Supported Indicators
Exponential Moving Average
Introduction
This indicator represents the traditional exponential moving average indicator (EMA). When the indicator is ready, the first value of the EMA is equivalent to the simple moving average. After the first EMA value, the EMA value is a function of the previous EMA value. Therefore, depending on the number of samples you feed into the indicator, it can provide different EMA values for a single security and lookback period. To make the indicator values consistent across time, warm up the indicator with all the trailing security price history.
To view the implementation of this indicator, see the LEAN GitHub repository.
Using EMA Indicator
To create an automatic indicators for ExponentialMovingAverage
, call the EMA
helper method from the QCAlgorithm
class. The EMA
method creates a ExponentialMovingAverage
object, hooks it up for automatic updates, and returns it so you can used it in your algorithm. In most cases, you should call the helper method in the Initialize
initialize
method.
public class ExponentialMovingAverageAlgorithm : QCAlgorithm { private Symbol _symbol; private ExponentialMovingAverage _ema; public override void Initialize() { _symbol = AddEquity("SPY", Resolution.Daily).Symbol; _ema = EMA(_symbol, 20, 0.5); } public override void OnData(Slice data) { if (_ema.IsReady) { // The current value of _ema is represented by itself (_ema) // or _ema.Current.Value Plot("ExponentialMovingAverage", "ema", _ema); } } }
class ExponentialMovingAverageAlgorithm(QCAlgorithm): def initialize(self) -> None: self._symbol = self.add_equity("SPY", Resolution.DAILY).symbol self.ema = self.EMA(self.symbol, 20, 0.5) def on_data(self, slice: Slice) -> None: if self.ema.is_ready: # The current value of self.ema is represented by self.ema.current.value self.plot("ExponentialMovingAverage", "ema", self.ema.current.value)
The following reference table describes the EMA
method:
ema(symbol, period, smoothing_factor, resolution=None, selector=None)
[source]Creates an ExponentialMovingAverage indicator for the symbol. The indicator will be automatically updated on the given resolution.
- symbol (Symbol) — The symbol whose EMA we want
- period (int) — The period of the EMA
- smoothing_factor (float) — The percentage of data from the previous value to be carried into the next value
- resolution (Resolution, optional) — The resolution
- selector (Callable[IBaseData, float], optional) — x.Value)
The ExponentialMovingAverage for the given parameters
EMA(symbol, period, smoothingFactor, resolution=None, selector=None)
[source]Creates an ExponentialMovingAverage indicator for the symbol. The indicator will be automatically updated on the given resolution.
- symbol (Symbol) — The symbol whose EMA we want
- period (Int32) — The period of the EMA
- smoothingFactor (decimal) — The percentage of data from the previous value to be carried into the next value
- resolution (Resolution, optional) — The resolution
- selector (Func<IBaseData, Decimal>, optional) — x.Value)
The ExponentialMovingAverage for the given parameters
If you don't provide a resolution, it defaults to the security resolution. If you provide a resolution, it must be greater than or equal to the resolution of the security. For instance, if you subscribe to hourly data for a security, you should update its indicator with data that spans 1 hour or longer.
For more information about the selector argument, see Alternative Price Fields.
For more information about plotting indicators, see Plotting Indicators.
You can manually create a ExponentialMovingAverage
indicator, so it doesn't automatically update. Manual indicators let you update their values with any data you choose.
Updating your indicator manually enables you to control when the indicator is updated and what data you use to update it. To manually update the indicator, call the Update
update
method with time/number pair or an IndicatorDataPoint
. The indicator will only be ready after you prime it with enough data.
public class ExponentialMovingAverageAlgorithm : QCAlgorithm { private Symbol _symbol; private ExponentialMovingAverage _ema; public override void Initialize() { _symbol = AddEquity("SPY", Resolution.Daily).Symbol; _ema = new ExponentialMovingAverage(20, 0.5); } public override void OnData(Slice data) { if (data.Bars.TryGetValue(_symbol, out var bar)) { _ema.Update(bar.EndTime, bar.Close); } if (_ema.IsReady) { // The current value of _ema is represented by itself (_ema) // or _ema.Current.Value Plot("ExponentialMovingAverage", "ema", _ema); } } }
class ExponentialMovingAverageAlgorithm(QCAlgorithm): def initialize(self) -> None: self._symbol = self.add_equity("SPY", Resolution.DAILY).symbol self.ema = ExponentialMovingAverage(20, 0.5) def on_data(self, slice: Slice) -> None: bar = slice.bars.get(self._symbol) if bar: self.ema.update(bar.EndTime, bar.Close) if self.ema.is_ready: # The current value of self.ema is represented by self.ema.current.value self.plot("ExponentialMovingAverage", "ema", self.ema.current.value)
To register a manual indicator for automatic updates with the security data, call the RegisterIndicator
register_indicator
method.
public class ExponentialMovingAverageAlgorithm : QCAlgorithm { private Symbol _symbol; private ExponentialMovingAverage _ema; public override void Initialize() { _symbol = AddEquity("SPY", Resolution.Daily).Symbol; _ema = new ExponentialMovingAverage(20, 0.5); RegisterIndicator(_symbol, _ema, Resolution.Daily); } public override void OnData(Slice data) { if (_ema.IsReady) { // The current value of _ema is represented by itself (_ema) // or _ema.Current.Value Plot("ExponentialMovingAverage", "ema", _ema); } } }
class ExponentialMovingAverageAlgorithm(QCAlgorithm): def initialize(self) -> None: self._symbol = self.add_equity("SPY", Resolution.DAILY).symbol self.ema = ExponentialMovingAverage(20, 0.5) self.register_indicator(self._symbol, self.ema, Resolution.DAILY) def on_data(self, slice: Slice) -> None: if self.ema.is_ready: # The current value of self.ema is represented by self.ema.current.value self.plot("ExponentialMovingAverage", "ema", self.ema.current.value)
The following reference table describes the ExponentialMovingAverage
constructor:
ExponentialMovingAverage
Represents the traditional exponential moving average indicator (EMA). When the indicator is ready, the first value of the EMA is equivalent to the simple moving average. After the first EMA value, the EMA value is a function of the previous EMA value. Therefore, depending on the number of samples you feed into the indicator, it can provide different EMA values for a single security and lookback period. To make the indicator values consistent across time, warm up the indicator with all the trailing security price history.
get_enumerator()
Returns an enumerator that iterates through the history window.
IEnumerator[IndicatorDataPoint]
reset()
Resets this indicator to its initial state
to_detailed_string()
Provides a more detailed string of this indicator in the form of {Name} - {Value}
str
update(time, value)
Updates the state of this indicator with the given value and returns true if this indicator is ready, false otherwise
- time (datetime)
- value (float)
bool
update(input)
Updates the state of this indicator with the given value and returns true if this indicator is ready, false otherwise
- input (IBaseData)
bool
consolidators
The data consolidators associated with this indicator if any
The data consolidators associated with this indicator if any
ISet[IDataConsolidator]
current
Gets the current state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
Gets the current state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
IndicatorDataPoint
is_ready
Gets a flag indicating when this indicator is ready and fully initialized
Gets a flag indicating when this indicator is ready and fully initialized
bool
item
Indexes the history windows, where index 0 is the most recent indicator value. If index is greater or equal than the current count, it returns null. If the index is greater or equal than the window size, it returns null and resizes the windows to i + 1.
Indexes the history windows, where index 0 is the most recent indicator value. If index is greater or equal than the current count, it returns null. If the index is greater or equal than the window size, it returns null and resizes the windows to i + 1.
IndicatorDataPoint
name
Gets a name for this indicator
Gets a name for this indicator
str
previous
Gets the previous state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
Gets the previous state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
IndicatorDataPoint
samples
Gets the number of samples processed by this indicator
Gets the number of samples processed by this indicator
int
warm_up_period
Required period, in data points, for the indicator to be ready and fully initialized.
Required period, in data points, for the indicator to be ready and fully initialized.
int
window
A rolling window keeping a history of the indicator values of a given period
A rolling window keeping a history of the indicator values of a given period
RollingWindow[IndicatorDataPoint]
ExponentialMovingAverage
Represents the traditional exponential moving average indicator (EMA). When the indicator is ready, the first value of the EMA is equivalent to the simple moving average. After the first EMA value, the EMA value is a function of the previous EMA value. Therefore, depending on the number of samples you feed into the indicator, it can provide different EMA values for a single security and lookback period. To make the indicator values consistent across time, warm up the indicator with all the trailing security price history.
GetEnumerator()
Returns an enumerator that iterates through the history window.
IEnumerator[IndicatorDataPoint]
Reset()
Resets this indicator to its initial state
ToDetailedString()
Provides a more detailed string of this indicator in the form of {Name} - {Value}
String
Update(time, value)
Updates the state of this indicator with the given value and returns true if this indicator is ready, false otherwise
- time (DateTime)
- value (decimal)
Boolean
Update(input)
Updates the state of this indicator with the given value and returns true if this indicator is ready, false otherwise
- input (IBaseData)
Boolean
Consolidators
The data consolidators associated with this indicator if any
The data consolidators associated with this indicator if any
ISet<IDataConsolidator>
Current
Gets the current state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
Gets the current state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
IndicatorDataPoint
IsReady
Gets a flag indicating when this indicator is ready and fully initialized
Gets a flag indicating when this indicator is ready and fully initialized
bool
Name
Gets a name for this indicator
Gets a name for this indicator
string
Previous
Gets the previous state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
Gets the previous state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
IndicatorDataPoint
Samples
Gets the number of samples processed by this indicator
Gets the number of samples processed by this indicator
int
WarmUpPeriod
Required period, in data points, for the indicator to be ready and fully initialized.
Required period, in data points, for the indicator to be ready and fully initialized.
Int32
Window
A rolling window keeping a history of the indicator values of a given period
A rolling window keeping a history of the indicator values of a given period
RollingWindow<IndicatorDataPoint>
[System.Int32]
Indexes the history windows, where index 0 is the most recent indicator value. If index is greater or equal than the current count, it returns null. If the index is greater or equal than the window size, it returns null and resizes the windows to i + 1.
Indexes the history windows, where index 0 is the most recent indicator value. If index is greater or equal than the current count, it returns null. If the index is greater or equal than the window size, it returns null and resizes the windows to i + 1.
IndicatorDataPoint
Visualization
The following image shows plot values of selected properties of ExponentialMovingAverage
using the plotly library.
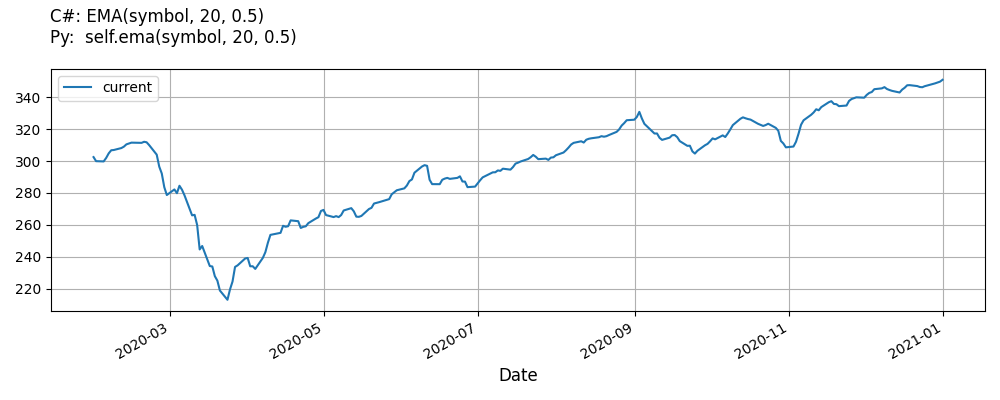