Key Concepts
Security Identifiers
Encoding Symbols
SecurityIdentifier
objects have several public properties to uniquely identify each asset. The following table describes these properties:
Property | Data Type | Description |
---|---|---|
market Market | str string | The market in which the asset trades |
security_type SecurityType | SecurityType | The asset class |
option_style OptionStyle | OptionStyle | American or European Option style |
option_right OptionRight | OptionRight | Call or put Option type |
strike_price StrikePrice | float decimal | The Option contract strike price |
date Date | datetime DateTime | Earliest listing date for Equities; expiry for Futures and Options; December 30, 1899 for continuous Futures contracts and Indices. |
has_underlying HasUnderlying | bool | A flag to represent if its a derivative asset with another underlying asset |
We encode the preceding properties to create Symbol
objects. We do our best to hide the details of this process from your algorithm, but you'll occasionally see it come through as an encoded hash like AAPL R735QTJ8XC9X
. The first half of the encoded string represents the first ticker AAPL was listed under. The other letters at the end of the string represent the serialized form of the preceding SecurityIdentifier
properties. You may also see an encoded has like AAPL XL7X5I241SO6|AAPL R735QTJ8XC9X
for a derivative contract. In this case, the part before the |
is contract hash and the part after the |
is the underlying hash. This serialization approach lets LEAN assign a short, unique string to octillions of different security objects.
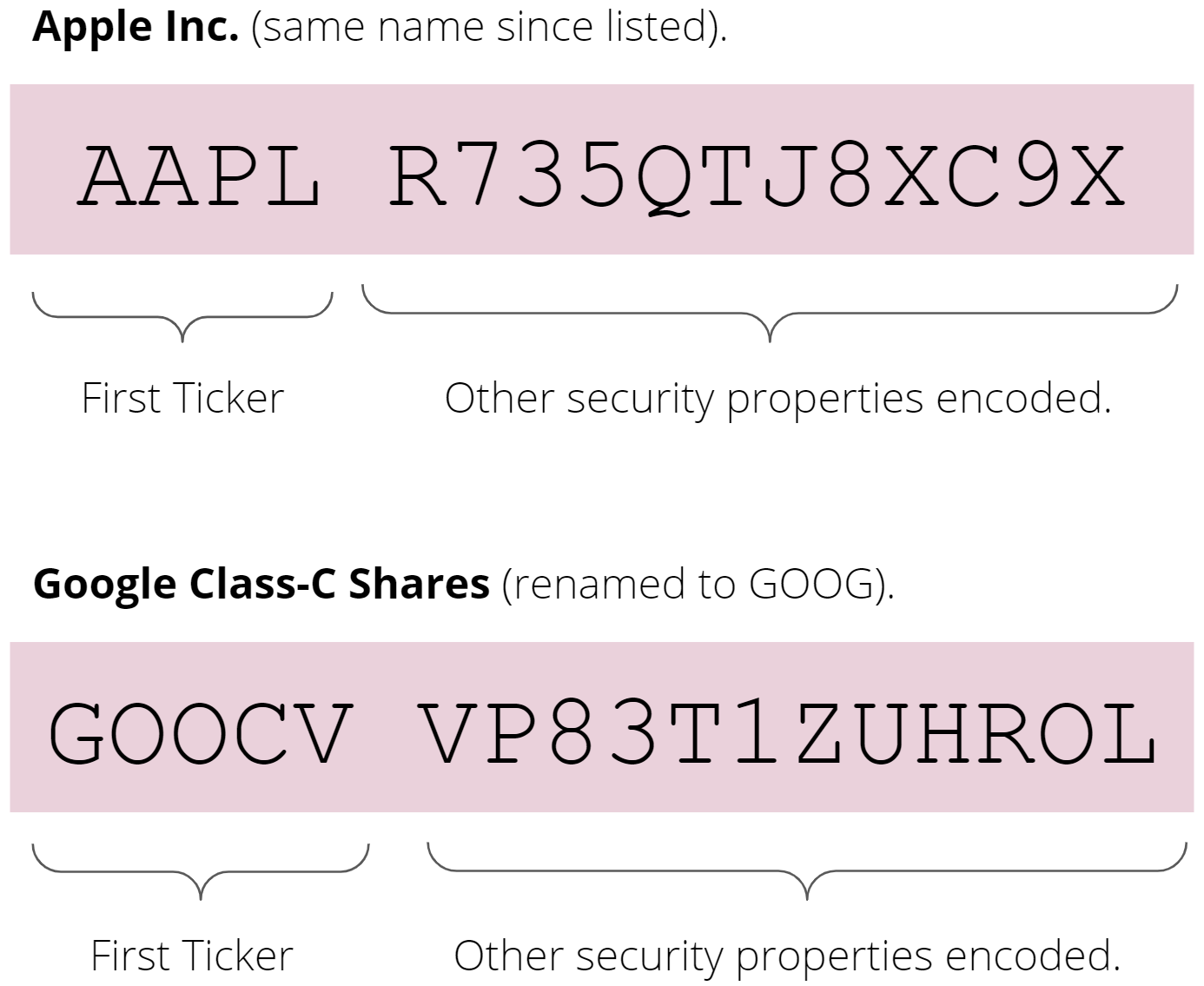
LEAN assumes the ticker you pass to the AddEquityadd_equity method is the current ticker of the Equity asset. To access this ticker, use the Value
value
property of the Symbol
object.
self._symbol = self.add_equity("GOOG").symbol self.debug(self.symbol.id.value) # Prints "GOOCV" self.debug(self.symbol.value) # Prints "GOOG"
var symbol = AddEquity("GOOG").Symbol; Debug(symbol.ID.Value); // Prints "GOOCV" Debug(symbol.Value); // Prints "GOOG"
If you create the security subscription with a universe selection function, the Value
value
property of the Symbol
object is the point-in-time ticker.
Decoding Symbols
When a SecurityIdentifier
is serialized to a string, it looks something like SPY R735QTJ8XC9X
. This two-part string is a base64 encoded set of data. Encoding all of the properties into a short format allows dense communication without requiring a third-party list or look-up. Most of the time, you will not need to work with these encoded strings. However, to deserialize the string into a Symbol
object, call the Symbol
symbol
method.
var symbol = Symbol("GOOCV VP83T1ZUHROL"); symbol.ID.Market; // => "USA" symbol.SecurityType; // => SecurityType.Equity symbol.Value; // => "GOOCV"
symbol = self.symbol("GOOCV VP83T1ZUHROL") symbol.id.market # => "USA" symbol.security_type # => SecurityType.EQUITY symbol.value # => "GOOCV"
The Market
market
property distinguishes between tickers that have the same string value but represent different underlying assets. A prime example of this is the various market makers who have different prices for EURUSD. We store this data separately and as they have different fill prices, we treat the execution venues as different markets.
Tickers
Tickers are a string shortcode representation for an asset. Some examples of popular tickers include "AAPL" for Apple Corporation and "IBM" for International Business Machines Corporation. These tickers often change when the company rebrands or they undergo a merger or reverse merger.
The ticker of an asset is not the same as the Symbol
. Symbol
objects are permanent and track the underlying entity. When a company rebrands or changes its name, the Symbol
object remains constant, giving algorithms a way to reliably track assets over time.
Tickers are also often reused by different brokerages. For example Coinbase, a leading US Crypto Brokerage lists the "BTCUSD" ticker for trading. Its competitor, Bitfinex, also lists "BTCUSD". You can trade both tickers with LEAN. Symbol
objects allow LEAN to identify which market you reference in your algorithms.
To create a Symbol
object for a point-in-time ticker, call the GenerateEquity
generate_equity
method to create the security identifier and then call the Symbol
constructor. For example, Heliogen, Inc. changed their ticker from ATHN to HLGN on December 31, 2021. To convert the ATHN ticker to the Equity Symbol
, type:
ticker = "ATHN" security_id = SecurityIdentifier.generate_equity(ticker, Market.USA, mapping_resolve_date=datetime(2021, 12, 1)) symbol = Symbol(security_id, ticker)
var ticker = "ATHN"; var securityID = SecurityIdentifier.GenerateEquity(ticker, Market.USA, mappingResolveDate:new DateTime(2021, 12, 1)); var symbol = new Symbol(securityID, ticker);
In the preceding code snippet, the mappingResolveDate
mapping_resolve_date
must be a date when the point-in-time ticker was trading.
Symbol Cache
To make using the API easier, we have built the Symbol Cache technology, which accepts strings and tries to guess which Symbol
object you might mean. With the Symbol Cache, many methods can accept a string such as "IBM" instead of a complete Symbol
object. We highly recommend you don't rely on this technology and instead save explicit references to your securities when you need them.
# Example 1: Relying On Symbol Cache: self.add_equity("IBM") # Add by IBM string ticker, save reference to Symbol Cache. self.market_order("IBM", 100) # Determine refering to IBM Equity from Symbol Cache. self.history("AAPL", 14) # Makes a guess referring to AAPL Equity. # Example 2: Correctly Using Symbols: self.ibm = self.add_equity("IBM").symbol # Add IBM Equity string ticker, save Symbol. self.market_order(self.ibm, 100) # Use IBM Symbol in future API calls. self.aapl = Symbol.create("AAPL", SecurityType.EQUITY, Market.USA) self.history(self.aapl, 14)
// Example 1: Relying On Symbol Cache: AddEquity("IBM"); // Add by IBM string ticker, save reference to Symbol Cache. MarketOrder("IBM", 100); // Determine refering to IBM Equity from Symbol Cache. History("AAPL", 14); // Guess referring to AAPL Equity. // Example 2: Correctly Using Symbols: var ibm = AddEquity("IBM").Symbol; // Add IBM Equity string ticker, save Symbol. MarketOrder(ibm, 100); // Use IBM Symbol in future API calls. var aapl = Symbol.Create("AAPL", SecurityType.Equity, Market.USA); History(aapl, 14);
Industry Standard Identifiers
You can convert industry-standard security identifiers like CUSIP, FIGI, ISIN, and SEDOL to Symbol
objects and you can convert Symbol
objects to industry-standard security identifiers.
CUSIP
To convert a Committee on Uniform Securities Identification Procedures (CUSIP) number to a Symbol
or a Symbol
to a CUSIP number, call the CUSIP
cusip
method.
symbol = self.cusip("03783310") # AAPL Symbol cusip = self.cusip(symbol) # AAPL CUSIP (03783310)
var symbol = CUSIP("03783310"); // AAPL Symbol var cusip = CUSIP(symbol); // AAPL CUSIP (03783310)
FIGI
To convert a Financial Instrument Global Identifier (FIGI) to a Symbol
or a Symbol
to a FIGI, call the CompositeFIGI
composite_figi
method.
symbol = self.composite_figi("BBG000B9XRY4") # AAPL Symbol figi = self.composite_figi(symbol) # AAPL FIGI (BBG000B9XRY4)
var symbol = CompositeFIGI("BBG000B9XRY4"); // AAPL Symbol var figi = CompositeFIGI(symbol); // AAPL FIGI (BBG000B9XRY4)
ISIN
To convert an International Securities Identification Number (ISIN) to a Symbol
or a Symbol
to an ISIN, call the ISIN
isin
method.
symbol = self.isin("US0378331005") # AAPL Symbol isin = self.isin(symbol) # AAPL ISIN (US0378331005)
var symbol = ISIN("US0378331005"); // AAPL Symbol var isin = ISIN(symbol); // AAPL ISIN (US0378331005)
SEDOL
To convert a Stock Exchange Daily Official List (SEDOL) number to a Symbol
or a Symbol
to a SEDOL number, call the SEDOL
sedol
method.
symbol = self.sedol("2046251") # AAPL Symbol sedol = self.sedol(symbol) # AAPL SEDOL (2046251)
var symbol = SEDOL("2046251"); // AAPL Symbol var sedol = SEDOL(symbol); // AAPL SEDOL (2046251)