Index
Handling Data
Introduction
LEAN passes the data you request to the OnData
on_data
method so you can make trading decisions. The default OnData
on_data
method accepts a Slice
object, but you can define additional OnData
on_data
methods that accept different data types. For example, if you define an OnData
on_data
method that accepts a Tick
argument, it only receives Tick
objects. The Slice
object that the OnData
on_data
method receives groups all the data together at a single moment in time. To access the Slice
outside of the OnData
on_data
method, use the CurrentSlice
current_slice
property of your algorithm.
All the data formats use DataDictionary
objects to group data by Symbol
and provide easy access to information. The plural of the type denotes the collection of objects. For instance, the Ticks
DataDictionary
is made up of Tick
objects. To access individual data points in the dictionary, you can index the dictionary with the Index ticker or Symbol
symbol
, but we recommend you use the Symbol
symbol
.
To view the resolutions that are available for Index data, see Resolutions.
Bars
You can't trade Indices, but TradeBar
objects are bars that represent the open, high, low, and close of an Index price over a period of time.
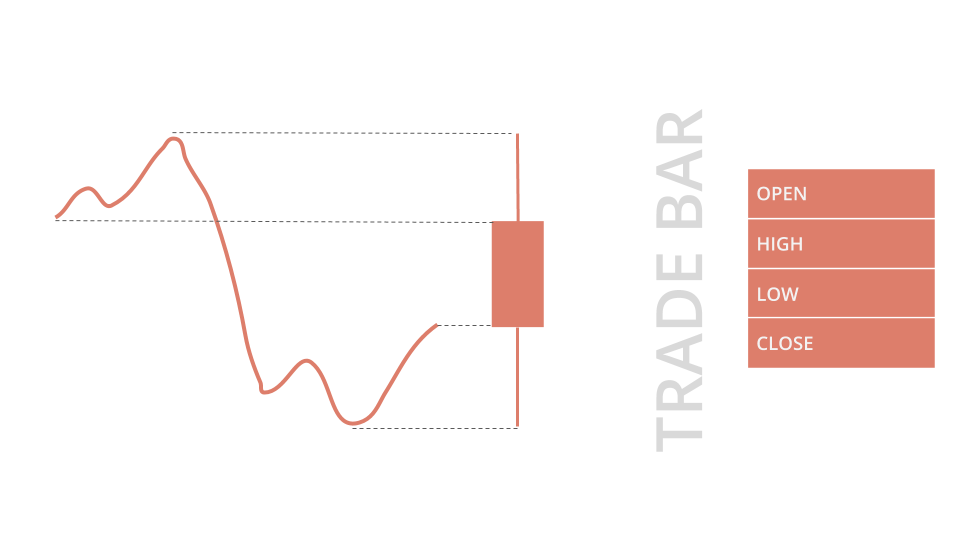
TradeBar
objects have the following properties:
To get the TradeBar
objects in the Slice
, index the Slice
or index the Bars
bars
property of the Slice
with the Index Symbol
. The Slice
may not contain data for your Symbol
at every time step. To avoid issues, check if the Slice
contains data for your Index before you index the Slice
with the Index Symbol
.
public override void OnData(Slice slice) { if (slice.Bars.ContainsKey(_symbol)) { var tradeBar = slice.Bars[_symbol]; var value = tradeBar.Value; } }
def on_data(self, slice: Slice) -> None: if self._symbol in slice.bars: trade_bar = slice.bars[self._symbol] value = trade_bar.value