Option Strategies
Short Put Butterfly
Introduction
Short Put butterfly is the combination of a bull put spread and a bear put spread. In this strategy, all the puts have the same underlying stock, the same expiration date, and the strike price distance of ITM-ATM and OTM-ATM put pairs are the same. The short put butterfly strategy consists of selling an ITM put, selling an OTM put, and buying 2 ATM puts. This strategy profits from a drastic change in underlying price.
Implementation
Follow these steps to implement the short put butterfly strategy:
- In the
Initialize
initialize
method, set the start date, end date, cash, and Option universe. - In the
OnData
on_data
method, select strikes and expiration date of the contracts in the strategy legs. - In the
OnData
on_data
method, call theOptionStrategies.ShortButterflyPut
method and then submit the order.
private Symbol _symbol; public override void Initialize() { SetStartDate(2017, 2, 1); SetEndDate(2017, 3, 5); SetCash(500000); UniverseSettings.Asynchronous = true; var option = AddOption("GOOG", Resolution.Minute); _symbol = option.Symbol; option.SetFilter(universe => universe.IncludeWeeklys().Strikes(-15, 15).Expiration(0, 31)); }
def initialize(self) -> None: self.set_start_date(2017, 2, 1) self.set_end_date(2017, 3, 5) self.set_cash(500000) self.universe_settings.asynchronous = True option = self.add_option("GOOG", Resolution.MINUTE) self._symbol = option.symbol option.set_filter(lambda universe: universe.include_weeklys().strikes(-15, 15).expiration(0, 31))
public override void OnData(Slice slice) { if (Portfolio.Invested) return; // Get the OptionChain var chain = slice.OptionChains.get(_symbol, null); if (chain == null || chain.Count() == 0) return; // Select an expiry date var expiry = chain.OrderByDescending(x => x.Expiry).First().Expiry; // Select the put contracts that expire on the selected date var puts = chain.Where(x => x.Expiry == expiry && x.Right == OptionRight.Put); if (puts.Count() == 0) return; // Sort the put contracts by their strike prices var putStrikes = puts.Select(x => x.Strike).OrderBy(x => x); // Get the ATM strike price var atmStrike = puts.OrderBy(x => Math.Abs(x.Strike - chain.Underlying.Price)).First().Strike; // Get the distance between lowest strike price and ATM strike, and highest strike price and ATM strike // Get the lower value as the spread distance as equidistance is needed for both sides var spread = Math.Min(Math.Abs(putStrikes.First() - atmStrike), Math.Abs(putStrikes.Last() - atmStrike)); // Select the strike prices of the strategy legs var itmStrike = atmStrike + spread; var otmStrike = atmStrike - spread;
def on_data(self, slice: Slice) -> None: if self.portfolio.invested: return # Get the OptionChain chain = slice.option_chains.get(self.symbol, None) if not chain: return # Select an expiry date expiry = sorted(chain, key = lambda x: x.expiry, reverse=True)[0].expiry # Select the put contracts that expire on the selected date puts = [i for i in chain if i.expiry == expiry and i.right == OptionRight.PUT] if len(puts) == 0: return # Sort the put contracts by their strike prices put_strikes = sorted([x.strike for x in puts]) # Get the ATM strike price atm_strike = sorted(puts, key=lambda x: abs(x.strike - chain.underlying.price))[0].strike # Get the distance between lowest strike price and ATM strike, and highest strike price and ATM strike. # Get the lower value as the spread distance as equidistance is needed for both sides spread = min(abs(put_strikes[0] - atm_strike), abs(put_strikes[-1] - atm_strike)) # Select the strike prices of the strategy legs itm_strike = atm_strike + spread otm_strike = atm_strike - spread
var optionStrategy = OptionStrategies.ShortButterflyPut(_symbol, itmStrike, atmStrike, otmStrike, expiry); Buy(optionStrategy, 1);
option_strategy = OptionStrategies.short_butterfly_put(self.symbol, itm_strike, atm_strike, otm_strike, expiry) self.buy(option_strategy, 1)
Option strategies synchronously execute by default. To asynchronously execute Option strategies, set the asynchronous
argument to False
false
. You can also provide a tag and order properties to the
Buy
method.
Buy(optionStrategy, quantity, asynchronous, tag, orderProperties);
self.Buy(option_strategy, quantity, asynchronous, tag, order_properties)
Strategy Payoff
Short Put Butterfly
The short put butterfly is a limited-reward-limited-risk strategy. The payoff is
$$ \begin{array}{rcll} P^{OTM}_T & = & (K^{OTM} - S_T)^{+}\\ P^{ITM}_T & = & (K^{ITM} - S_T)^{+}\\ P^{ATM}_T & = & (K^{ATM} - S_T)^{+}\\ P_T & = & (2\times P^{ATM}_T - P^{OTM}_T - P^{ITM}_T - 2\times P^{ATM}_0 + P^{ITM}_0 + P^{OTM}_0)\times m - fee \end{array} $$ $$ \begin{array}{rcll} \textrm{where} & P^{OTM}_T & = & \textrm{OTM put value at time T}\\ & P^{ITM}_T & = & \textrm{ITM put value at time T}\\ & P^{ATM}_T & = & \textrm{ATM put value at time T}\\ & S_T & = & \textrm{Underlying asset price at time T}\\ & K^{OTM} & = & \textrm{OTM put strike price}\\ & K^{ITM} & = & \textrm{ITM put strike price}\\ & K^{ATM} & = & \textrm{ATM put strike price}\\ & P_T & = & \textrm{Payout total at time T}\\ & P^{ITM}_0 & = & \textrm{ITM put value at position opening (credit received)}\\ & P^{OTM}_0 & = & \textrm{OTM put value at position opening (credit received)}\\ & P^{ATM}_0 & = & \textrm{ATM put value at position opening (debit paid)}\\ & m & = & \textrm{Contract multiplier}\\ & T & = & \textrm{Time of expiration} \end{array} $$The following chart shows the payoff at expiration:
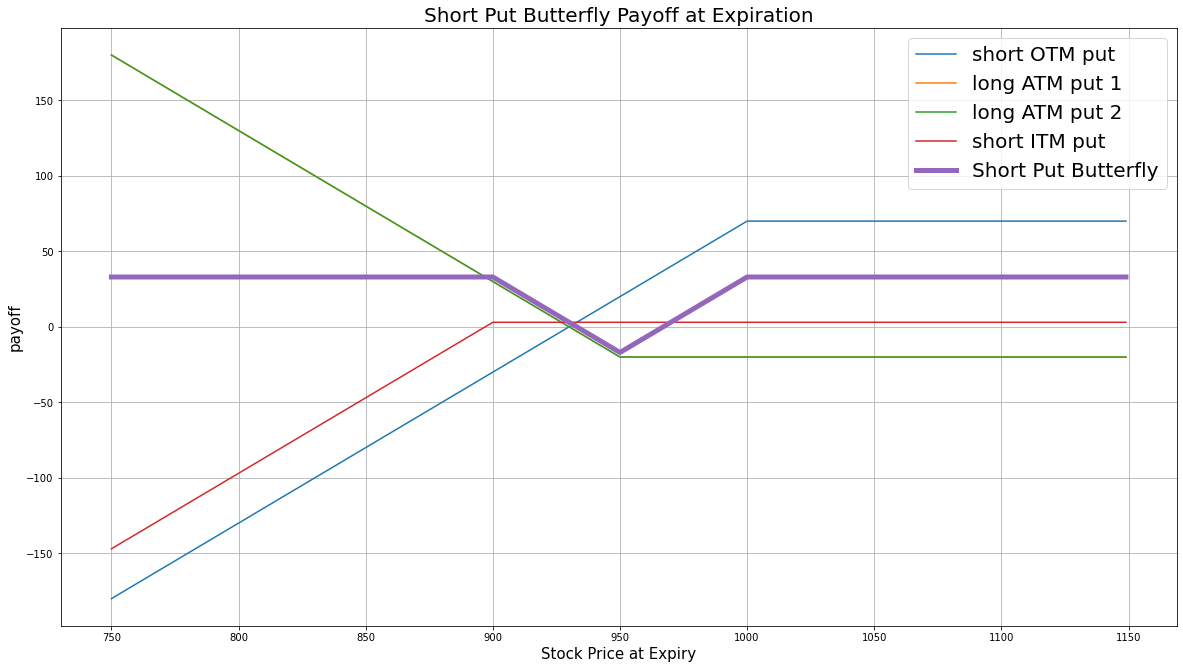
The maximum profit is the net credit received, $P^{ITM}_0 + P^{OTM}_0 - 2\times P^{ATM}_0$. It occurs when the underlying price is below the ITM strike or above the OTM strike at expiration.
The maximum loss is $K^{ATM} - K^{OTM} - 2\times P^{ATM}_0 + P^{ITM}_0 + P^{OTM}_0$. It occurs when the underlying price at expiration is at the same price as when you opened the trade.
If the Option is American Option, there is risk of early assignment on the sold contracts.
Example
The following table shows the price details of the assets in the short put butterfly algorithm:
Asset | Price ($) | Strike ($) |
---|---|---|
ITM put | 37.80 | 832.50 |
ATM put | 14.70 | 800.00 |
OTM put | 5.70 | 767.50 |
Underlying Equity at expiration | 829.08 | - |
Therefore, the payoff is
$$ \begin{array}{rcll} P^{OTM}_T & = & (K^{OTM} - S_T)^{+}\\ & = & (829.08-832.50)^{+}\\ & = & 0\\ P^{ITM}_T & = & (K^{ITM} - S_T)^{+}\\ & = & (829.08-767.50)^{+}\\ & = & 61.58\\ P^{ATM}_T & = & (K^{ATM} - S_T)^{+}\\ & = & (829.08-800.00)^{+}\\ & = & 29.08\\ P_T & = & (-P^{OTM}_T - P^{ITM}_T + 2\times P^{ATM}_T - 2\times P^{ATM}_0 + P^{ITM}_0 + P^{OTM}_0)\times m - fee\\ & = & (-61.58-0+29.08\times2+5.70+37.80-14.70\times2)\times100-1.00\times4\\ & = & 1064 \end{array} $$So, the strategy gains $1,064.
The following algorithm implements a short put butterfly Option strategy: