Option Strategies
Long Straddle
Introduction
Long Straddle is an Options trading strategy that consists of buying an ATM call and an ATM put, where both contracts have the same underlying asset, strike price, and expiration date. This strategy aims to profit from volatile movements in the underlying stock, either positive or negative.
Implementation
Follow these steps to implement the long straddle strategy:
- In the
Initialize
initialize
method, set the start date, end date, cash, and Option universe. - In the
OnData
on_data
method, select the expiration date and strike price of the contracts in the strategy legs. - In the
OnData
on_data
method, call theOptionStrategies.Straddle
method and then submit the order.
private Symbol _symbol; public override void Initialize() { SetStartDate(2017, 4, 1); SetEndDate(2017, 6, 30); SetCash(100000); UniverseSettings.Asynchronous = true; var option = AddOption("GOOG"); _symbol = option.Symbol; option.SetFilter(-1, 1, 30, 60); }
def initialize(self) -> None: self.set_start_date(2017, 4, 1) self.set_end_date(2017, 6, 30) self.set_cash(100000) self.universe_settings.asynchronous = True option = self.add_option("GOOG") self._symbol = option.symbol option.set_filter(-1, 1, 30, 60)
public override void OnData(Slice slice) { if (Portfolio.Invested || !slice.OptionChains.TryGetValue(_symbol, out var chain)) { return; } // Find ATM options with the nearest expiry var expiry = chain.Min(contract => contract.Expiry); var contracts = chain.Where(contract => contract.Expiry == expiry) .OrderBy(contract => Math.Abs(contract.Strike - chain.Underlying.Price)) .ToArray(); if (contracts.Length < 2) return;
def on_data(self, slice: Slice) -> None: if self.Portfolio.Invested: return chain = slice.OptionChains.get(self._symbol, None) if not chain: return # Find ATM options with the nearest expiry expiry = min([x.Expiry for x in chain]) contracts = sorted([x for x in chain if x.Expiry == expiry], key=lambda x: abs(chain.Underlying.Price - x.Strike)) if len(contracts) < 2: return
var longStraddle = OptionStrategies.Straddle(_symbol, contracts[0].Strike, expiry); Buy(longStraddle, 1);
long_straddle = OptionStrategies.straddle(self._symbol, contracts[0].strike, expiry) self.buy(long_straddle, 1)
Option strategies synchronously execute by default. To asynchronously execute Option strategies, set the asynchronous
argument to False
false
. You can also provide a tag and order properties to the
Buy
method.
Buy(optionStrategy, quantity, asynchronous, tag, orderProperties);
self.Buy(option_strategy, quantity, asynchronous, tag, order_properties)
Strategy Payoff
The payoff of the strategy is
$$ \begin{array}{rcll} C^{ATM}_T & = & (S_T - K^{C})^{+}\\ P^{ATM}_T & = & (K^{P} - S_T)^{+}\\ P_T & = & (C^{ATM}_T + P^{ATM}_T - C^{ATM}_0 - P^{ATM}_0)\times m - fee \end{array} $$ $$ \begin{array}{rcll} \textrm{where} & C^{ATM}_T & = & \textrm{ATM call value at time T}\\ & P^{ATM}_T & = & \textrm{ATM put value at time T}\\ & S_T & = & \textrm{Underlying asset price at time T}\\ & K^{C} & = & \textrm{ATM call strike price}\\ & K^{P} & = & \textrm{ATM put strike price}\\ & P_T & = & \textrm{Payout total at time T}\\ & C^{ATM}_0 & = & \textrm{ATM call value at position opening (debit paid)}\\ & P^{ATM}_0 & = & \textrm{ATM put value at position opening (debit paid)}\\ & m & = & \textrm{Contract multiplier}\\ & T & = & \textrm{Time of expiration} \end{array} $$The following chart shows the payoff at expiration:
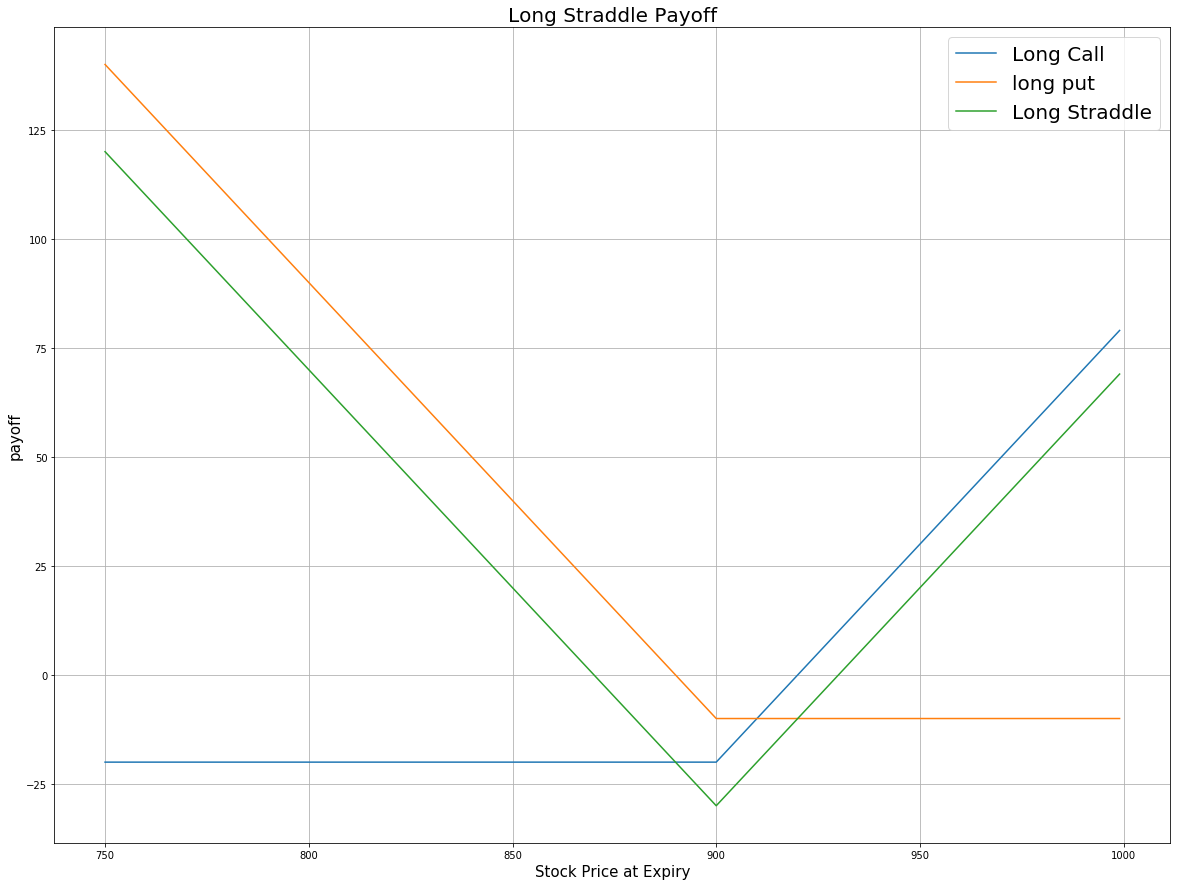
The maximum profit is unlimited if the underlying price rises to infinity or substantial, $K^{P} - C^{OTM}_0 - P^{OTM}_0$, if it drops to zero at expiration.
The maximum loss is the net debit paid, $C^{ATM}_0 + P^{ATM}_0$. It occurs when the underlying price is the same at expiration as it was when you opened the trade. In this case, both Options expire worthless.
Example
The following table shows the price details of the assets in the algorithm at Option expiration (2017-05-20):
Asset | Price ($) | Strike ($) |
---|---|---|
Call | 22.30 | 835.00 |
Put | 23.90 | 835.00 |
Underlying Equity at expiration | 934.01 | - |
Therefore, the payoff is
$$ \begin{array}{rcll} C^{ATM}_T & = & (S_T - K^{C})^{+}\\ & = & (934.01-835.00)^{+}\\ & = & 98.99\\ P^{ATM}_T & = & (K^{P} - S_T)^{+}\\ & = & (835.00-934.01)^{+}\\ & = & 0\\ P_T & = & (C^{ATM}_T + P^{ATM}_T - C^{ATM}_0 - P^{ATM}_0)\times m - fee\\ & = & (98.99+0-22.3-23.9)\times100-1.00\times2\\ & = & 5277 \end{array} $$So, the strategy gains $5,277.
The following algorithm implements a long straddle strategy: