Futures
Handling Data
Introduction
LEAN passes the data you request to the OnData
on_data
method so you can make trading decisions. The default OnData
on_data
method accepts a Slice
object, but you can define additional OnData
on_data
methods that accept different data types. For example, if you define an OnData
on_data
method that accepts a TradeBar
argument, it only receives TradeBar
objects. The Slice
object that the OnData
on_data
method receives groups all the data together at a single moment in time. To access the Slice
outside of the OnData
on_data
method, use the CurrentSlice
current_slice
property of your algorithm.
All the data formats use DataDictionary
objects to group data by Symbol
and provide easy access to information. The plural of the type denotes the collection of objects. For instance, the TradeBars
DataDictionary
is made up of TradeBar
objects. To access individual data points in the dictionary, you can index the dictionary with the contract ticker or Symbol
symbol
, but we recommend you use the Symbol
symbol
.
To view the resolutions that are available for Futures data, see Resolutions.
Trades
TradeBar
objects are price bars that consolidate individual trades from the exchanges. They contain the open, high, low, close, and volume of trading activity over a period of time.
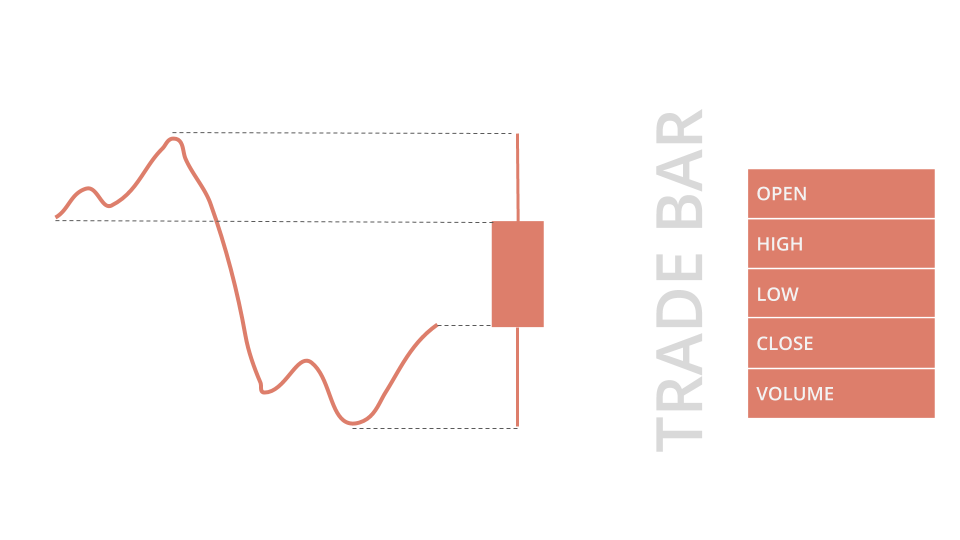
TradeBar
objects have the following properties:
To get the TradeBar
objects in the Slice
, index the Slice
or index the Bars
bars
property of the Slice
with the contract Symbol
symbol
. If the contract doesn't actively trade or you are in the same time step as when you added the contract subscription, the Slice
may not contain data for your Symbol
symbol
. To avoid issues, check if the Slice
contains data for your contract before you index the Slice
with the contract Symbol
symbol
.
public override void OnData(Slice slice) { if (slice.Bars.ContainsKey(_contractSymbol)) { var tradeBar = slice.Bars[_contractSymbol]; } }
def on_data(self, slice: Slice) -> None: trade_bar = slice.bars.get(self._contract_symbol) # None if not found
You can also iterate through the TradeBars
dictionary. The keys of the dictionary are the Symbol
objects and the values are the TradeBar
objects.
public override void OnData(Slice slice) { foreach (var kvp in slice.Bars) { var symbol = kvp.Key; var tradeBar = kvp.Value; var closePrice = tradeBar.Close; } }
def on_data(self, slice: Slice) -> None: for symbol, trade_bar in slice.bars.items(): close_price = trade_bar.close
Quotes
QuoteBar
objects are bars that consolidate NBBO quotes from the exchanges. They contain the open, high, low, and close prices of the bid and ask. The Open
open
, High
high
, Low
low
, and Close
close
properties of the QuoteBar
object are the mean of the respective bid and ask prices. If the bid or ask portion of the QuoteBar
has no data, the Open
open
, High
high
, Low
low
, and Close
close
properties of the QuoteBar
copy the values of either the Bid
bid
or Ask
ask
instead of taking their mean.
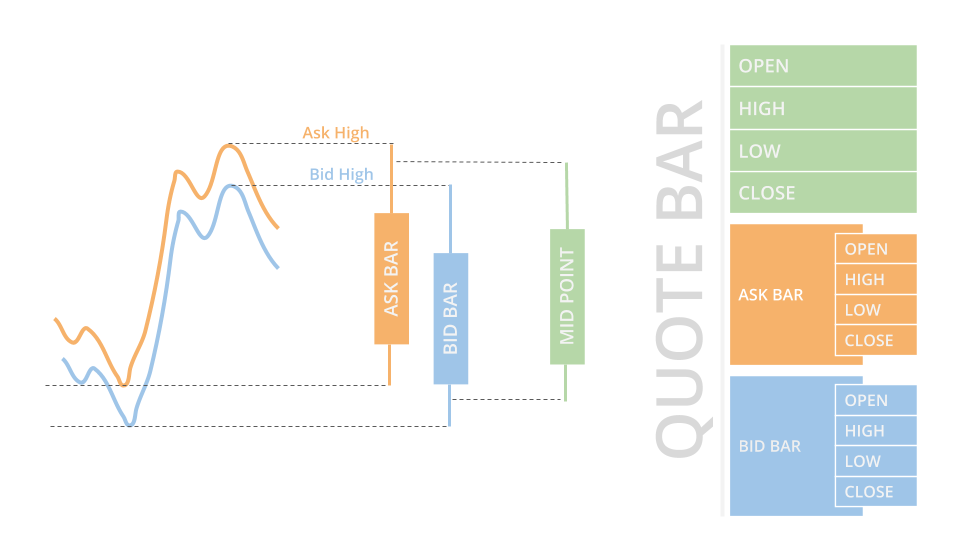
QuoteBar
objects have the following properties:
To get the QuoteBar
objects in the Slice
, index the QuoteBars
property of the Slice
with the contract Symbol
symbol
. If the contract doesn't actively get quotes or you are in the same time step as when you added the contract subscription, the Slice
may not contain data for your Symbol
symbol
. To avoid issues, check if the Slice
contains data for your contract before you index the Slice
with the contract Symbol
symbol
.
public override void OnData(Slice slice) { if (slice.QuoteBars.ContainsKey(_contractSymbol)) { var quoteBar = slice.QuoteBars[_contractSymbol]; } }
def on_data(self, slice: Slice) -> None: quote_bar = slice.quote_bars.get(self._contract_symbol) # None if not found
You can also iterate through the QuoteBars
dictionary. The keys of the dictionary are the Symbol
objects and the values are the QuoteBar
objects.
public override void OnData(Slice slice) { foreach (var kvp in slice.QuoteBars) { var symbol = kvp.Key; var quoteBar = kvp.Value; var askPrice = quoteBar.Ask.Close; } }
def on_data(self, slice: Slice) -> None: for symbol, quote_bar in slice.quote_bars.items(): ask_price = quote_bar.ask.close
QuoteBar
objects let LEAN incorporate spread costs into your simulated trade fills to make backtest results more realistic.
Ticks
Tick
objects represent a single trade or quote at a moment in time. A trade tick is a record of a transaction for the contract. A quote tick is an offer to buy or sell the contract at a specific price. Tick
objects have the following properties:
Trade ticks have a non-zero value for the Quantity
quantity
and Price
price
properties, but they have a zero value for the BidPrice
bid_price
, BidSize
bid_size
, AskPrice
ask_price
, and AskSize
ask_size
properties. Quote ticks have non-zero values for BidPrice
bid_price
and BidSize
bid_size
properties or have non-zero values for AskPrice
ask_price
and AskSize
ask_size
properties. To check if a tick is a trade or a quote, use the TickType
ticktype
property.
In backtests, LEAN groups ticks into one millisecond buckets. In live trading, LEAN groups ticks into ~70-millisecond buckets. To get the Tick
objects in the Slice
, index the Ticks
property of the Slice
with a Symbol
symbol
. If the contract doesn't actively trade or you are in the same time step as when you added the contract subscription, the Slice
may not contain data for your Symbol
symbol
. To avoid issues, check if the Slice
contains data for your contract before you index the Slice
with the contract Symbol
symbol
.
public override void OnData(Slice slice) { if (slice.Ticks.ContainsKey(_contractSymbol)) { var ticks = slice.Ticks[_contractSymbol]; foreach (var tick in ticks) { var price = tick.Price; } } }
def on_data(self, slice: Slice) -> None: ticks = slice.ticks.get(self._contract_symbol, []) # Empty if not found for tick in ticks: price = tick.price
You can also iterate through the Ticks
dictionary. The keys of the dictionary are the Symbol
objects and the values are the List<Tick>
list[Tick]
objects.
public override void OnData(Slice slice) { foreach (var kvp in slice.Ticks) { var symbol = kvp.Key; var ticks = kvp.Value; foreach (var tick in ticks) { var price = tick.Price; } } }
def on_data(self, slice: Slice) -> None: for symbol, ticks in slice.ticks.items(): for tick in ticks: price = tick.price
Tick data is raw and unfiltered, so it can contain bad ticks that skew your trade results. For example, some ticks come from dark pools, which aren't tradable. We recommend you only use tick data if you understand the risks and are able to perform your own online tick filtering.
Futures Chains
FuturesChain
objects represent an entire chain of contracts for a single underlying Future. They have the following properties:
To get the FuturesChain
, index the FuturesChains
futures_chains
property of the Slice
with the continuous contract Symbol
.
public override void OnData(Slice slice) { if (slice.FuturesChains.TryGetValue(_contractSymbol.Canonical, out var chain)) { var contracts = chain.Contracts; } }
def on_data(self, slice: Slice) -> None: chain = slice.futures_chains.get(self._contract_symbol.canonical) if chain: contracts = chain.contracts
You can also loop through the FuturesChains
futures_chains
property to get each FuturesChain
.
public override void OnData(Slice slice) { foreach (var kvp in slice.FuturesChains) { var continuousContractSymbol = kvp.Key; var chain = kvp.Value; var contracts = chain.Contracts; } } public void OnData(FuturesChains futuresChains) { foreach (var kvp in futuresChains) { var continuousContractSymbol = kvp.Key; var chain = kvp.Value; var contracts = chain.Contracts; } }
def on_data(self, slice: Slice) -> None: for continuous_contract_symbol, chain in slice.futures_chains.items(): contracts = chain.contracts
Futures Contracts
FuturesContract
objects represent the data of a single Futures contract in the market. They have the following properties:
To get the Futures contracts in the Slice
, use the Contracts
contracts
property of the FuturesChain
.
public override void OnData(Slice slice) { if (slice.FuturesChains.TryGetValue(_contractSymbol.Canonical, out var chain)) { if (chain.Contracts.TryGetValue(_contractSymbol, out var contract)) { var price = contract.LastPrice; } } } public void OnData(FuturesChains futuresChains) { if (futuresChains.TryGetValue(_contractSymbol.Canonical, out var chain)) { if (chain.Contracts.TryGetValue(_contractSymbol, out var contract)) { var price = contract.LastPrice; } } }
def on_data(self, slice: Slice) -> None: chain = slice.FuturesChains.get(self._contract_symbol.Canonical) if chain: contract = chain.Contracts.get(self._contract_symbol) if contract: price = contract.LastPrice
Open interest is the number of outstanding contracts that haven't been settled. It provides a measure of investor interest and the market liquidity, so it's a popular metric to use for contract selection. Open interest is calculated once per day. To get the latest open interest value, use the OpenInterest
open_interest
property of the Future
or FutureContract
Futurecontract
.
public override void OnData(Slice slice) { if (slice.FuturesChains.TryGetValue(_contractSymbol.Canonical, out var chain)) { if (chain.Contracts.TryGetValue(_contractSymbol, out var contract)) { var openInterest = contract.OpenInterest; } } }
def on_data(self, slice: Slice) -> None: chain = slice.FuturesChains.get(self._contract_symbol.canonical) if chain: contract = chain.contracts.get(self._contract_symbol) if contract: open_interest = contract.open_interest
Symbol Changes
When the continuous contract rolls over, LEAN passes a SymbolChangedEvent
to your OnData
on_data
method, which contains the old contract Symbol
and the new contract Symbol
. SymbolChangedEvent
objects have the following properties:
To get the SymbolChangedEvent
, use the SymbolChangedEvents
symbol_changed_events
property of the Slice
. You can use the SymbolChangedEvent
to roll over contracts.
public override void OnData(Slice slice) { foreach (var (symbol, changedEvent) in slice.SymbolChangedEvents) { var oldSymbol = changedEvent.OldSymbol; var newSymbol = changedEvent.NewSymbol; var tag = $"Rollover - Symbol changed at {Time}: {oldSymbol} -> {newSymbol}"; var quantity = Portfolio[oldSymbol].Quantity; // Rolling over: to liquidate any position of the old mapped contract and switch to the newly mapped contract Liquidate(oldSymbol, tag: tag); if (quantity != 0) MarketOrder(newSymbol, quantity, tag: tag); Log(tag); } }
def on_data(self, slice: Slice) -> None: for symbol, changed_event in slice.symbol_changed_events.items(): old_symbol = changed_event.old_symbol new_symbol = changed_event.new_symbol tag = f"Rollover - Symbol changed at {self.time}: {old_symbol} -> {new_symbol}" quantity = self.portfolio[old_symbol].quantity # Rolling over: to liquidate any position of the old mapped contract and switch to the newly mapped contract self.liquidate(old_symbol, tag=tag) if quantity: self.market_order(new_symbol, quantity, tag=tag) self.log(tag)
In backtesting, the SymbolChangedEvent
occurs at midnight Eastern Time (ET). In live trading, the live data for continuous contract mapping arrives at 6/7 AM ET, so that's when it occurs.