Option Strategies
Bull Put Spread
Introduction
Bull put spread, also known as long put spread, consists of buying an OTM put and selling an ITM put. Both puts have the same underlying Equity and the same expiration date. The OTM put serves as a hedge for the ITM put. The bull put spread profits from a rise in underlying asset price.
Implementation
Follow these steps to implement the bull put spread strategy:
- In the
Initialize
initialize
method, set the start date, end date, cash, and Option universe. - In the
OnData
on_data
method, select the expiration and strikes of the contracts in the strategy legs. - In the
OnData
on_data
method, select the contracts and place the orders.
private Symbol _symbol; public override void Initialize() { SetStartDate(2017, 2, 1); SetEndDate(2017, 3, 5); SetCash(500000); UniverseSettings.Asynchronous = true; var option = AddOption("GOOG", Resolution.Minute); _symbol = option.Symbol; option.SetFilter(universe => universe.IncludeWeeklys().PutSpread(30, 5)); }
def initialize(self) -> None: self.set_start_date(2017, 2, 1) self.set_end_date(2017, 3, 5) self.set_cash(500000) self.universe_settings.asynchronous = True option = self.add_option("GOOG", Resolution.MINUTE) self._symbol = option.symbol option.set_filter(lambda universe: universe.include_weeklys().put_spread(30, 5))
The PutSpread
put_spread
filter narrows the universe down to just the two contracts you need to form a bull put spread.
public override void OnData(Slice slice) { if (Portfolio.Invested || !slice.OptionChains.TryGetValue(_symbol, out var chain)) { return; } // Select the put Option contracts with the furthest expiry var expiry = chain.Max(x => x.Expiry); var puts = chain.Where(x => x.Expiry == expiry && x.Right == OptionRight.Put); if (puts.Count() == 0) return; // Select the ITM and OTM contract strike prices from the remaining contracts var strikes = puts.Select(x => x.Strike).ToList(); var otmStrike = strikes.Min(); var itmStrike = strikes.Max();
def on_data(self, slice: Slice) -> None: if self.portfolio.invested: return # Get the OptionChain chain = slice.option_chains.get(self._symbol, None) if not chain: return # Select the put Option contracts with the furthest expiry expiry = max([x.expiry for x in chain]) puts = [i for i in chain if i.expiry == expiry and i.right == OptionRight.PUT] if not puts == 0: return # Select the ITM and OTM contract strike prices from the remaining contracts strikes = [x.strike for x in puts] otm_strike = min(strikes) itm_strike = max(strikes)
Approach A: Call the OptionStrategies.BullPutSpread
OptionStrategies.bull_put_spread
method with the details of each leg and then pass the result to the Buy
buy
method.
var optionStrategy = OptionStrategies.BullPutSpread(_symbol, itmStrike, otmStrike, expiry); Buy(optionStrategy, 1);
option_strategy = OptionStrategies.bull_put_spread(self._symbol, itm_strike, otm_strike, expiry) self.buy(option_strategy, 1)
Approach B: Create a list of Leg
objects and then call the Combo Market Ordercombo_market_order, Combo Limit Ordercombo_limit_order, or Combo Leg Limit Ordercombo_leg_limit_order method.
var itmPut = puts.Single(x => x.Strike == itmStrike); var otmPut = puts.Single(x => x.Strike == otmStrike); var legs = new List<Leg>() { Leg.Create(itmPut.Symbol, -1), Leg.Create(otmPut.Symbol, 1) }; ComboMarketOrder(legs, 1);
itm_put = [x for x in puts if x.strike == itm_strike][0] otm_put = [x for x in puts if x.strike == otm_strike][0] legs = [ Leg.create(itm_put.symbol, -1), Leg.create(otm_put.symbol, 1) ] self.combo_market_order(legs, 1)
Strategy Payoff
This is a limited-reward-limited-risk strategy. The payoff is
$$ \begin{array}{rcll} P^{OTM}_T & = & (K^{OTM} - S_T)^{+}\\ P^{ITM}_T & = & (K^{ITM} - S_T)^{+}\\ P_T & = & (P^{OTM}_T - P^{ITM}_T + P^{ITM}_0 - P^{OTM}_0)\times m - fee\\ \end{array} $$ $$ \begin{array}{rcll} \textrm{where} & P^{OTM}_T & = & \textrm{OTM put value at time T}\\ & P^{ITM}_T & = & \textrm{ITM put value at time T}\\ & S_T & = & \textrm{Underlying asset price at time T}\\ & K^{OTM} & = & \textrm{OTM put strike price}\\ & K^{ITM} & = & \textrm{ITM put strike price}\\ & P_T & = & \textrm{Payout total at time T}\\ & P^{ITM}_0 & = & \textrm{ITM put value at position opening (credit received)}\\ & P^{OTM}_0 & = & \textrm{OTM put value at position opening (debit paid)}\\ & m & = & \textrm{Contract multiplier}\\ & T & = & \textrm{Time of expiration} \end{array} $$The following chart shows the payoff at expiration:
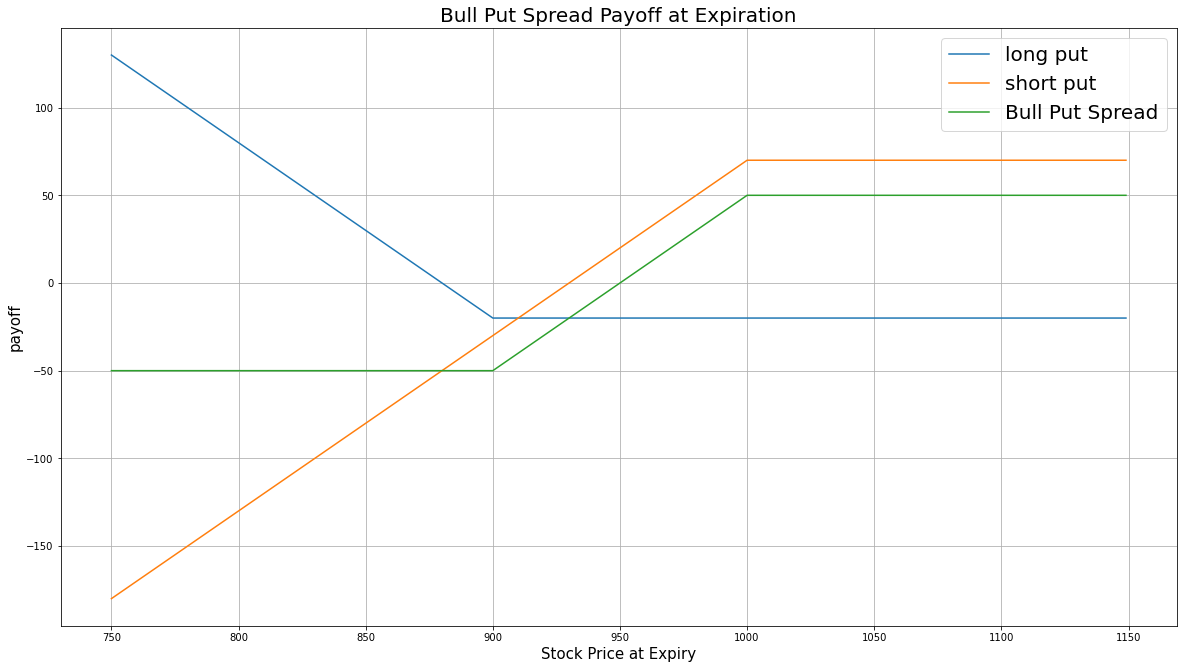
The maximum profit is the net credit you received when opening the position, $P^{ITM}_0 - P^{OTM}_0$. If the underlying price is higher than the strike prices of both put contracts at expiration, both puts expire worthless.
The maximum loss is $K^{ITM} - K^{OTM} + P^{ITM}_0 - P^{OTM}_0$.
If the Option is American Option, there is a risk of early assignment on the contract you sell.
Example
The following table shows the price details of the assets in the algorithm:
Asset | Price ($) | Strike ($) |
---|---|---|
OTM put | 5.70 | 767.50 |
ITM put | 35.50 | 835.00 |
Underlying Equity at expiration | 829.08 | - |
Therefore, the payoff is
$$ \begin{array}{rcll} P^{OTM}_T & = & (K^{OTM} - S_T)^{+}\\ & = & (767.50-829.08)^{+}\\ & = & 0\\ P^{ITM}_T & = & (K^{ITM} - S_T)^{+}\\ & = & (835.00-829.08)^{+}\\ & = & 5.92\\ P_T & = & (P^{OTM}_T - P^{ITM}_T + P^{ITM}_0 - P^{OTM}_0)\times m - fee\\ & = & (0-5.92+35.50-5.70)\times100-1.00\times2\\ & = & 2386\\ \end{array} $$So, the strategy profits $2,386.
The following algorithm implements a bull put spread strategy: