Supported Indicators
Volume Profile
Introduction
This indicator represents an Indicator of the Market Profile with Volume Profile mode and its attributes
To view the implementation of this indicator, see the LEAN GitHub repository.
Using VP Indicator
To create an automatic indicators for VolumeProfile
, call the VP
helper method from the QCAlgorithm
class. The VP
method creates a VolumeProfile
object, hooks it up for automatic updates, and returns it so you can used it in your algorithm. In most cases, you should call the helper method in the Initialize
initialize
method.
public class VolumeProfileAlgorithm : QCAlgorithm { private Symbol _symbol; private VolumeProfile _vp; public override void Initialize() { _symbol = AddEquity("SPY", Resolution.Daily).Symbol; _vp = VP(_symbol, 3, 0.70, 0.05); } public override void OnData(Slice data) { if (_vp.IsReady) { // The current value of _vp is represented by itself (_vp) // or _vp.Current.Value Plot("VolumeProfile", "vp", _vp); // Plot all properties of vp Plot("VolumeProfile", "profilehigh", _vp.ProfileHigh); Plot("VolumeProfile", "profilelow", _vp.ProfileLow); Plot("VolumeProfile", "pocprice", _vp.POCPrice); Plot("VolumeProfile", "pocvolume", _vp.POCVolume); Plot("VolumeProfile", "valueareavolume", _vp.ValueAreaVolume); Plot("VolumeProfile", "valueareahigh", _vp.ValueAreaHigh); Plot("VolumeProfile", "valuearealow", _vp.ValueAreaLow); } } }
class VolumeProfileAlgorithm(QCAlgorithm): def initialize(self) -> None: self._symbol = self.add_equity("SPY", Resolution.DAILY).symbol self._vp = self.vp(self._symbol, 3, 0.70, 0.05) def on_data(self, slice: Slice) -> None: if self._vp.is_ready: # The current value of self._vp is represented by self._vp.current.value self.plot("VolumeProfile", "vp", self._vp.current.value) # Plot all attributes of self._vp self.plot("VolumeProfile", "profile_high", self._vp.profile_high) self.plot("VolumeProfile", "profile_low", self._vp.profile_low) self.plot("VolumeProfile", "poc_price", self._vp.poc_price) self.plot("VolumeProfile", "poc_volume", self._vp.poc_volume) self.plot("VolumeProfile", "value_area_volume", self._vp.value_area_volume) self.plot("VolumeProfile", "value_area_high", self._vp.value_area_high) self.plot("VolumeProfile", "value_area_low", self._vp.value_area_low)
The following reference table describes the VP
method:
vp(symbol, period=2, value_area_volume_percentage=0.7, price_range_round_off=0.05, resolution=4, selector=None)
[source]Creates an Market Profile indicator for the symbol with Volume Profile (VOL) mode. The indicator will be automatically updated on the given resolution.
- symbol (Symbol) — The symbol whose VP we want
- period (int, optional) — The period of the VP
- value_area_volume_percentage (float, optional) — The percentage of volume contained in the value area
- price_range_round_off (float, optional) — How many digits you want to round and the precision. i.e 0.01 round to two digits exactly.
- resolution (Resolution, optional) — The resolution
- selector (Callable[IBaseData, TradeBar], optional) — Selects a value from the BaseData to send into the indicator, if null defaults to casting the input value to a TradeBar
The Volume Profile indicator for the given parameters
VP(symbol, period=2, valueAreaVolumePercentage=0.7, priceRangeRoundOff=0.05, resolution=4, selector=None)
[source]Creates an Market Profile indicator for the symbol with Volume Profile (VOL) mode. The indicator will be automatically updated on the given resolution.
- symbol (Symbol) — The symbol whose VP we want
- period (Int32, optional) — The period of the VP
- valueAreaVolumePercentage (decimal, optional) — The percentage of volume contained in the value area
- priceRangeRoundOff (decimal, optional) — How many digits you want to round and the precision. i.e 0.01 round to two digits exactly.
- resolution (Resolution, optional) — The resolution
- selector (Func<IBaseData, TradeBar>, optional) — Selects a value from the BaseData to send into the indicator, if null defaults to casting the input value to a TradeBar
The Volume Profile indicator for the given parameters
If you don't provide a resolution, it defaults to the security resolution. If you provide a resolution, it must be greater than or equal to the resolution of the security. For instance, if you subscribe to hourly data for a security, you should update its indicator with data that spans 1 hour or longer.
For more information about the selector argument, see Alternative Price Fields.
For more information about plotting indicators, see Plotting Indicators.
You can manually create a VolumeProfile
indicator, so it doesn't automatically update. Manual indicators let you update their values with any data you choose.
Updating your indicator manually enables you to control when the indicator is updated and what data you use to update it. To manually update the indicator, call the Update
update
method with a TradeBar
. The indicator will only be ready after you prime it with enough data.
public class VolumeProfileAlgorithm : QCAlgorithm { private Symbol _symbol; private VolumeProfile _vp; public override void Initialize() { _symbol = AddEquity("SPY", Resolution.Daily).Symbol; _vp = new VolumeProfile("", 3, 0.70, 0.05); } public override void OnData(Slice data) { if (data.Bars.TryGetValue(_symbol, out var bar)) { _vp.Update(bar); } if (_vp.IsReady) { // The current value of _vp is represented by itself (_vp) // or _vp.Current.Value Plot("VolumeProfile", "vp", _vp); // Plot all properties of vp Plot("VolumeProfile", "profilehigh", _vp.ProfileHigh); Plot("VolumeProfile", "profilelow", _vp.ProfileLow); Plot("VolumeProfile", "pocprice", _vp.POCPrice); Plot("VolumeProfile", "pocvolume", _vp.POCVolume); Plot("VolumeProfile", "valueareavolume", _vp.ValueAreaVolume); Plot("VolumeProfile", "valueareahigh", _vp.ValueAreaHigh); Plot("VolumeProfile", "valuearealow", _vp.ValueAreaLow); } } }
class VolumeProfileAlgorithm(QCAlgorithm): def initialize(self) -> None: self._symbol = self.add_equity("SPY", Resolution.DAILY).symbol self._vp = VolumeProfile("", 3, 0.70, 0.05) def on_data(self, slice: Slice) -> None: bar = slice.bars.get(self._symbol) if bar: self._vp.update(bar) if self._vp.is_ready: # The current value of self._vp is represented by self._vp.current.value self.plot("VolumeProfile", "vp", self._vp.current.value) # Plot all attributes of self._vp self.plot("VolumeProfile", "profile_high", self._vp.profile_high) self.plot("VolumeProfile", "profile_low", self._vp.profile_low) self.plot("VolumeProfile", "poc_price", self._vp.poc_price) self.plot("VolumeProfile", "poc_volume", self._vp.poc_volume) self.plot("VolumeProfile", "value_area_volume", self._vp.value_area_volume) self.plot("VolumeProfile", "value_area_high", self._vp.value_area_high) self.plot("VolumeProfile", "value_area_low", self._vp.value_area_low)
To register a manual indicator for automatic updates with the security data, call the RegisterIndicator
register_indicator
method.
public class VolumeProfileAlgorithm : QCAlgorithm { private Symbol _symbol; private VolumeProfile _vp; public override void Initialize() { _symbol = AddEquity("SPY", Resolution.Daily).Symbol; _vp = new VolumeProfile("", 3, 0.70, 0.05); RegisterIndicator(_symbol, _vp, Resolution.Daily); } public override void OnData(Slice data) { if (_vp.IsReady) { // The current value of _vp is represented by itself (_vp) // or _vp.Current.Value Plot("VolumeProfile", "vp", _vp); } } }
class VolumeProfileAlgorithm(QCAlgorithm): def initialize(self) -> None: self._symbol = self.add_equity("SPY", Resolution.DAILY).symbol self._vp = VolumeProfile("", 3, 0.70, 0.05) self.register_indicator(self._symbol, self._vp, Resolution.DAILY) def on_data(self, slice: Slice) -> None: if self._vp.is_ready: # The current value of self._vp is represented by self._vp.current.value self.plot("VolumeProfile", "vp", self._vp.current.value)
The following reference table describes the VolumeProfile
constructor:
VolumeProfile
Represents an Indicator of the Market Profile with Volume Profile mode and its attributes
get_enumerator()
Returns an enumerator that iterates through the history window.
IEnumerator[IndicatorDataPoint]
reset()
Resets this indicator to its initial state
to_detailed_string()
Provides a more detailed string of this indicator in the form of {Name} - {Value}
str
update(time, value)
Updates the state of this indicator with the given value and returns true if this indicator is ready, false otherwise
- time (datetime)
- value (float)
bool
update(input)
Updates the state of this indicator with the given value and returns true if this indicator is ready, false otherwise
- input (IBaseData)
bool
consolidators
The data consolidators associated with this indicator if any
The data consolidators associated with this indicator if any
ISet[IDataConsolidator]
current
Gets the current state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
Gets the current state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
IndicatorDataPoint
is_ready
Gets a flag indicating when the indicator is ready and fully initialized
Gets a flag indicating when the indicator is ready and fully initialized
bool
item
Indexes the history windows, where index 0 is the most recent indicator value. If index is greater or equal than the current count, it returns null. If the index is greater or equal than the window size, it returns null and resizes the windows to i + 1.
Indexes the history windows, where index 0 is the most recent indicator value. If index is greater or equal than the current count, it returns null. If the index is greater or equal than the window size, it returns null and resizes the windows to i + 1.
IndicatorDataPoint
name
Gets a name for this indicator
Gets a name for this indicator
str
poc_price
Price where the most trading occured (Point of Control(POC)) This price is MarketProfile.Current.Value
Price where the most trading occured (Point of Control(POC)) This price is MarketProfile.Current.Value
float
poc_volume
Volume where the most tradding occured (Point of Control(POC))
Volume where the most tradding occured (Point of Control(POC))
float
previous
Gets the previous state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
Gets the previous state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
IndicatorDataPoint
profile_high
The highest reached close price level during the period. That value is called Profile High
The highest reached close price level during the period. That value is called Profile High
float
profile_low
The lowest reached close price level during the period. That value is called Profile Low
The lowest reached close price level during the period. That value is called Profile Low
float
samples
Gets the number of samples processed by this indicator
Gets the number of samples processed by this indicator
int
value_area_high
The highest close price level within the value area
The highest close price level within the value area
float
value_area_low
The lowest close price level within the value area
The lowest close price level within the value area
float
value_area_volume
The range of price levels in which a specified percentage of all volume was traded during the time period. Typically, this percentage is set to 70% however it is up to the trader’s discretion.
The range of price levels in which a specified percentage of all volume was traded during the time period. Typically, this percentage is set to 70% however it is up to the trader’s discretion.
float
volume_per_price
Get a copy of the _volumePerPrice field
Get a copy of the _volumePerPrice field
SortedList[float, float]
warm_up_period
Required period, in data points, for the indicator to be ready and fully initialized.
Required period, in data points, for the indicator to be ready and fully initialized.
int
window
A rolling window keeping a history of the indicator values of a given period
A rolling window keeping a history of the indicator values of a given period
RollingWindow[IndicatorDataPoint]
VolumeProfile
Represents an Indicator of the Market Profile with Volume Profile mode and its attributes
GetEnumerator()
Returns an enumerator that iterates through the history window.
IEnumerator[IndicatorDataPoint]
Reset()
Resets this indicator to its initial state
ToDetailedString()
Provides a more detailed string of this indicator in the form of {Name} - {Value}
String
Update(time, value)
Updates the state of this indicator with the given value and returns true if this indicator is ready, false otherwise
- time (DateTime)
- value (decimal)
Boolean
Update(input)
Updates the state of this indicator with the given value and returns true if this indicator is ready, false otherwise
- input (IBaseData)
Boolean
Consolidators
The data consolidators associated with this indicator if any
The data consolidators associated with this indicator if any
ISet<IDataConsolidator>
Current
Gets the current state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
Gets the current state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
IndicatorDataPoint
IsReady
Gets a flag indicating when the indicator is ready and fully initialized
Gets a flag indicating when the indicator is ready and fully initialized
bool
Name
Gets a name for this indicator
Gets a name for this indicator
string
POCPrice
Price where the most trading occured (Point of Control(POC)) This price is MarketProfile.Current.Value
Price where the most trading occured (Point of Control(POC)) This price is MarketProfile.Current.Value
decimal
POCVolume
Volume where the most tradding occured (Point of Control(POC))
Volume where the most tradding occured (Point of Control(POC))
decimal
Previous
Gets the previous state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
Gets the previous state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
IndicatorDataPoint
ProfileHigh
The highest reached close price level during the period. That value is called Profile High
The highest reached close price level during the period. That value is called Profile High
decimal
ProfileLow
The lowest reached close price level during the period. That value is called Profile Low
The lowest reached close price level during the period. That value is called Profile Low
decimal
Samples
Gets the number of samples processed by this indicator
Gets the number of samples processed by this indicator
int
ValueAreaHigh
The highest close price level within the value area
The highest close price level within the value area
decimal
ValueAreaLow
The lowest close price level within the value area
The lowest close price level within the value area
decimal
ValueAreaVolume
The range of price levels in which a specified percentage of all volume was traded during the time period. Typically, this percentage is set to 70% however it is up to the trader’s discretion.
The range of price levels in which a specified percentage of all volume was traded during the time period. Typically, this percentage is set to 70% however it is up to the trader’s discretion.
decimal
VolumePerPrice
Get a copy of the _volumePerPrice field
Get a copy of the _volumePerPrice field
SortedList<Decimal, Decimal>
WarmUpPeriod
Required period, in data points, for the indicator to be ready and fully initialized.
Required period, in data points, for the indicator to be ready and fully initialized.
Int32
Window
A rolling window keeping a history of the indicator values of a given period
A rolling window keeping a history of the indicator values of a given period
RollingWindow<IndicatorDataPoint>
[System.Int32]
Indexes the history windows, where index 0 is the most recent indicator value. If index is greater or equal than the current count, it returns null. If the index is greater or equal than the window size, it returns null and resizes the windows to i + 1.
Indexes the history windows, where index 0 is the most recent indicator value. If index is greater or equal than the current count, it returns null. If the index is greater or equal than the window size, it returns null and resizes the windows to i + 1.
IndicatorDataPoint
Visualization
The following image shows plot values of selected properties of VolumeProfile
using the plotly library.
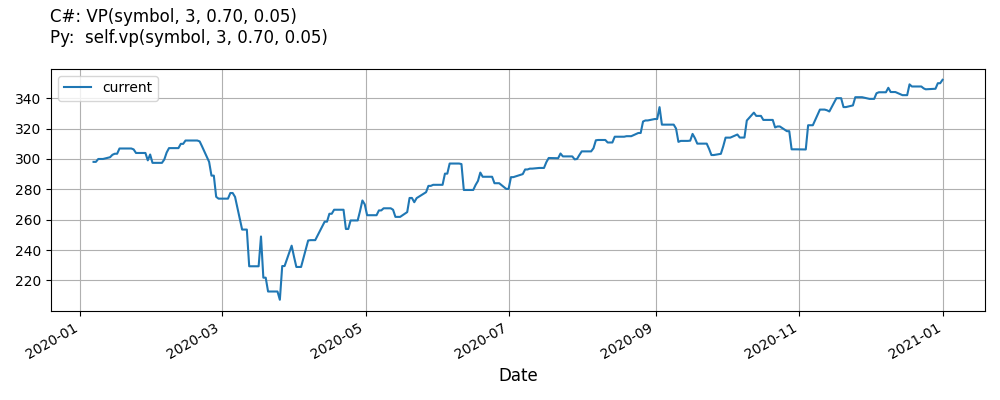
Indicator History
To get the historical data of the VolumeProfile
indicator, call the IndicatorHistory
self.indicator_history
method.
This method resets your indicator, makes a history request, and updates the indicator with the historical data.
Just like with regular history requests, the IndicatorHistory
indicator_history
method supports time periods based on a trailing number of bars, a trailing period of time, or a defined period of time.
If you don't provide a resolution
argument, it defaults to match the resolution of the security subscription.
public class VolumeProfileAlgorithm : QCAlgorithm { private Symbol _symbol; public override void Initialize() { _symbol = AddEquity("SPY", Resolution.Daily).Symbol; var vp = VP(_symbol, 3, 0.70, 0.05); var countIndicatorHistory = IndicatorHistory(vp, _symbol, 100, Resolution.Minute); var timeSpanIndicatorHistory = IndicatorHistory(vp, _symbol, TimeSpan.FromDays(10), Resolution.Minute); var timePeriodIndicatorHistory = IndicatorHistory(vp, _symbol, new DateTime(2024, 7, 1), new DateTime(2024, 7, 5), Resolution.Minute); } }
class VolumeProfileAlgorithm(QCAlgorithm): def initialize(self) -> None: self._symbol = self.add_equity("SPY", Resolution.DAILY).symbol vp = self.vp(self._symbol, 3, 0.70, 0.05) count_indicator_history = self.indicator_history(vp, self._symbol, 100, Resolution.MINUTE) timedelta_indicator_history = self.indicator_history(vp, self._symbol, timedelta(days=10), Resolution.MINUTE) time_period_indicator_history = self.indicator_history(vp, self._symbol, datetime(2024, 7, 1), datetime(2024, 7, 5), Resolution.MINUTE)
To make the IndicatorHistory
indicator_history
method update the indicator with an alternative price field instead of the close (or mid-price) of each bar, pass a selector
argument.
var indicatorHistory = IndicatorHistory(vp, 100, Resolution.Minute, (bar) => ((TradeBar)bar).High);
indicator_history = self.indicator_history(vp, 100, Resolution.MINUTE, lambda bar: bar.high) indicator_history_df = indicator_history.data_frame
If you already have a list of Slice objects, you can pass them to the IndicatorHistory
indicator_history
method to avoid the internal history request.
var history = History(_symbol, 100, Resolution.Minute); var historyIndicatorHistory = IndicatorHistory(vp, history);