and then call the Combo Market Ordercombo_market_order, Combo Limit Ordercombo_limit_order, or Combo Leg Limit Ordercombo_leg_limit_order method.
var legs = new List<Leg>() { Leg.Create(atmPut.Symbol, -1), Leg.Create(chain.Underlying.Symbol, -chain.Underlying.SymbolProperties.ContractMultiplier) }; ComboMarketOrder(legs, 1);
legs = [ Leg.create(atm_put.symbol, -1), Leg.create(chain.underlying.symbol, -chain.underlying.symbol_properties.contract_multiplier) ] self.combo_market_order(legs, 1)
Strategy Payoff
The payoff of the strategy is
$$ \begin{array}{rcll} P^{K}_T & = & (K - S_T)^{+}\\ P_T & = & (S_0 - S_T + P^{K}_0 - P^{K}_T)\times m - fee \end{array} $$ $$ \begin{array}{rcll} \textrm{where} & P^{K}_T & = & \textrm{Put value at time T}\\ & S_T & = & \textrm{Underlying asset price at time T}\\ & K & = & \textrm{Put strike price}\\ & P_T & = & \textrm{Payout total at time T}\\ & S_0 & = & \textrm{Underlying asset price when the trade opened}\\ & P^{K}_0 & = & \textrm{Put price when the trade opened (credit received)}\\ & m & = & \textrm{Contract multiplier}\\ & T & = & \textrm{Time of expiration} \end{array} $$The following chart shows the payoff at expiration:
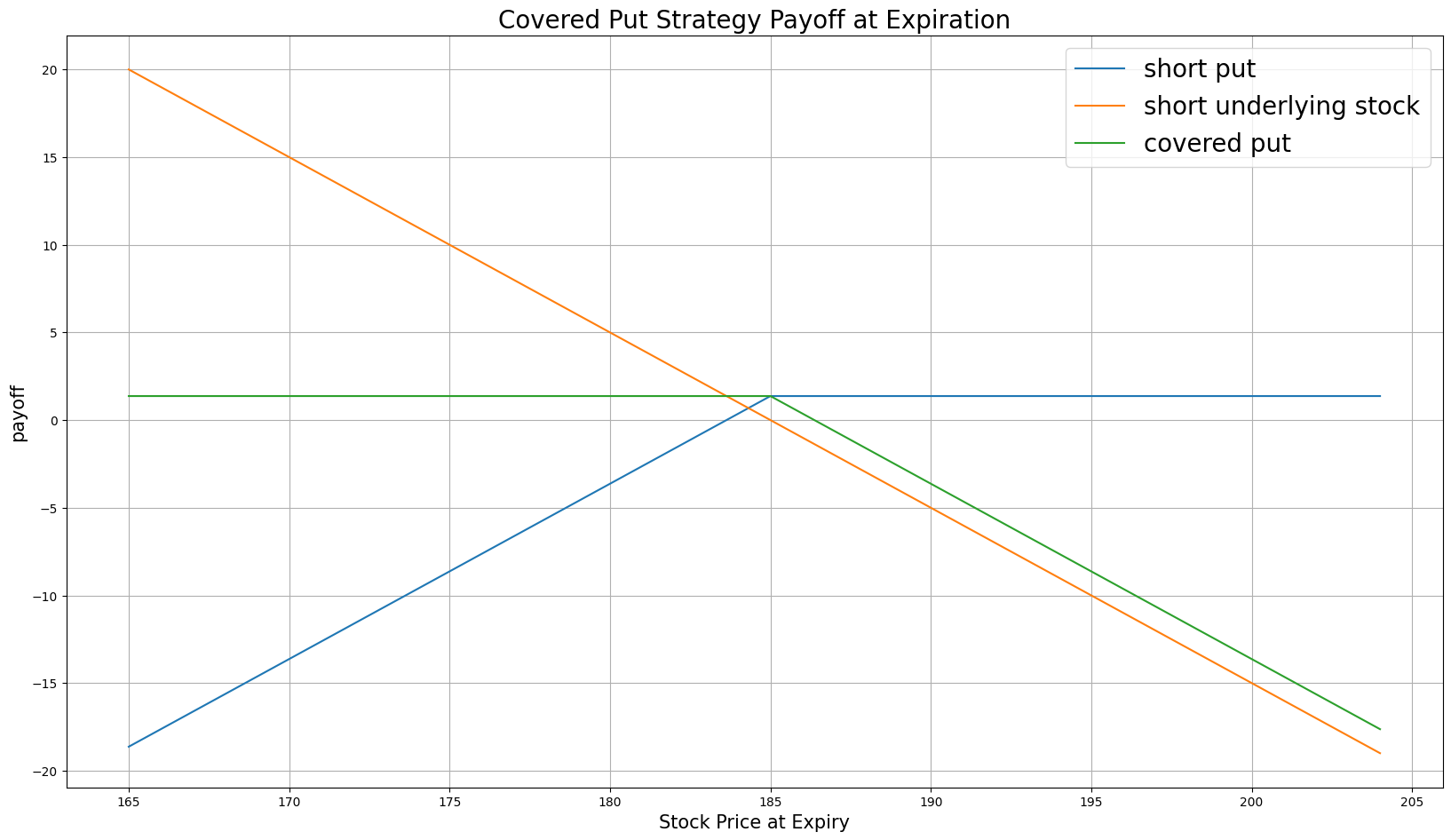
The maximum profit is $S_T - K + P^{K}_0$. It occurs when the underlying price is at or below the strike price of the put at expiration.
If the underlying price increase, the maximum loss is unlimited.
If the Option is American Option, there is a risk of early assignment on the contract you sell.
Example
The following table shows the price details of the assets in the algorithm:
Asset | Price ($) | Strike ($) |
---|---|---|
Put | 1.37 | 185.00 |
Underlying Equity at start of the trade | 186.94 | - |
Underlying Equity at expiration | 190.01 | - |
Therefore, the payoff is
$$ \begin{array}{rcll} P^{K}_T & = & (K - S_T)^{+}\\ & = & (185 - 190.01)^{+}\\ & = & 0\\ P_T & = & (S_0 - S_T + P^{K}_0 - P^{K}_T)\times m - fee\\ & = & (186.94 - 190.01 + 1.37 - 0)\times m - fee\\ & = & -1.70 \times 100 - 2\\ & = & -172 \end{array} $$So, the strategy loses $172.
The following algorithm implements a covered put strategy: