Supported Indicators
Theta
Introduction
Option Theta indicator that calculate the theta of an option
To view the implementation of this indicator, see the LEAN GitHub repository.
Using T Indicator
To create an automatic indicators for Theta
, call the T
helper method from the QCAlgorithm
class. The T
method creates a Theta
object, hooks it up for automatic updates, and returns it so you can used it in your algorithm. In most cases, you should call the helper method in the Initialize
initialize
method.
public class ThetaAlgorithm : QCAlgorithm { private Symbol _symbol; private Symbol _option, _mirrorOption; private Theta _t; public override void Initialize() { _symbol = AddEquity("SPY", Resolution.Daily).Symbol; _option = QuantConnect.Symbol.CreateOption("SPY", Market.USA, OptionStyle.American, OptionRight.Put, 450m, new DateTime(2023, 12, 22)); AddOptionContract(_option, Resolution.Daily); _mirrorOption = QuantConnect.Symbol.CreateOption("SPY", Market.USA, OptionStyle.American, OptionRight.Call, 450m, new DateTime(2023, 12, 22)); AddOptionContract(_mirrorOption, Resolution.Daily); _t = T(_option, _mirrorOption); } public override void OnData(Slice data) { if (_t.IsReady) { // The current value of _t is represented by itself (_t) // or _t.Current.Value Plot("Theta", "t", _t); // Plot all properties of t Plot("Theta", "impliedvolatility", _t.ImpliedVolatility); Plot("Theta", "optionsymbol", _t.OptionSymbol); Plot("Theta", "riskfreerate", _t.RiskFreeRate); Plot("Theta", "dividendyield", _t.DividendYield); Plot("Theta", "price", _t.Price); Plot("Theta", "oppositeprice", _t.OppositePrice); Plot("Theta", "underlyingprice", _t.UnderlyingPrice); } } }
class ThetaAlgorithm(QCAlgorithm): def initialize(self) -> None: self._symbol = self.add_equity("SPY", Resolution.DAILY).symbol self.option = Symbol.create_option("SPY", Market.USA, OptionStyle.AMERICAN, OptionRight.PUT, 450, datetime(2023, 12, 22)) self.add_option_contract(self.option, Resolution.DAILY) self.mirror_option = Symbol.create_option("SPY", Market.USA, OptionStyle.AMERICAN, OptionRight.CALL, 450, datetime(2023, 12, 22)) self.add_option_contract(self.mirror_option, Resolution.DAILY) self._t = self.t(self.option, self.mirror_option) def on_data(self, slice: Slice) -> None: if self._t.is_ready: # The current value of self._t is represented by self._t.current.value self.plot("Theta", "t", self._t.current.value) # Plot all attributes of self._t self.plot("Theta", "implied_volatility", self._t.implied_volatility.current.value) self.plot("Theta", "option_symbol", self._t.option_symbol.current.value) self.plot("Theta", "risk_free_rate", self._t.risk_free_rate.current.value) self.plot("Theta", "dividend_yield", self._t.dividend_yield.current.value) self.plot("Theta", "price", self._t.price.current.value) self.plot("Theta", "opposite_price", self._t.opposite_price.current.value) self.plot("Theta", "underlying_price", self._t.underlying_price.current.value)
The following reference table describes the T
method:
t(symbol, mirror_option=None, risk_free_rate=None, dividend_yield=None, option_model=0, iv_model=None, resolution=None)
[source]For the given symbol will resolve the ticker it used at the current algorithm date
- symbol (Symbol) — The option symbol whose values we want as an indicator
- mirror_option (Symbol, optional) — The mirror option for parity calculation
- risk_free_rate (float, optional) — The risk free rate
- dividend_yield (float, optional) — The dividend yield
- option_model (OptionPricingModelType, optional) — The option pricing model used to estimate Theta
- iv_model (OptionPricingModelType, optional) — The option pricing model used to estimate IV
- resolution (Resolution, optional) — The desired resolution of the data
A new Theta indicator for the specified symbol
T(symbol, mirrorOption=None, riskFreeRate=None, dividendYield=None, optionModel=0, ivModel=None, resolution=None)
[source]For the given symbol will resolve the ticker it used at the current algorithm date
- symbol (Symbol) — The option symbol whose values we want as an indicator
- mirrorOption (Symbol, optional) — The mirror option for parity calculation
- riskFreeRate (decimal, optional) — The risk free rate
- dividendYield (decimal, optional) — The dividend yield
- optionModel (OptionPricingModelType, optional) — The option pricing model used to estimate Theta
- ivModel (OptionPricingModelType, optional) — The option pricing model used to estimate IV
- resolution (Resolution, optional) — The desired resolution of the data
A new Theta indicator for the specified symbol
If you don't provide a resolution, it defaults to the security resolution. If you provide a resolution, it must be greater than or equal to the resolution of the security. For instance, if you subscribe to hourly data for a security, you should update its indicator with data that spans 1 hour or longer.
For more information about the selector argument, see Alternative Price Fields.
For more information about plotting indicators, see Plotting Indicators.
You can manually create a Theta
indicator, so it doesn't automatically update. Manual indicators let you update their values with any data you choose.
Updating your indicator manually enables you to control when the indicator is updated and what data you use to update it. To manually update the indicator, call the Update
update
method with time/number pair or an IndicatorDataPoint
. The indicator will only be ready after you prime it with enough data.
public class ThetaAlgorithm : QCAlgorithm { private Symbol _symbol; private Symbol _option, _mirrorOption; private Theta _t; public override void Initialize() { _symbol = AddEquity("SPY", Resolution.Daily).Symbol; _option = QuantConnect.Symbol.CreateOption("SPY", Market.USA, OptionStyle.American, OptionRight.Put, 450m, new DateTime(2023, 12, 22)); AddOptionContract(_option, Resolution.Daily); _mirrorOption = QuantConnect.Symbol.CreateOption("SPY", Market.USA, OptionStyle.American, OptionRight.Call, 450m, new DateTime(2023, 12, 22)); AddOptionContract(_mirrorOption, Resolution.Daily); _t = new Theta(_option, interest_rate_model, dividend_yield_model, _mirrorOption); } public override void OnData(Slice data) { if (data.Bars.TryGetValue(_symbol, out var bar)) { _t.Update(new IndicatorDataPoint(_symbol, bar.EndTime, bar.Close)); } if (data.QuoteBars.TryGetValue(_option, out bar)) { _t.Update(new IndicatorDataPoint(_option, bar.EndTime, bar.Close)); } if (data.QuoteBars.TryGetValue(_mirrorOption, out bar)) { _t.Update(new IndicatorDataPoint(_mirrorOption, bar.EndTime, bar.Close)); } if (_t.IsReady) { // The current value of _t is represented by itself (_t) // or _t.Current.Value Plot("Theta", "t", _t); // Plot all properties of t Plot("Theta", "impliedvolatility", _t.ImpliedVolatility); Plot("Theta", "optionsymbol", _t.OptionSymbol); Plot("Theta", "riskfreerate", _t.RiskFreeRate); Plot("Theta", "dividendyield", _t.DividendYield); Plot("Theta", "price", _t.Price); Plot("Theta", "oppositeprice", _t.OppositePrice); Plot("Theta", "underlyingprice", _t.UnderlyingPrice); } } }
class ThetaAlgorithm(QCAlgorithm): def initialize(self) -> None: self._symbol = self.add_equity("SPY", Resolution.DAILY).symbol self.option = Symbol.create_option("SPY", Market.USA, OptionStyle.AMERICAN, OptionRight.PUT, 450, datetime(2023, 12, 22)) self.add_option_contract(self.option, Resolution.DAILY) self.mirror_option = Symbol.create_option("SPY", Market.USA, OptionStyle.AMERICAN, OptionRight.CALL, 450, datetime(2023, 12, 22)) self.add_option_contract(self.mirror_option, Resolution.DAILY) self._t = Theta(self.option, interest_rate_model, dividend_yield_model, self.mirror_option) def on_data(self, slice: Slice) -> None: bar = slice.bars.get(self._symbol) if bar: self._t.update(IndicatorDataPoint(self._symbol, bar.end_time, bar.close)) bar = slice.quote_bars.get(self.option) if bar: self._t.update(IndicatorDataPoint(self.option, bar.end_time, bar.close)) bar = slice.quote_bars.get(self.mirror_option) if bar: self._t.update(IndicatorDataPoint(self.mirror_option, bar.end_time, bar.close)) if self._t.is_ready: # The current value of self._t is represented by self._t.current.value self.plot("Theta", "t", self._t.current.value) # Plot all attributes of self._t self.plot("Theta", "implied_volatility", self._t.implied_volatility.current.value) self.plot("Theta", "option_symbol", self._t.option_symbol.current.value) self.plot("Theta", "risk_free_rate", self._t.risk_free_rate.current.value) self.plot("Theta", "dividend_yield", self._t.dividend_yield.current.value) self.plot("Theta", "price", self._t.price.current.value) self.plot("Theta", "opposite_price", self._t.opposite_price.current.value) self.plot("Theta", "underlying_price", self._t.underlying_price.current.value)
To register a manual indicator for automatic updates with the security data, call the RegisterIndicator
register_indicator
method.
public class ThetaAlgorithm : QCAlgorithm { private Symbol _symbol; private Symbol _option, _mirrorOption; private Theta _t; public override void Initialize() { _symbol = AddEquity("SPY", Resolution.Daily).Symbol; _option = QuantConnect.Symbol.CreateOption("SPY", Market.USA, OptionStyle.American, OptionRight.Put, 450m, new DateTime(2023, 12, 22)); AddOptionContract(_option, Resolution.Daily); _mirrorOption = QuantConnect.Symbol.CreateOption("SPY", Market.USA, OptionStyle.American, OptionRight.Call, 450m, new DateTime(2023, 12, 22)); AddOptionContract(_mirrorOption, Resolution.Daily); _t = new Theta(_option, interest_rate_model, dividend_yield_model, _mirrorOption); RegisterIndicator(_symbol, _t, Resolution.Daily); RegisterIndicator(_option, _t, Resolution.Daily); RegisterIndicator(_mirrorOption, _t, Resolution.Daily); } public override void OnData(Slice data) { if (_t.IsReady) { // The current value of _t is represented by itself (_t) // or _t.Current.Value Plot("Theta", "t", _t); // Plot all properties of t Plot("Theta", "impliedvolatility", _t.ImpliedVolatility); Plot("Theta", "optionsymbol", _t.OptionSymbol); Plot("Theta", "riskfreerate", _t.RiskFreeRate); Plot("Theta", "dividendyield", _t.DividendYield); Plot("Theta", "price", _t.Price); Plot("Theta", "oppositeprice", _t.OppositePrice); Plot("Theta", "underlyingprice", _t.UnderlyingPrice); } } }
class ThetaAlgorithm(QCAlgorithm): def initialize(self) -> None: self._symbol = self.add_equity("SPY", Resolution.DAILY).symbol self.option = Symbol.create_option("SPY", Market.USA, OptionStyle.AMERICAN, OptionRight.PUT, 450, datetime(2023, 12, 22)) self.add_option_contract(self.option, Resolution.DAILY) self.mirror_option = Symbol.create_option("SPY", Market.USA, OptionStyle.AMERICAN, OptionRight.CALL, 450, datetime(2023, 12, 22)) self.add_option_contract(self.mirror_option, Resolution.DAILY) self._t = Theta(self.option, interest_rate_model, dividend_yield_model, self.mirror_option) self.register_indicator(self._symbol, self._t, Resolution.DAILY) self.register_indicator(self.option, self._t, Resolution.DAILY) self.register_indicator(self.mirror_option, self._t, Resolution.DAILY) def on_data(self, slice: Slice) -> None: if self._t.is_ready: # The current value of self._t is represented by self._t.current.value self.plot("Theta", "t", self._t.current.value) # Plot all attributes of self._t self.plot("Theta", "implied_volatility", self._t.implied_volatility.current.value) self.plot("Theta", "option_symbol", self._t.option_symbol.current.value) self.plot("Theta", "risk_free_rate", self._t.risk_free_rate.current.value) self.plot("Theta", "dividend_yield", self._t.dividend_yield.current.value) self.plot("Theta", "price", self._t.price.current.value) self.plot("Theta", "opposite_price", self._t.opposite_price.current.value) self.plot("Theta", "underlying_price", self._t.underlying_price.current.value)
The following reference table describes the Theta
constructor:
Theta
Option Theta indicator that calculate the theta of an option
get_enumerator()
Returns an enumerator that iterates through the history window.
IEnumerator[IndicatorDataPoint]
reset()
Resets this indicator and all sub-indicators
to_detailed_string()
Provides a more detailed string of this indicator in the form of {Name} - {Value}
str
update(time, value)
Updates the state of this indicator with the given value and returns true if this indicator is ready, false otherwise
- time (datetime)
- value (float)
bool
update(input)
Updates the state of this indicator with the given value and returns true if this indicator is ready, false otherwise
- input (IBaseData)
bool
consolidators
The data consolidators associated with this indicator if any
The data consolidators associated with this indicator if any
ISet[IDataConsolidator]
current
Gets the current state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
Gets the current state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
IndicatorDataPoint
dividend_yield
Dividend Yield
Dividend Yield
Identity
expiry
Gets the expiration time of the option
Gets the expiration time of the option
datetime
implied_volatility
Gets the implied volatility of the option
Gets the implied volatility of the option
ImpliedVolatility
is_ready
Gets a flag indicating when this indicator is ready and fully initialized
Gets a flag indicating when this indicator is ready and fully initialized
bool
item
Indexes the history windows, where index 0 is the most recent indicator value. If index is greater or equal than the current count, it returns null. If the index is greater or equal than the window size, it returns null and resizes the windows to i + 1.
Indexes the history windows, where index 0 is the most recent indicator value. If index is greater or equal than the current count, it returns null. If the index is greater or equal than the window size, it returns null and resizes the windows to i + 1.
IndicatorDataPoint
name
Gets a name for this indicator
Gets a name for this indicator
str
opposite_price
Gets the mirror option price level, for implied volatility
Gets the mirror option price level, for implied volatility
IndicatorBase[IndicatorDataPoint]
option_symbol
Option's symbol object
Option's symbol object
Symbol
previous
Gets the previous state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
Gets the previous state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
IndicatorDataPoint
price
Gets the option price level
Gets the option price level
IndicatorBase[IndicatorDataPoint]
right
Gets the option right (call/put) of the option
Gets the option right (call/put) of the option
OptionRight
risk_free_rate
Risk Free Rate
Risk Free Rate
Identity
samples
Gets the number of samples processed by this indicator
Gets the number of samples processed by this indicator
int
strike
Gets the strike price of the option
Gets the strike price of the option
float
style
Gets the option style (European/American) of the option
Gets the option style (European/American) of the option
OptionStyle
underlying_price
Gets the underlying's price level
Gets the underlying's price level
IndicatorBase[IndicatorDataPoint]
use_mirror_contract
Flag if mirror option is implemented for parity type calculation
Flag if mirror option is implemented for parity type calculation
bool
warm_up_period
Required period, in data points, for the indicator to be ready and fully initialized.
Required period, in data points, for the indicator to be ready and fully initialized.
int
window
A rolling window keeping a history of the indicator values of a given period
A rolling window keeping a history of the indicator values of a given period
RollingWindow[IndicatorDataPoint]
Theta
Option Theta indicator that calculate the theta of an option
GetEnumerator()
Returns an enumerator that iterates through the history window.
IEnumerator[IndicatorDataPoint]
Reset()
Resets this indicator and all sub-indicators
ToDetailedString()
Provides a more detailed string of this indicator in the form of {Name} - {Value}
String
Update(time, value)
Updates the state of this indicator with the given value and returns true if this indicator is ready, false otherwise
- time (DateTime)
- value (decimal)
Boolean
Update(input)
Updates the state of this indicator with the given value and returns true if this indicator is ready, false otherwise
- input (IBaseData)
Boolean
Consolidators
The data consolidators associated with this indicator if any
The data consolidators associated with this indicator if any
ISet<IDataConsolidator>
Current
Gets the current state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
Gets the current state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
IndicatorDataPoint
DividendYield
Dividend Yield
Dividend Yield
Identity
Expiry
Gets the expiration time of the option
Gets the expiration time of the option
DateTime
ImpliedVolatility
Gets the implied volatility of the option
Gets the implied volatility of the option
ImpliedVolatility
IsReady
Gets a flag indicating when this indicator is ready and fully initialized
Gets a flag indicating when this indicator is ready and fully initialized
bool
Name
Gets a name for this indicator
Gets a name for this indicator
string
OppositePrice
Gets the mirror option price level, for implied volatility
Gets the mirror option price level, for implied volatility
IndicatorBase<IndicatorDataPoint>
OptionSymbol
Option's symbol object
Option's symbol object
Symbol
Previous
Gets the previous state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
Gets the previous state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
IndicatorDataPoint
Price
Gets the option price level
Gets the option price level
IndicatorBase<IndicatorDataPoint>
Right
Gets the option right (call/put) of the option
Gets the option right (call/put) of the option
OptionRight
RiskFreeRate
Risk Free Rate
Risk Free Rate
Identity
Samples
Gets the number of samples processed by this indicator
Gets the number of samples processed by this indicator
int
Strike
Gets the strike price of the option
Gets the strike price of the option
decimal
Style
Gets the option style (European/American) of the option
Gets the option style (European/American) of the option
OptionStyle
UnderlyingPrice
Gets the underlying's price level
Gets the underlying's price level
IndicatorBase<IndicatorDataPoint>
UseMirrorContract
Flag if mirror option is implemented for parity type calculation
Flag if mirror option is implemented for parity type calculation
bool
WarmUpPeriod
Required period, in data points, for the indicator to be ready and fully initialized.
Required period, in data points, for the indicator to be ready and fully initialized.
Int32
Window
A rolling window keeping a history of the indicator values of a given period
A rolling window keeping a history of the indicator values of a given period
RollingWindow<IndicatorDataPoint>
[System.Int32]
Indexes the history windows, where index 0 is the most recent indicator value. If index is greater or equal than the current count, it returns null. If the index is greater or equal than the window size, it returns null and resizes the windows to i + 1.
Indexes the history windows, where index 0 is the most recent indicator value. If index is greater or equal than the current count, it returns null. If the index is greater or equal than the window size, it returns null and resizes the windows to i + 1.
IndicatorDataPoint
Visualization
The following image shows plot values of selected properties of Theta
using the plotly library.
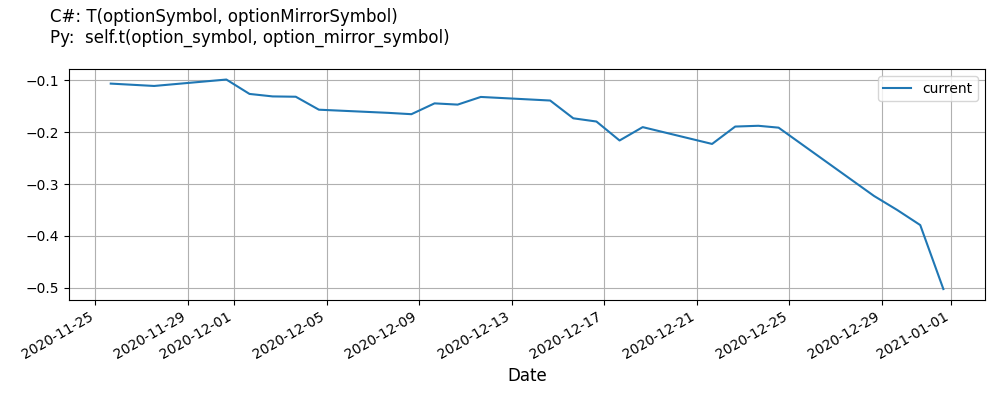
Indicator History
To get the historical data of the Theta
indicator, call the IndicatorHistory
self.indicator_history
method.
This method resets your indicator, makes a history request, and updates the indicator with the historical data.
Just like with regular history requests, the IndicatorHistory
indicator_history
method supports time periods based on a trailing number of bars, a trailing period of time, or a defined period of time.
If you don't provide a resolution
argument, it defaults to match the resolution of the security subscription.
public class ThetaAlgorithm : QCAlgorithm { private Symbol _symbol; private Symbol _option, _mirrorOption; public override void Initialize() { _symbol = AddEquity("SPY", Resolution.Daily).Symbol; _option = QuantConnect.Symbol.CreateOption("SPY", Market.USA, OptionStyle.American, OptionRight.Put, 225m, new DateTime(2024, 7, 12)); AddOptionContract(_option, Resolution.Daily); _mirrorOption = QuantConnect.Symbol.CreateOption("SPY", Market.USA, OptionStyle.American, OptionRight.Call, 225m, new DateTime(2024, 7, 12)); AddOptionContract(_mirrorOption, Resolution.Daily); var t = T(_option, _mirrorOption); var countIndicatorHistory = IndicatorHistory(t, new[] { _symbol, _option, _mirrorOption }, 100, Resolution.Minute); var timeSpanIndicatorHistory = IndicatorHistory(t, new[] { _symbol, _option, _mirrorOption }, TimeSpan.FromDays(10), Resolution.Minute); var timePeriodIndicatorHistory = IndicatorHistory(t, new[] { _symbol, _option, _mirrorOption }, new DateTime(2024, 7, 1), new DateTime(2024, 7, 5), Resolution.Minute); } }
class ThetaAlgorithm(QCAlgorithm): def initialize(self) -> None: self._symbol = self.add_equity("SPY", Resolution.DAILY).symbol self._option = Symbol.create_option("SPY", Market.USA, OptionStyle.AMERICAN, OptionRight.PUT, 225, datetime(2024, 7, 12)) self.add_option_contract(self._option, Resolution.DAILY) self._mirror_option = Symbol.create_option("SPY", Market.USA, OptionStyle.AMERICAN, OptionRight.CALL, 225, datetime(2024, 7, 12)) self.add_option_contract(self._mirror_option, Resolution.DAILY) t = self.t(self.option, self.mirror_option) count_indicator_history = self.indicator_history(t, [self._symbol, self._option, self._mirror_option], 100, Resolution.MINUTE) timedelta_indicator_history = self.indicator_history(t, [self._symbol, self._option, self._mirror_option], timedelta(days=10), Resolution.MINUTE) time_period_indicator_history = self.indicator_history(t, [self._symbol, self._option, self._mirror_option], datetime(2024, 7, 1), datetime(2024, 7, 5), Resolution.MINUTE)
To make the IndicatorHistory
indicator_history
method update the indicator with an alternative price field instead of the close (or mid-price) of each bar, pass a selector
argument.
var indicatorHistory = IndicatorHistory(t, 100, Resolution.Minute, (bar) => ((TradeBar)bar).High);
indicator_history = self.indicator_history(t, 100, Resolution.MINUTE, lambda bar: bar.high) indicator_history_df = indicator_history.data_frame
If you already have a list of Slice objects, you can pass them to the IndicatorHistory
indicator_history
method to avoid the internal history request.
var history = History(new[] { _symbol, _option, _mirrorOption }, 100, Resolution.Minute); var historyIndicatorHistory = IndicatorHistory(t, history);
To access the properties of the indicator history, invoke the property of each IndicatorDataPoint
object.index the DataFrame with the property name.
var impliedvolatility = indicatorHistory.Select(x => ((dynamic)x).ImpliedVolatility).ToList(); var optionsymbol = indicatorHistory.Select(x => ((dynamic)x).OptionSymbol).ToList(); var riskfreerate = indicatorHistory.Select(x => ((dynamic)x).RiskFreeRate).ToList(); var dividendyield = indicatorHistory.Select(x => ((dynamic)x).DividendYield).ToList(); var price = indicatorHistory.Select(x => ((dynamic)x).Price).ToList(); var oppositeprice = indicatorHistory.Select(x => ((dynamic)x).OppositePrice).ToList(); var underlyingprice = indicatorHistory.Select(x => ((dynamic)x).UnderlyingPrice).ToList(); // Alternative way // var impliedvolatility = indicatorHistory.Select(x => x["impliedvolatility"]).ToList(); // var optionsymbol = indicatorHistory.Select(x => x["optionsymbol"]).ToList(); // var riskfreerate = indicatorHistory.Select(x => x["riskfreerate"]).ToList(); // var dividendyield = indicatorHistory.Select(x => x["dividendyield"]).ToList(); // var price = indicatorHistory.Select(x => x["price"]).ToList(); // var oppositeprice = indicatorHistory.Select(x => x["oppositeprice"]).ToList(); // var underlyingprice = indicatorHistory.Select(x => x["underlyingprice"]).ToList();
implied_volatility = indicator_history_df["implied_volatility"] option_symbol = indicator_history_df["option_symbol"] risk_free_rate = indicator_history_df["risk_free_rate"] dividend_yield = indicator_history_df["dividend_yield"] price = indicator_history_df["price"] opposite_price = indicator_history_df["opposite_price"] underlying_price = indicator_history_df["underlying_price"] # Alternative way # implied_volatility = indicator_history_df.implied_volatility # option_symbol = indicator_history_df.option_symbol # risk_free_rate = indicator_history_df.risk_free_rate # dividend_yield = indicator_history_df.dividend_yield # price = indicator_history_df.price # opposite_price = indicator_history_df.opposite_price # underlying_price = indicator_history_df.underlying_price