Option Strategies
Short Strangle
Introduction
Short Strangle is an Options trading strategy that consists of simultaneously selling an OTM put and an OTM call, where both contracts have the same underlying asset and expiration date. By doing so, the trader is essentially betting that the underlying asset will remain relatively stable and not experience significant price movements before the Options' expiration.
Compared to a short straddle, the net debit of a short strangle is lower since OTM Options are cheaper. Additionally, the winning range of a short straddle is wider and the strike spread is wider.
Implementation
Follow these steps to implement the short strangle strategy:
- In the
Initialize
initialize
method, set the start date, end date, cash, and Option universe. - In the
OnData
on_data
method, select the expiration date and strike prices of the contracts in the strategy legs. - In the
OnData
on_data
method, call theOptionStrategies.Strangle
method and then submit the order.
private Symbol _symbol; public override void Initialize() { SetStartDate(2017, 4, 1); SetEndDate(2017, 4, 30); SetCash(100000); UniverseSettings.Asynchronous = true; var option = AddOption("GOOG"); _symbol = option.Symbol; option.SetFilter(-5, 5, 0, 30); }
def initialize(self) -> None: self.set_start_date(2017, 4, 1) self.set_end_date(2017, 4, 30) self.set_cash(100000) self.universe_settings.asynchronous = True option = self.add_option("GOOG") self._symbol = option.symbol option.set_filter(-5, 5, 0, 30)
public override void OnData(Slice slice) { if (Portfolio.Invested || !slice.OptionChains.TryGetValue(_symbol, out var chain)) { return; } // Find options with the farthest expiry var expiry = chain.Max(contract => contract.Expiry); var contracts = chain.Where(contract => contract.Expiry == expiry).ToList(); // Order the OTM calls by strike to find the nearest to ATM var callContracts = contracts .Where(contract => contract.Right == OptionRight.Call && contract.Strike > chain.Underlying.Price) .OrderBy(contract => contract.Strike).ToArray(); if (callContracts.Length == 0) return; // Order the OTM puts by strike to find the nearest to ATM var putContracts = contracts .Where(contract => contract.Right == OptionRight.Put && contract.Strike < chain.Underlying.Price) .OrderByDescending(contract => contract.Strike).ToArray(); if (putContracts.Length == 0) return; var callStrike = callContracts[0].Strike; var putStrike = putContracts[0].Strike; }
def on_data(self, slice: Slice) -> None: if self.Portfolio.Invested: return chain = slice.OptionChains.get(self._symbol) if not chain: return # Find options with the farthest expiry expiry = max([x.Expiry for x in chain]) contracts = [contract for contract in chain if contract.Expiry == expiry] # Order the OTM calls by strike to find the nearest to ATM call_contracts = sorted([contract for contract in contracts if contract.Right == OptionRight.Call and contract.Strike > chain.Underlying.Price], key=lambda x: x.Strike) if not call_contracts: return # Order the OTM puts by strike to find the nearest to ATM put_contracts = sorted([contract for contract in contracts if contract.Right == OptionRight.Put and contract.Strike < chain.Underlying.Price], key=lambda x: x.Strike, reverse=True) if not put_contracts: return call_strike = call_contracts[0].Strike put_strike = put_contracts[0].Strike
var shortStrangle = OptionStrategies.ShortStrangle(_symbol, callStrike, putStrike, expiry); Buy(shortStrangle, 1);
short_strangle = OptionStrategies.short_strangle(self._symbol, call_strike, put_strike, expiry) self.buy(short_strangle, 1)
Option strategies synchronously execute by default. To asynchronously execute Option strategies, set the asynchronous
argument to False
false
. You can also provide a tag and order properties to the
Buy
method.
Buy(optionStrategy, quantity, asynchronous, tag, orderProperties);
self.Buy(option_strategy, quantity, asynchronous, tag, order_properties)
Strategy Payoff
The payoff of the strategy is
$$ \begin{array}{rcll} C^{OTM}_T & = & (S_T - K^{C})^{+}\\ P^{OTM}_T & = & (K^{P} - S_T)^{+}\\ P_T & = & (-C^{OTM}_T - P^{OTM}_T + C^{OTM}_0 + P^{OTM}_0)\times m - fee \end{array} $$ $$ \begin{array}{rcll} \textrm{where} & C^{OTM}_T & = & \textrm{OTM call value at time T}\\ & P^{OTM}_T & = & \textrm{OTM put value at time T}\\ & S_T & = & \textrm{Underlying asset price at time T}\\ & K^{C} & = & \textrm{OTM call strike price}\\ & K^{P} & = & \textrm{OTM put strike price}\\ & P_T & = & \textrm{Payout total at time T}\\ & C^{OTM}_0 & = & \textrm{OTM call value at position opening (debit paid)}\\ & P^{OTM}_0 & = & \textrm{OTM put value at position opening (debit paid)}\\ & m & = & \textrm{Contract multiplier}\\ & T & = & \textrm{Time of expiration} \end{array} $$The following chart shows the payoff at expiration:
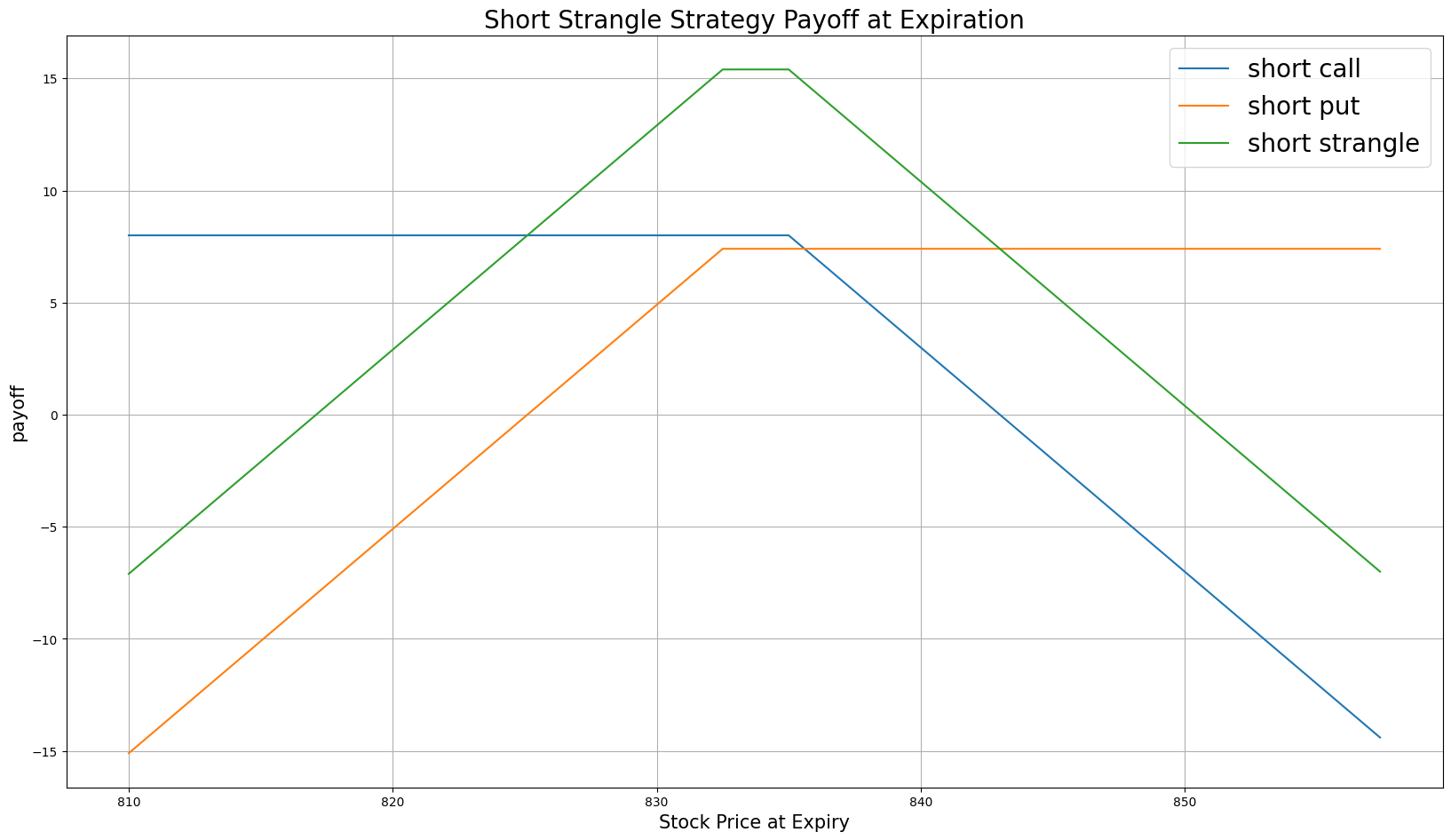
The maximum profit $C^{OTM}_0 + P^{OTM}_0$. It occurs when the underlying price at expiration remains within the range of the strike prices. In this case, both Options expire worthless.
The maximum loss is unlimited if the underlying price rises to infinity or substantial, $C^{OTM}_0 + P^{OTM}_0 - K^{P}$, if it drops to zero at expiration.
Example
The following table shows the price details of the assets in the algorithm at Option expiration (2017-04-22):
Asset | Price ($) | Strike ($) |
---|---|---|
Call | 8.00 | 835.00 |
Put | 7.40 | 832.50 |
Underlying Equity at expiration | 843.19 | - |
Therefore, the payoff is
$$ \begin{array}{rcll} C^{OTM}_T & = & (S_T - K^{C})^{+}\\ & = & (843.19-835.00)^{+}\\ & = & 8.19\\ P^{OTM}_T & = & (K^{P} - S_T)^{+}\\ & = & (832.50-843.19)^{+}\\ & = & 0\\ P_T & = & (-C^{OTM}_T - P^{OTM}_T + C^{OTM}_0 + P^{OTM}_0)\times m - fee\\ & = & (-8.19-0+8.00+7.40)\times100-2.00\times2\\ & = & 719 \end{array} $$So, the strategy gains $719.
The following algorithm implements a short straddle strategy: